Button & LED Events
In this lesson, you’ll learn how to write a program that gets input from a button using events and outputs to an LED!
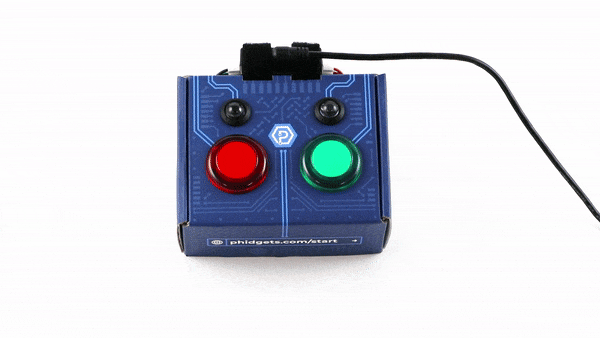
Write Code (Java)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
package gettingstarted;
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
//Turn on/off LEDs with Global Variables
static boolean turnRedLEDOn = false;
static boolean turnGreenLEDOn = false;
//Handle Exceptions
public static void main(String[] args) throws Exception {
//Create
DigitalInput redButton = new DigitalInput();
DigitalInput greenButton = new DigitalInput();
DigitalOutput redLED = new DigitalOutput();
DigitalOutput greenLED = new DigitalOutput();
//Address
redButton.setHubPort(0);
redButton.setIsHubPortDevice(true);
greenButton.setHubPort(5);
greenButton.setIsHubPortDevice(true);
redLED.setHubPort(1);
redLED.setIsHubPortDevice(true);
greenLED.setHubPort(4);
greenLED.setIsHubPortDevice(true);
//Event | Event code runs when data input from the sensor changes. The following event is a state change event. The code will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
redButton.addStateChangeListener(new DigitalInputStateChangeListener() {
public void onStateChange(DigitalInputStateChangeEvent e) {
//Record button state to turn on/off the red LED
turnRedLEDOn = e.getState();
}
});
//Event | Event code runs when data input from the sensor changes. The following event is a state change event. It will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
greenButton.addStateChangeListener(new DigitalInputStateChangeListener() {
public void onStateChange(DigitalInputStateChangeEvent e) {
//Record button state to turn on/off the green LED
turnGreenLEDOn = e.getState();
}
});
//Open
redLED.open(1000);
greenLED.open(1000);
redButton.open(1000);
greenButton.open(1000);
//Use your Phidgets | In the button events you recorded the Button State. Here we will use that data to turn on/off the LEDs
while(true) {
//turn red LED on based on red button input
redLED.setState(turnRedLEDOn);
//turn green LED on based on green button input
greenLED.setState(turnGreenLEDOn);
//sleep for 150 milliseconds
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
//Turn on/off LEDs with Global Variables
static boolean turnRedLEDOn = false;
static boolean turnGreenLEDOn = false;
//Handle Exceptions
public static void main(String[] args) throws Exception {
//Create
DigitalInput redButton = new DigitalInput();
DigitalInput greenButton = new DigitalInput();
DigitalOutput redLED = new DigitalOutput();
DigitalOutput greenLED = new DigitalOutput();
//Address
redButton.setHubPort(0);
redButton.setIsHubPortDevice(true);
greenButton.setHubPort(5);
greenButton.setIsHubPortDevice(true);
redLED.setHubPort(1);
redLED.setIsHubPortDevice(true);
greenLED.setHubPort(4);
greenLED.setIsHubPortDevice(true);
//Event | Event code runs when data input from the sensor changes. The following event is a state change event. The code will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
redButton.addStateChangeListener(new DigitalInputStateChangeListener() {
public void onStateChange(DigitalInputStateChangeEvent e) {
//Record button state to turn on/off the red LED
turnRedLEDOn = e.getState();
}
});
//Event | Event code runs when data input from the sensor changes. The following event is a state change event. It will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
greenButton.addStateChangeListener(new DigitalInputStateChangeListener() {
public void onStateChange(DigitalInputStateChangeEvent e) {
//Record button state to turn on/off the green LED
turnGreenLEDOn = e.getState();
}
});
//Open
redLED.open(1000);
greenLED.open(1000);
redButton.open(1000);
greenButton.open(1000);
//Use your Phidgets | In the button events you recorded the Button State. Here we will use that data to turn on/off the LEDs
while(true) {
//turn red LED on based on red button input
redLED.setState(turnRedLEDOn);
//turn green LED on based on green button input
greenLED.setState(turnGreenLEDOn);
//sleep for 150 milliseconds
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
DigitalInput redButton;
DigitalInput greenButton;
DigitalOutput redLED;
DigitalOutput greenLED;
boolean turnRedLEDOn = false;
boolean turnGreenLEDOn = false;
void setup(){
try{
//Create
redButton = new DigitalInput();
greenButton = new DigitalInput();
redLED = new DigitalOutput();
greenLED = new DigitalOutput();
//Address
redButton.setHubPort(0);
redButton.setIsHubPortDevice(true);
greenButton.setHubPort(5);
greenButton.setIsHubPortDevice(true);
redLED.setHubPort(1);
redLED.setIsHubPortDevice(true);
greenLED.setHubPort(4);
greenLED.setIsHubPortDevice(true);
//Event | Event code runs when data input from the sensor changes. The following event is a state change event. The code will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
redButton.addStateChangeListener(new DigitalInputStateChangeListener() {
public void onStateChange(DigitalInputStateChangeEvent e) {
//Record button state to turn on/off the red LED
turnRedLEDOn = e.getState();
}
});
//Event | Event code runs when data input from the sensor changes. The following event is a state change event. The code will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
greenButton.addStateChangeListener(new DigitalInputStateChangeListener() {
public void onStateChange(DigitalInputStateChangeEvent e) {
//Record button state to turn on/off the green LED
turnGreenLEDOn = e.getState();
}
});
//Open
redLED.open(1000);
greenLED.open(1000);
redButton.open(1000);
greenButton.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets | In the button events you recorded the Button State. Here we will use that data to turn on/off the LEDs
