How to Give Your Phidgets a Custom Label
Using labels to easily identify Phidget devices in software
by Kat
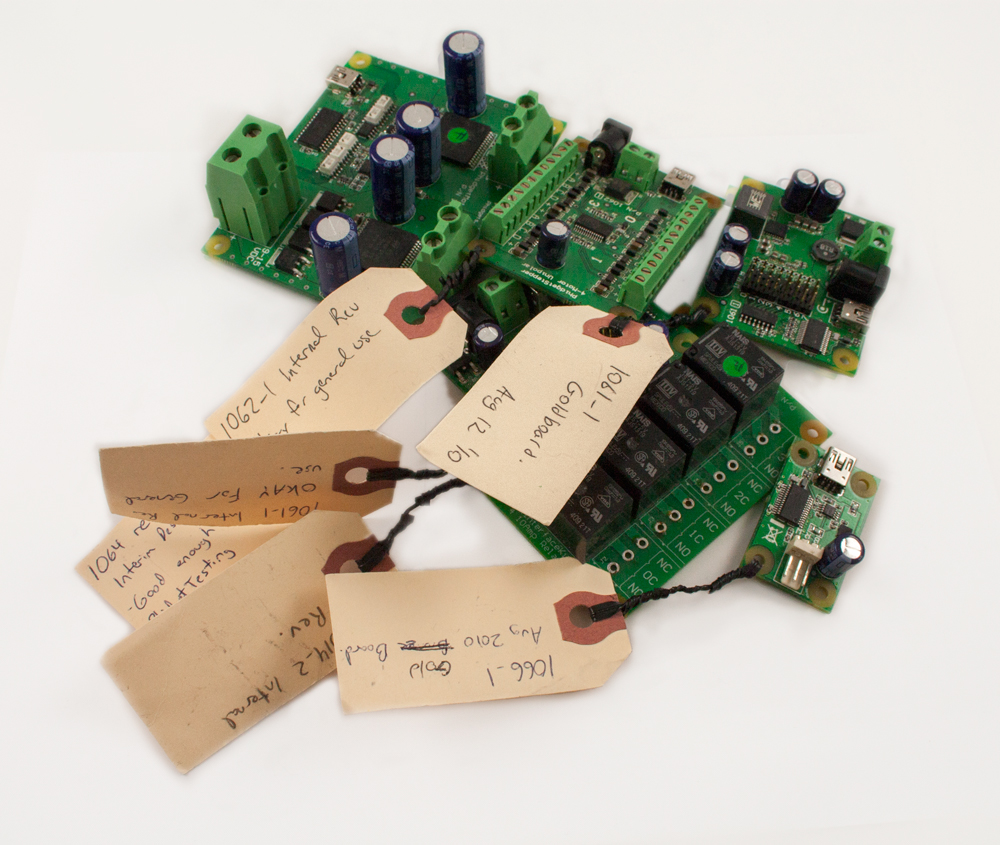
Introduction
At first glance, the device label property looks simple and maybe even dismissible. If you noticed it at all, you may have even wondered why it’s there, but be assured, there are some really neat things that you can do with it. You can hold device-specific flags, differentiate between multiple Phidgets by giving them meaningful names, or give them custom serial numbers. Labels are ideal for users who want to periodically store a small amount of information – like an identifier or flags – on a Phidget USB device, wether that’s across different computers or between uses. Because the label is stored on flash memory, it’s non destructive and will be held by the device even when unplugged.
The device label property is a ten character string that allows you to assign an identifier to a device. It can be used extensively in Linux, Mac, iOS and Android. Most features also work on Windows. We’ll discuss the limitations later in this post, and show examples of how the device label property is used.
Note: Labels have full Unicode support (UTF-8), allowing 20 bytes of storage technically (10 characters).
Limitations
First, the label has a ten character limit, but unless you need more than 1024 unique identifiers, this shouldn’t be a problem. If you try to send more than ten characters, the label will not set, so it’s best to truncate the string before sending it.
Next, the labels are on flash storage, which has a limit of 10,000 re-writes. If you set the device label every time you run a program, when not needing to, you can easily reach the 10,000 re-write limit. Best practice is to set the label only when needed, like in the Mac Phidget Control Panel, a one-off program or after checking that certain conditions are met to be sure a re-write is needed.
The biggest limitations are in the languages that device labels and label functions do not work in, these are:
- You cannot set a label using any language in Windows. However, you can get the label of a Phidget on Windows, and open a Phidget by its label
- Android Java has support for only a subset of the open() functions that use labels, see the Android Java language page for details
- Labels are not built into the Max 6 libraries
- LabVIEW, Python, LiveCode and all .COM languages on Windows (including AutoIt, Adobe Director, Delphi and all of the Visual Basic flavours) cannot open by label
UPDATE: Labels can actually be set on Windows for the newest Phidgets, which are based on the LPC1343 microprocessor. These include: 1024, 1032, 1041, 1042, 1043, 1044 and 1067.
