In this step, you will learn how to avoid obstacles using your Sonar Phidget.
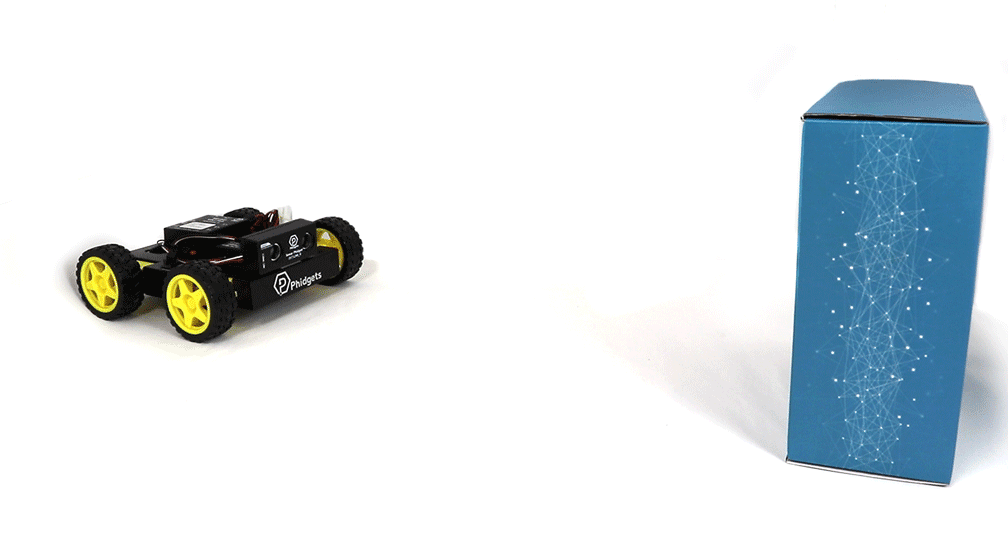
You can keep working from your PhidgetsRover project in this step. Add the following code to your file and run your code.
Write code (Java)
Not your programming language? Set your preferences so we can display relevant code examples
package phidgetsrover;
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsRover {
public static void main(String[] args) throws Exception {
//Connect to wireless rover
Net.addServer("", "192.168.100.1", 5661, "", 0);
//Create
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
DistanceSensor sonar = new DistanceSensor();
//Address
leftMotors.setChannel(0);
rightMotors.setChannel(1);
//Open
leftMotors.open(5000);
rightMotors.open(5000);
sonar.open(5000);
while (true) {
System.out.println("Distance: " + sonar.getDistance() + " mm");
if (sonar.getDistance() < 200) {
//Object detected! Stop motors
leftMotors.setTargetVelocity(0);
rightMotors.setTargetVelocity(0);
} else {
//Move forward slowly (25% max speed)
leftMotors.setTargetVelocity(0.25);
rightMotors.setTargetVelocity(0.25);
}
//Wait for 250 milliseconds
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsRover {
public static void main(String[] args) throws Exception {
//Connect to wireless rover
Net.addServer("", "192.168.100.1", 5661, "", 0);
//Create
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
DistanceSensor sonar = new DistanceSensor();
//Address
leftMotors.setChannel(0);
rightMotors.setChannel(1);
//Open
leftMotors.open(5000);
rightMotors.open(5000);
sonar.open(5000);
while (true) {
System.out.println("Distance: " + sonar.getDistance() + " mm");
if (sonar.getDistance() < 200) {
//Object detected! Stop motors
leftMotors.setTargetVelocity(0);
rightMotors.setTargetVelocity(0);
} else {
//Move forward slowly (25% max speed)
leftMotors.setTargetVelocity(0.25);
rightMotors.setTargetVelocity(0.25);
}
//Wait for 250milliseconds
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
DCMotor leftMotors;
DCMotor rightMotors;
DistanceSensor sonar;
void setup(){
try{
//Connect to wireless rover
Net.addServer("","192.168.100.1", 5661,"",0);
//Create
leftMotors = new DCMotor();
rightMotors = new DCMotor();
sonar = new DistanceSensor();
//Address
leftMotors.setChannel(0);
rightMotors.setChannel(1);
//Open
leftMotors.open(5000);
rightMotors.open(5000);
sonar.open(5000);
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
void draw(){
try{
println("Distance: " + sonar.getDistance() + " mm");
if(sonar.getDistance() < 200){
//Object detected! Stop motors
leftMotors.setTargetVelocity(0);
rightMotors.setTargetVelocity(0);
} else{
//Use your Phidgets
leftMotors.setTargetVelocity(0.25);
rightMotors.setTargetVelocity(0.25);
}
//Wait for 250 milliseconds
delay(250);
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
Write code (Python)
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets library
from Phidget22.Phidget import *
from Phidget22.Net import *
from Phidget22.Devices.DCMotor import *
from Phidget22.Devices.DistanceSensor import *
#Required for sleep statement
import time
#Connect to your rover
