Now that you understand how each component of your Plant Kit works, you can begin designing your system.
You can continue working from your PhidgetsPlant project in this step. Replace your previous code with the following code.
Write Code (Java)
Not your programming language? Set your preferences so we can display relevant code examples.
package phidgetsplant;
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsPlant {
public static void main(String[] args) throws Exception {
//Create
VoltageRatioInput soil = new VoltageRatioInput();
DigitalOutput pump = new DigitalOutput();
LightSensor light = new LightSensor();
//Address
pump.setHubPort(1);
pump.setIsHubPortDevice(true);
//Open
soil.open(1000);
pump.open(1000);
light.open(1000);
//Use your Phidgets
while(true){
System.out.println("Illuminance: " + light.getIlluminance() + " lx");
System.out.println("Soil Moisture Level: " + soil.getVoltageRatio() + "\n");
Thread.sleep(500);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsPlant {
public static void main(String[] args) throws Exception {
//Create
VoltageRatioInput soil = new VoltageRatioInput();
DigitalOutput pump = new DigitalOutput();
LightSensor light = new LightSensor();
//Address
pump.setHubPort(1);
pump.setIsHubPortDevice(true);
//Open
soil.open(1000);
pump.open(1000);
light.open(1000);
//Use your Phidgets
while(true){
System.out.println("Illuminance: " + light.getIlluminance() + " lx");
System.out.println("Soil Moisture Level: " + soil.getVoltageRatio() + "\n");
Thread.sleep(500);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
VoltageRatioInput soil;
DigitalOutput pump;
LightSensor light;
void setup(){
try{
//Create
soil = new VoltageRatioInput();
pump = new DigitalOutput();
light = new LightSensor();
//Address
pump.setHubPort(1);
pump.setIsHubPortDevice(true);
//Open
soil.open(1000);
light.open(1000);
pump.open(1000);
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Illuminance: " + light.getIlluminance() + " lx");
println("Soil Moisture Level: " + soil.getVoltageRatio() + "\n");
delay(500);
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
Write Code (Python)
Not your programming language? Set your preferences so we can display relevant code examples.
#Add Phidget Library
from Phidget22.Phidget import *
from Phidget22.Devices.DigitalOutput import *
from Phidget22.Devices.VoltageRatioInput import *
from Phidget22.Devices.LightSensor import *
#Required for sleep statement
import time
#Create
soil = VoltageRatioInput()
pump = DigitalOutput()
light = LightSensor()
#Address
pump.setHubPort(1)
pump.setIsHubPortDevice(True)
#Open
pump.openWaitForAttachment(1000)
soil.openWaitForAttachment(1000)
light.openWaitForAttachment(1000)
#Use your Phidgets
while(True):
print("Illuminance: " + str(light.getIlluminance()) + " lx")
print("Soil Moisture Level: " + str(soil.getVoltageRatio()) + "\n")
time.sleep(0.5)
Write Code (C#)
Not your programming language? Set your preferences so we can display relevant code examples.
//Add Phidgets Library
using Phidget22;
namespace PhidgetsPlant
{
class Program
{
static void Main(string[] args)
{
//Create
VoltageRatioInput soil = new VoltageRatioInput();
DigitalOutput pump = new DigitalOutput();
LightSensor light = new LightSensor();
//Address
pump.HubPort = 1;
pump.IsHubPortDevice = true;
//Open
soil.Open(1000);
pump.Open(1000);
light.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("Illuminance: " + light.Illuminance + " lx");
System.Console.WriteLine("Soil Moisture Level: " + soil.VoltageRatio +"\n");
System.Threading.Thread.Sleep(250);
}
}
}
}
Write Code (Swift)
Not your programming language? Set your preferences so we can display relevant code examples.
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var lightLabel: NSTextField!
@IBOutlet weak var soilLabel: NSTextField!
@IBOutlet weak var pumpButton: NSButton!
//Create
let light = LightSensor()
let soil = VoltageRatioInput()
let pump = DigitalOutput()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to events
let _ = light.illuminanceChange.addHandler(light_change)
let _ = soil.voltageRatioChange.addHandler(soil_change)
//Address
try pump.setHubPort(1)
try pump.setIsHubPortDevice(true)
//Open
try light.open()
try soil.open()
try pump.open()
}catch{
print(error)
}
}
func light_change(sender:LightSensor, illuminance: Double){
DispatchQueue.main.async {
//Use your Phidget
self.lightLabel.stringValue = "Illuminance: " + String(illuminance) + " lx"
}
}
func soil_change(sender:VoltageRatioInput, voltageRatio: Double){
DispatchQueue.main.async {
//Use your Phidget
self.soilLabel.stringValue = "Soil Moisture Level: " + String(voltageRatio)
}
}
@IBAction func runPump(_ sender: Any) {
do{
let currentState = try pump.getState()
let buttonStr = currentState ? "Start Pump" : "Stop Pump"
pumpButton.title = buttonStr
try pump.setState(!currentState)
}catch{
print(error)
}
}
}
Run Your Program
You will see the moisture level and the light level printed to your screen.
Modify Your System
Using the code above and the values you recorded in previous steps, complete the following:
Light Modifications
- If the light level is lower than required for your plant, output "Light Status: Low" to the user.
- If the light level is higher than required for your plant, output "Light Status: High" to the user.
- If the light level is adequate, output "Light Status: Good" to the user.
Moisture Modifications
- If the plant needs watering (i.e. the soil is dry), output "Plant Status: Needs Watering" to the user.
- If the moisture level is adequate, output "Plant Status: Good" to the user.
Test Your Modifications
Test your modifications to make sure it behaves properly.
- Cover the Light Phidget with your hand to simulate darkness.
- Use your phone flashlight to simulate high light levels.
- Take the Moisture Phidget out of the plant to simulate a dry plant.
- Dip the Moisture Phidget in water to simulate a watered plant.
Add the Water Pump
Now that have verified your system is working, you can add the water pump to your system. Do not water the plant yet. Keep the pump attached to the test reservoir.
When the plant status "needs watering" try activating your pump. Reference the data you collected in a previous step to determine how long to keep the pump on for.
Complete Your System
When you are confident that your logic is working properly, try adding the tube to your plant and running your program!
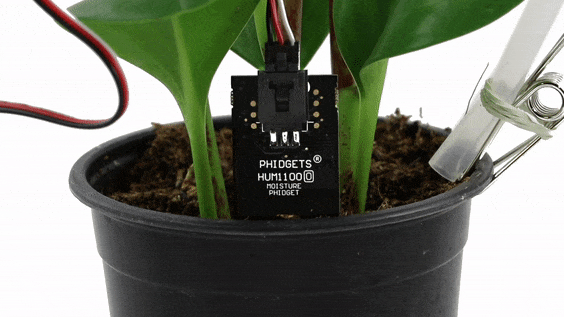
Troubleshoot
- Make sure everything is plugged in properly and your VINT Hub is connected to your computer.
- Stop running other programs that are using your Phidgets before running a program.
If you are still having issues visit Advanced Troubleshooting