While almost all of the Phidgets we tested did support labels (even older versions and discontinued models), there is a possibility that some older versions of Phidgets won’t support labels. The only Phidget we tested that didn’t support labels was the 1045 Temperature Sensor IR.
Finally, when using labels to identify devices, it’s important to remember that labels are easily changed in the Mac Phidgets Control Panel. On top of that, anyone who knows how to program Phidgets can overwrite the label with a program of their own.
Keep these limitations in mind when using device labels in your programs.
Implementation
Note: The code examples in this article use Phidget21 code and may not work with newer versions of the Phidget library.
The label property can be set from a program on Linux, Mac, iOS and Android. In most languages,
the function setDeviceLabel(string label)
is called on a Phidget
object.
For example, in C, this is what it looks like to set the label of an interface kit:
CPhidgetInterfaceKitHandle ifKit = 0;
CPhidgetInterfaceKit_create(&ifKit);
CPhidget_open((CPhidgetHandle)ifKit, -1);
if ( result = CPhidget_waitForAttachment((CPhidgetHandle)ifKit, 10000) ) return 1;
// set the device label here
CPhidget_setDeviceLabel((CPhidgetHandle)ifKit, "myPhidget1");
CPhidget_close((CPhidgetHandle)ifKit);
CPhidget_delete((CPhidgetHandle)ifKit);
You can get the label in any language, and on any operating system. In most cases, call
the function getDeviceLabel()
on the Phidget object to get the label
as a string. In C, it looks something like this:
char *label;
CPhidgetInterfaceKitHandle ifKit = 0;
CPhidgetInterfaceKit_create(&ifKit);
CPhidget_open((CPhidgetHandle)ifKit, -1);
if ( result = CPhidget_waitForAttachment((CPhidgetHandle)ifKit, 10000) ) return 1;
// save the device label here
CPhidget_getDeviceLabel((CPhidgetHandle)ifKit, &label);
CPhidget_close((CPhidgetHandle)ifKit);
CPhidget_delete((CPhidgetHandle)ifKit);
Similarly, in Python the call could look like this:
try:
interfaceKit = InterfaceKit()
interfaceKit.openPhidget()
interfaceKit.waitForAttach(10000)
# save the device label here
myLabel = interfaceKit.getDeviceLabel()
except PhidgetException as e:
print("Phidget Exception %i: %s" % (e.code, e.details))
print("Exiting....")
exit(1)
The last label function is open. It’s not available in any of the Windows .COM languages
and limited on Android Java. The openLabel function allows you to use a device’s label to open the Phidget.
In C/C++, instead of using a call like CPhidget_Open(CPhidgetHandle ifkit, int serialNumber)
,
you can specify the Phidget you want to open with the label by calling CPhidget_openLabel(CPhidgetHandle ifkit, string label)
.
Likewise, in Java, call openLabel(string)
on the Phidget object. There
are other variations of the openLabel function, like being able to open a Phidget by the device label
remotely using a Server ID or IP address and port. Check the API documentation for the language you want to work with.
For people using OSX, it’s even easier. You can use the Phidget Control Panel to check and change device labels. When you have the Control Panel open, click on the “Labels” tab. You can change labels for Phidgets connected to your computer by selecting the “Local Phidgets” radio button.
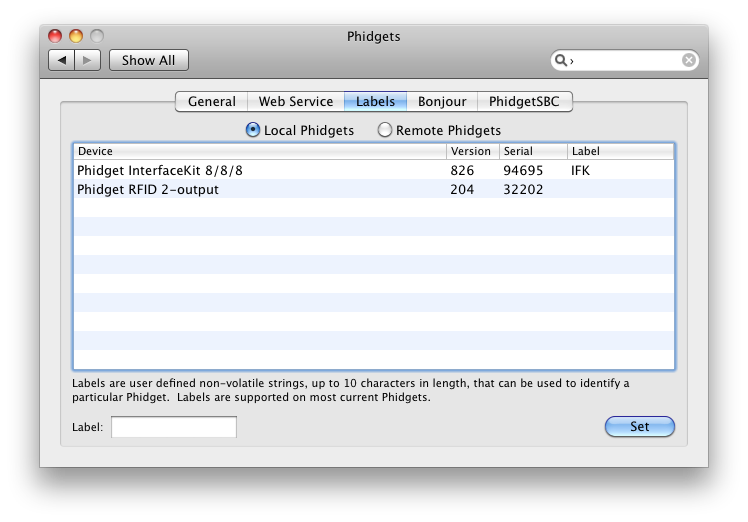
For Phidgets connected on your local network, you can check and change their labels by going to the “Remote Phidgets” radio button.
