Use Buttons and LEDs
In this lesson, you’ll learn how to write a program that uses both LEDs and buttons!
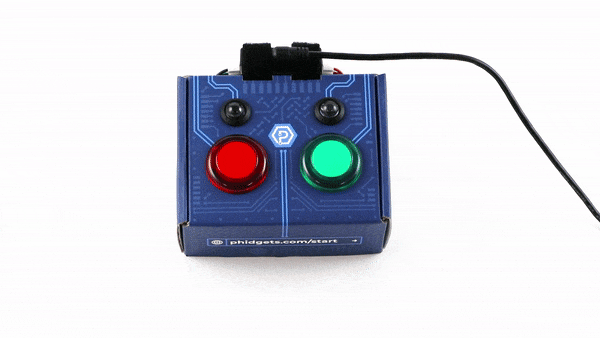
Write Code (Java)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
package gettingstarted;
//Add Phidgets Library | You added a file called phidget22 when configuring your project. Import gives you access to the Phidgets library code inside that file.
import com.phidget22.*;
public class GettingStarted {
//Handle Exceptions | Exceptions will happen in your code from time to time. These are caused by unexpected things happening. Make sure you’ve added "throws Exception" to your main method.
public static void main(String[] args) throws Exception{
//Create | Create objects for your buttons and LEDs.
DigitalInput redButton = new DigitalInput();
DigitalOutput redLED = new DigitalOutput();
DigitalInput greenButton = new DigitalInput();
DigitalOutput greenLED = new DigitalOutput();
//Address | Address your four objects which lets your program know where to find them.
redButton.setHubPort(0);
redButton.setIsHubPortDevice(true);
redLED.setHubPort(1);
redLED.setIsHubPortDevice(true);
greenButton.setHubPort(5);
greenButton.setIsHubPortDevice(true);
greenLED.setHubPort(4);
greenLED.setIsHubPortDevice(true);
//Open | Connect your program to your physical devices.
redButton.open(1000);
redLED.open(1000);
greenButton.open(1000);
greenLED.open(1000);
//Use your Phidgets | This code will turn on the LED when the matching button is pressed and turns off the LED when the matching button is released. The sleep function slows down the loop so the button state is only checked every 150ms.
while(true){
if( redButton.getState()){
redLED.setState(true);
} else {
redLED.setState(false);
}
if(greenButton.getState()){
greenLED.setState(true);
} else {
greenLED.setState(false);
}
Thread.sleep(150);
}
}
}
//Add Phidgets Library | You added a file called phidget22 when configuring your project. Import gives you access to the Phidgets library code inside that file.
import com.phidget22.*;
public class GettingStarted {
//Handle Exceptions | Exceptions will happen in your code from time to time. These are caused by unexpected things happening. Make sure you’ve added "throws Exception" to your main method.
public static void main(String[] args) throws Exception{
//Create | Create objects for your buttons and LEDs.
DigitalInput redButton = new DigitalInput();
DigitalOutput redLED = new DigitalOutput();
DigitalInput greenButton = new DigitalInput();
DigitalOutput greenLED = new DigitalOutput();
//Address | Address your four objects which lets your program know where to find them.
redButton.setHubPort(0);
redButton.setIsHubPortDevice(true);
redLED.setHubPort(1);
redLED.setIsHubPortDevice(true);
greenButton.setHubPort(5);
greenButton.setIsHubPortDevice(true);
greenLED.setHubPort(4);
greenLED.setIsHubPortDevice(true);
//Open | Connect your program to your physical devices.
redButton.open(1000);
redLED.open(1000);
greenButton.open(1000);
greenLED.open(1000);
//Use your Phidgets | This code will turn on the LED when the matching button is pressed and turns off the LED when the matching button is released. The sleep function slows down the loop so the button state is only checked every 150ms.
while(true){
if( redButton.getState()){
redLED.setState(true);
} else {
redLED.setState(false);
}
if(greenButton.getState()){
greenLED.setState(true);
} else {
greenLED.setState(false);
}
Thread.sleep(150);
}
}
}
//Add Phidgets Library | You added a file called phidget22 when configuring your project. Import gives you access to the Phidgets library code inside that file.