//turn red LED on based on red button input
redLED.setState(turnRedLEDOn);
//turn green LED on based on green button input
greenLED.setState(turnGreenLEDOn);
delay(150);
}catch(Exception e){
e.printStackTrace();
}
}
Write Code (Python)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.DigitalInput import *
from Phidget22.Devices.DigitalOutput import *
import time
#Event | Event code runs when data input from the sensor changes. The following event is a state change event. The code will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
def redButtonStateChange(self, state):
global turnRedLEDOn
#Record button state to turn on/off the red LED
turnRedLEDOn = state
#Event | Event code runs when data input from the sensor changes. The following event is a state change event. The code will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
def greenButtonStateChange(self, state):
global turnGreenLEDOn
#Record button state to turn on/off the green LED
turnGreenLEDOn = state
#Create
redButton = DigitalInput()
greenButton = DigitalInput()
redLED = DigitalOutput()
greenLED = DigitalOutput()
#Address
redButton.setHubPort(0)
redButton.setIsHubPortDevice(True)
greenButton.setHubPort(5)
greenButton.setIsHubPortDevice(True)
redLED.setHubPort(1)
redLED.setIsHubPortDevice(True)
greenLED.setHubPort(4)
greenLED.setIsHubPortDevice(True)
#Subscribe to events | Subscribing to an Event tells your program to start listening to a Phidget. Here, the program will begin to listen to the state of the button (Digital Input Object).
redButton.setOnStateChangeHandler(redButtonStateChange)
greenButton.setOnStateChangeHandler(greenButtonStateChange)
#Open
redButton.openWaitForAttachment(1000)
greenButton.openWaitForAttachment(1000)
redLED.openWaitForAttachment(1000)
greenLED.openWaitForAttachment(1000)
#Turn on/off LEDs with Global Variables
turnRedLEDOn = False
turnGreenLEDOn = False
#Use your Phidgets | In the button events you recorded the Button State. Here we will use that data to turn on/off the LEDs
while (True):
#turn red LED on based on red button input
redLED.setState(turnRedLEDOn)
#turn green LED on based on red button input
greenLED.setState(turnGreenLEDOn)
time.sleep(0.15)
Write Code (C#)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
using Phidget22.Events;
namespace GettingStarted
{
class Program
{
// Turn on/off LEDs with Global Variables
static bool turnRedLEDOn = false;
static bool turnGreenLEDOn = false;
//Event | Event code runs when data input from the sensor changes. The following event is a state change event. The code will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
private static void redButton_StateChange(object sender, DigitalInputStateChangeEventArgs e)
{
//Record button state to turn on/off the red LED
turnRedLEDOn = e.State;
}
//Event | Event code runs when data input from the sensor changes. The following event is a state change event. The code will listen to the button (Digital Input Object) and only run the contain code when the button is pressed or released (state changes).
private static void greenButton_StateChange(object sender, DigitalInputStateChangeEventArgs e)
{
//Record button state to turn on/off the green LED
turnGreenLEDOn = e.State;
}
static void Main(string[] args)
{
//Create
DigitalInput redButton = new DigitalInput();
DigitalInput greenButton = new DigitalInput();
DigitalOutput redLED = new DigitalOutput();
DigitalOutput greenLED = new DigitalOutput();
//Address
redButton.HubPort = 0;
redButton.IsHubPortDevice = true;
greenButton.HubPort = 5;
greenButton.IsHubPortDevice = true;
redLED.HubPort = 1;
redLED.IsHubPortDevice = true;
greenLED.HubPort = 4;
greenLED.IsHubPortDevice = true;
//Subscribe to events | Subscribing to an Event tells your program to start listening to a Phidget. Here, the program will begin to listen to the state of the button (Digital Input Object).
redButton.StateChange += redButton_StateChange;
greenButton.StateChange += greenButton_StateChange;
//Open
redLED.Open(1000);
greenLED.Open(1000);
redButton.Open(1000);
greenButton.Open(1000);
//Use your Phidgets | In the button events you recorded the Button State. Here we will use that data to turn on/off the LEDs
while (true)
{
// turn red LED on based on red button input
redLED.State = turnRedLEDOn;
// turn green LED on based on red button input
greenLED.State = turnGreenLEDOn;
System.Threading.Thread.Sleep(150);
}
}
}
}
Write Code (Swift)
Oops, how did you end up here? There is no Button Events lesson for Swift. Go back to the main tutorial page.
Run Your Program
When you press a button, the corresponding LED will blink.
Practice
- Compare the code sample to the code sample in Lesson 3. Note the differences in the code and the program’s output.
- Modify your code so the green button controls the red LED and the red button controls the green LED.
- Modify your code to count the total number of button presses.
- Challenge: Recreate the tug of war game from Simple Phidgets . Each player is assigned a button (red or green). Players will race to see who can press their button ten times first. You should turn both LEDs on and print the winner to the user.
Troubleshoot
I am getting a "Timed Out" exception.
- Make sure the USB cable from your VINT Hub to your computer is attached properly.
- Make the Phidget cable (the black, red and white one) is connected to your VINT Hub and to your Humidity Phidget properly).
- Make sure no other program is running that uses Phidgets. If a Phidget is already in use in another program, it will be busy and won't respond to this one.
Still having issues?
Visit the Advanced Troubleshooting Page or contact us (education@phidgets.com).