In this step, you will learn how to use your Moisture Phidget.
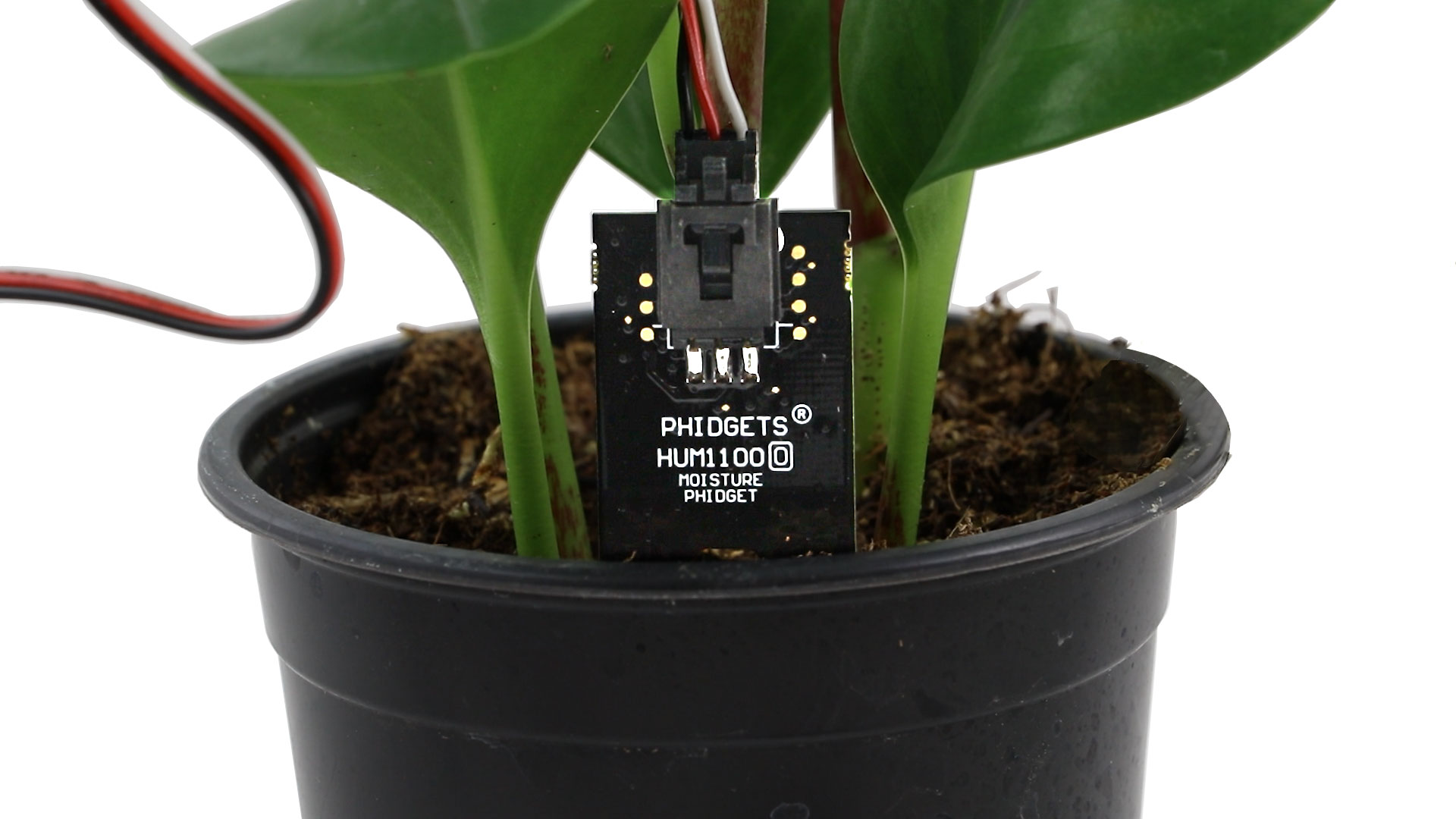
You will need to create a new project called PhidgetsPlant and import the Phidgets Library. If you’ve forgotten how to do this, revisit the Configure section from the Getting Started Kit.
Write Code (Java)
Not your programming language? Set your preferences so we can display relevant code examples.
package phidgetsplant;
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsPlant {
public static void main(String[] args) throws Exception {
//Create
VoltageRatioInput soil = new VoltageRatioInput();
//Open
soil.open(1000);
//Use your Phidgets
while(true){
System.out.println("Soil Moisture Level: " + soil.getVoltageRatio());
Thread.sleep(100);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsPlant {
public static void main(String[] args) throws Exception {
//Create
VoltageRatioInput soil = new VoltageRatioInput();
//Open
soil.open(1000);
//Use your Phidgets
while(true){
System.out.println("Soil Moisture Level: " + soil.getVoltageRatio());
Thread.sleep(100);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
VoltageRatioInput soil;
void setup(){
try{
//Create
soil = new VoltageRatioInput();
//Open
soil.open(1000);
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Soil Moisture Level: " + soil.getVoltageRatio());
delay(250);
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
Write Code (Python)
Not your programming language? Set your preferences so we can display relevant code examples.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.VoltageRatioInput import *
#Required for sleep statement
import time
#Create
soil = VoltageRatioInput()
#Open
soil.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Soil Moisture Level: " + str(soil.getVoltageRatio()))
time.sleep(0.1)
Write Code (C#)
Not your programming language? Set your preferences so we can display relevant code examples.
//Add Phidgets Library
using Phidget22;
namespace PhidgetsPlant
{
class Program
{
static void Main(string[] args)
{
//Create
VoltageRatioInput soil = new VoltageRatioInput();
//Open
soil.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("Soil Moisture Level: " + soil.VoltageRatio);
System.Threading.Thread.Sleep(100);
}
}
}
}
Write Code (Swift)
Not your programming language? Set your preferences so we can display relevant code examples.
The code below assumes you've created a single label and linked it to an IBAction named soilLabel
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var soilLabel: NSTextField!
//Create
let soil = VoltageRatioInput()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = soil.voltageRatioChange.addHandler(soil_change)
//Open
try soil.open()
}catch{
print(error)
}
}
func soil_change(sender:VoltageRatioInput, voltageRatio: Double){
DispatchQueue.main.async {
//Use your Phidget
self.soilLabel.stringValue = String(voltageRatio)
}
}
}
Run Your Program
Your program will print a soil moisture value between 0 and 1.
Collect Values
Using your program, determine the following values:
Moisture Sensor Location | Voltage Ratio |
---|---|
In Air | ? |
In Dry Soil | ? |
In Water | ? |
Make sure to write these values down. You will need them later when designing your system.
Troubleshoot
- Make sure everything is plugged in properly and your VINT Hub is connected to your computer.
- Stop running other programs that are using your Phidgets before running a program.
If you are still having issues visit Advanced Troubleshooting