import com.phidget22.*;
//Define
DigitalInput redButton;
DigitalInput greenButton;
DigitalOutput redLED;
DigitalOutput greenLED;
void setup(){
try{
//Create | Here you've created a DigitalOutput object for your LED. An object represents how you interact with your device. DigitalOutput is a class from the Phidgets library that's used to provide a voltage to things like LEDs.
redButton = new DigitalInput();
greenButton = new DigitalInput();
redLED = new DigitalOutput();
greenLED = new DigitalOutput();
//Address | This tells your program where to find the device you want to work with. Your LED is connected to port 1 and your code reflects that. IsHubPortDevice must be set if you are not using a Smart Phidget (more on this later).
redButton.setHubPort(0);
redButton.setIsHubPortDevice(true);
redLED.setHubPort(1);
redLED.setIsHubPortDevice(true);
greenButton.setHubPort(5);
greenButton.setIsHubPortDevice(true);
greenLED.setHubPort(4);
greenLED.setIsHubPortDevice(true);
//Open | Open establishes a connection between your object and your physical Phidget. You provide a timeout value of 1000 to give the program 1000 milliseconds (1 second) to locate your Phidget. If your Phidget can't be found, an exception will be thrown.
redButton.open(1000);
redLED.open(1000);
greenButton.open(1000);
greenLED.open(1000);
}catch(Exception e){
//Handle Exceptions | Exceptions will happen in your code from time to time. These are caused by unexpected things happening. Make sure you’ve surrounded your code with a try-catch.
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets | Here is where you can have some fun and use your Phidgets! You can turn your LED on/off by setting the state to true/false. The sleep command keeps your LED on by letting 1000 milliseconds pass before turning the LED off.
if(redButton.getState()){
redLED.setState(true);
}
else{
redLED.setState(false);
}
if(greenButton.getState()){
greenLED.setState(true);
} else {
greenLED.setState(false);
}
delay(150);
}catch(Exception e){
//Handle Exceptions | Exceptions will happen in your code from time to time. These are caused by unexpected things happening. Make sure you’ve surrounded your code with a try-catch.
e.printStackTrace();
}
}
Write Code (Python)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library | You used Python's package manager to install the Phidget libraries on your computer. The import statements below give your program access to that code.
from Phidget22.Phidget import *
from Phidget22.Devices.DigitalInput import *
from Phidget22.Devices.DigitalOutput import *
#Required for sleep statement
import time
#Create | Create objects for your buttons and LEDs.
redButton = DigitalInput()
redLED = DigitalOutput()
greenButton = DigitalInput()
greenLED = DigitalOutput()
#Address | Address your four objects which lets your program know where to find them.
redButton.setHubPort(0)
redButton.setIsHubPortDevice(True)
redLED.setHubPort(1)
redLED.setIsHubPortDevice(True)
greenButton.setHubPort(5)
greenButton.setIsHubPortDevice(True)
greenLED.setHubPort(4)
greenLED.setIsHubPortDevice(True)
#Open | Connect your program to your physical devices.
redButton.openWaitForAttachment(1000)
redLED.openWaitForAttachment(1000)
greenButton.openWaitForAttachment(1000)
greenLED.openWaitForAttachment(1000)
#Use your Phidgets | This code will turn on the LED when the matching button is pressed and turns off the LED when the matching button is released. The sleep function slows down the loop so the button state is only checked every 150ms.
while(True):
if(redButton.getState()):
redLED.setState(True)
else:
redLED.setState(False)
if(greenButton.getState()):
greenLED.setState(True)
else:
greenLED.setState(False)
time.sleep(0.15)
Write Code (C#)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library | You used NuGet to install the Phidget package. The statement below give your program access to that package.
using Phidget22;
namespace GettingStarted{
class Program{
static void Main(string[] args){
//Create | Create objects for your buttons and LEDs.