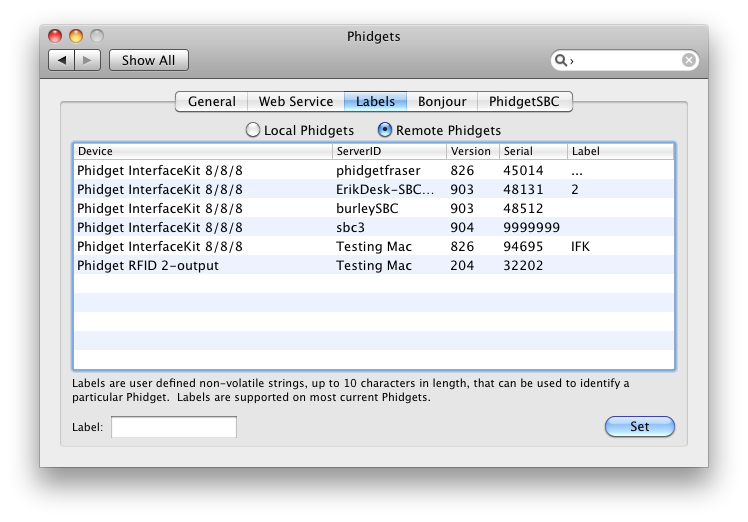
Unfortunately, this feature is not available on the Windows Control Panel, so all label functions must be done through code.
Benefits and Uses
Now that we’ve run through the basics, covering the limitations of labels as well as how to implement them, let’s look at these more closely to get an idea of how to use them.
Custom serial numbers are great if you’re using Phidgets in a larger implementation. This works especially well on Windows platforms, where the setDeviceLabel function doesn’t work. On other operating systems, you run the risk of people re-writing the label, if they know how. There are several ways to create custom device labels. You can use the device serial number and encryption to create the label, then check that the Phidget device belongs to your product. Similarly, you could create labels from a key that comes with your product.
Similarly, if you have several different programs using Phidgets on your computer, you might want to give different labels to the devices used by different applications. While serial numbers would perform the same function, a descriptive name can be more readable than an ambiguous number.
You can maximize the portability of your programs by using labels. Say you’re working on some code with others.
You all have the same Phidgets set up at your own computers, but some people use other Phidget devices
(not part of the project). Instead of each coder having to modify the code each time they go to work
on it, have everyone set the same labels for their devices related to the project. So, if the project
uses a motor controller and an interfaceKit with a few different distance sensors, have everyone label
the motor controller as “proj-moto” and the interface kit as “proj-ifkit” then use the language appropriate
calls to openLabel("proj-moto")
and openLabel("proj-ifkit")
to create the respective Phidget objects.
Perhaps, you want to save device specific information when you might be unplugging the Phidget device. While this isn’t the best use of labels, it can work in a pinch. You can use the label property to record the last state of the digital inputs or outputs. Use this with caution because you can only re-write the label 10,000 times.
For instance, you could use each character as a flag to set some LEDs to the last state they were in. Here’s a snippet of C code showing you how that might look:
//set the outputs according to flags
CPhidget_getDeviceLabel((CPhidgetHandle)ifKit, &label);
strcpy(flags, label);
for (i = numOuts - 1; i >= 0; i--)
{
if ( flags[i] == 'y' )
{
CPhidgetInterfaceKit_setOutputState(ifKit, i, PTRUE);
}
else
{
CPhidgetInterfaceKit_setOutputState(ifKit, i, PFALSE);
}
}
//do some stuff
usleep(1000000);
for (i = 0; i < numOuts; i++)
{
if (i%2 == 1) CPhidgetInterfaceKit_setOutputState(ifKit, i, PTRUE);
}
usleep(1000000);
//get the output states
for (i = 0; i < numOuts; i++)
{
CPhidgetInterfaceKit_getOutputState(ifKit, i, &result);
if (result)
{
flags[i] = 'y';
}
else
{
flags[i] = 'n';
}
}
CPhidget_getDeviceLabel((CPhidgetHandle)ifKit, &label);
// if the flags changed, set the label
if( (result = strcmp(flags,label)) != 0)
{
CPhidget_setDeviceLabel((CPhidgetHandle)ifKit, flags);
}
Conclusion
Although labels are simple, there are some powerful ways to make use of them. This ten character device property can be used for purposes from custom serial numbers to device-specific flags. There are no doubt other creative uses for device labels not explored here, and we’d love to hear them. Please leave a comment if you’ve used labels and let us know what you did.