DigitalInput redButton = new DigitalInput();
DigitalOutput redLED = new DigitalOutput();
DigitalInput greenButton = new DigitalInput();
DigitalOutput greenLED = new DigitalOutput();
//Address | Address your four objects which lets your program know where to find them.
redButton.HubPort = 0;
redButton.IsHubPortDevice = true;
redLED.HubPort = 1;
redLED.IsHubPortDevice = true;
greenButton.HubPort = 5;
greenButton.IsHubPortDevice = true;
greenLED.HubPort = 4;
greenLED.IsHubPortDevice = true;
//Open | Connect your program to your physical devices.
redButton.Open(1000);
redLED.Open(1000);
greenButton.Open(1000);
greenLED.Open(1000);
//Use your Phidgets | This code will turn on the LED when the matching button is pressed and turns off the LED when the matching button is released. The sleep function slows down the loop so the button state is only checked every 150ms.
while (true){
if (redButton.State){
redLED.State = true;
}else{
redLED.State = false;
}
if (greenButton.State){
greenLED.State = true;
}else{
greenLED.State = false;
}
System.Threading.Thread.Sleep(150);
}
}
}
}
Write Code (Swift)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
The window will be blank.
Add the following code and run your program.
import Cocoa
//Add Phidgets Library | You used cocoapods to install the Phidget library. The statement below give your program access to that code.
import Phidget22Swift
class ViewController: NSViewController {
//Create| Create objects for your buttons and LEDs.
let redButton = DigitalInput()
let redLED = DigitalOutput()
let greenButton = DigitalInput()
let greenLED = DigitalOutput()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Address | Address your four objects which lets your program know where to find them.
try redButton.setHubPort(0)
try redButton.setIsHubPortDevice(true)
try redLED.setHubPort(1)
try redLED.setIsHubPortDevice(true)
try greenButton.setHubPort(5)
try greenButton.setIsHubPortDevice(true)
try greenLED.setHubPort(4)
try greenLED.setIsHubPortDevice(true)
//Subscribe to events
let _ = redButton.stateChange.addHandler(red_state_change)
let _ = greenButton.stateChange.addHandler(green_state_change)
//Open | Connect your program to your physical devices.
try redButton.open()
try redLED.open()
try greenButton.open()
try greenLED.open()
}catch{
print(error)
}
}
func red_state_change(sender:DigitalInput, state: Bool){
do{
//Use your Phidgets | If the red button is pushed, turn the red LED. If the red button is released, turn the red LED off.
try redLED.setState(state)
}catch{
print(error)
}
}
func green_state_change(sender:DigitalInput, state: Bool){
do{
//Use your Phidgets | If the green button is pushed, turn the green LED. If the green button is released, turn the green LED off.
try greenLED.setState(state)
}catch{
print(error)
}
}
}
Run Your Program
When you press a button, the corresponding LED will blink.
Practice
- Modify your code so the LED turns off when the button is pressed and turns on when the button is released.
- Modify your code so the green button controls the red LED and the red button controls the green LED.
- Modify your code to the count the total number of button presses. (Hint: Make sure you have completed Lesson 2, Practice Question 3).
Practice
- Modify your code so the LED turns off when the button is pressed and turns on when the button is released.
- Modify your code so the green button controls the red LED and the red button controls the green LED.
- Modify your code to the count the total number of button presses. (Hint: Make sure you have completed Lesson 2, Practice Question 3).
Practice
- Modify your code so the LED turns off when the button is pressed and turns on when the button is released.
- Modify your code so the green button controls the red LED and the red button controls the green LED.
- Modify your code to the count the total number of button presses. (Hint: Make sure you have completed Lesson 2, Practice Question 3).
Practice
- Modify your code so the LED turns off when the button is pressed and turn on when the button is released.
- Modify your code so the green button controls the red LED and the red button controls the green LED.
- Modify your code to the count the total number of button presses.
Troubleshoot
I am getting a "Timed Out" exception.
- Make sure the USB cable from your VINT Hub to your computer is attached properly.
- Make sure you are connected to the correct ports on your VINT Hub. Red button is port 0. Red LED is port 1. Green button is port 5. Green LED is port 4.
- Make sure no other program is running that uses Phidgets. If a Phidget is already in use in another program, it will be busy and won't respond to this one.
I can't see the LED flash.
- The LED flash can be quick, make sure you are looking the the Getting Started Kit when you run your program.
- If you think you have a defective LED, try swapping it out for another (a classmates or the green LED). If you do have a defective LED, take one from your classroom's spare kits or contact us.
The button is not working properly.
- Check the button assembly. If the switch and button are not assembled correctly your program will not work properly. The plastic plunger should align with the activator as shown below.
Still having issues?
Visit the Advanced Troubleshooting Page or contact us (education@phidgets.com).