Net.addServer("", "192.168.100.1", 5661, "", 0)
#Create
leftMotors = DCMotor()
rightMotors = DCMotor()
sonar = DistanceSensor()
#Address
leftMotors.setChannel(0)
rightMotors.setChannel(1)
#Open
leftMotors.openWaitForAttachment(5000)
rightMotors.openWaitForAttachment(5000)
sonar.openWaitForAttachment(5000)
while(True):
print("Distance: " + str(sonar.getDistance()) + " mm")
if(sonar.getDistance() < 200):
#Object detected! Stop motors
leftMotors.setTargetVelocity(0)
rightMotors.setTargetVelocity(0)
else:
#Move forward slowly (25% full speed)
leftMotors.setTargetVelocity(0.25)
rightMotors.setTargetVelocity(0.25)
#Wait for 250 milliseconds
time.sleep(0.25)
Write code (C#)
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
namespace PhidgetsRover{
class Program{
static void Main(string[] args){
//Connect to wireless rover
Net.AddServer("", "192.168.100.1", 5661, "", 0);
//Create
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
DistanceSensor sonar = new DistanceSensor();
//Address
leftMotors.Channel = 0;
rightMotors.Channel = 1;
//Open
leftMotors.Open(5000);
rightMotors.Open(5000);
sonar.Open(5000);
while (true) {
System.Console.WriteLine("Distance: " + sonar.Distance + " mm");
if(sonar.Distance < 200) {
//Object detected! Stop motors
leftMotors.TargetVelocity = 0;
rightMotors.TargetVelocity = 0;
} else {
//Move forward slowly (25% full speed)
leftMotors.TargetVelocity = 0.25;
rightMotors.TargetVelocity = 0.25;
}
//Wait for 250 milliseconds
System.Threading.Thread.Sleep(250);
}
}
}
}
Write code (Swift)
Not your programming language? Set your preferences so we can display relevant code examples
NOTE: the code below assumes you've created a single button and linked it to an IBAction named moveRover
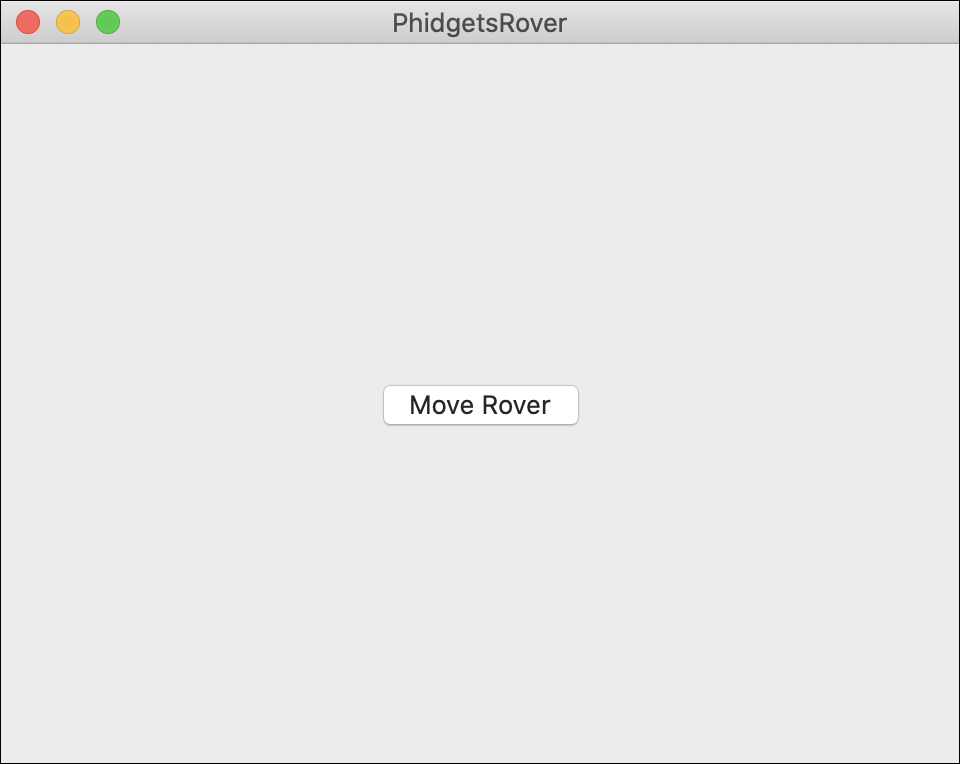
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let leftMotors = DCMotor()
let rightMotors = DCMotor()
let distanceSensor = DistanceSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Connect to wireless rover
try Net.addServer(serverName: "", address: "192.168.100.1", port: 5661, flags: 0)
//Address
try leftMotors.setChannel(0)
try rightMotors.setChannel(1)
//Subscribe to event
let _ = distanceSensor.distanceChange.addHandler(onDistanceChange)
//Open
try leftMotors.open()
try rightMotors.open()
try distanceSensor.open()
}catch{
print(error)
}
}
func onDistanceChange(sender:DistanceSensor, distance:UInt32){
do{
if(distance < 200){
//Object Detected!
try leftMotors.setTargetVelocity(0)
try rightMotors.setTargetVelocity(0)
}
else{
//Move forward slowly (25% full speed)
try leftMotors.setTargetVelocity(0.25)
try rightMotors.setTargetVelocity(0.25)
}
//Update label
DispatchQueue.main.async {
self.sensorLabel.stringValue = String(distance) + " mm"
}
}catch{
print(error)
}
}
}
Wrong Direction?
Remember, if your Rover is moving backwards instead of forwards, simply negate the Target Velocity.
Practice
- Try modifying the speed of the rover and the object detection distance.
- Instead of stopping when identifying an object, try backing up or turning.
- Modify the Sonar Phidget's Data Interval so it updates every 100 milliseconds (10 times a second) instead of every 250 milliseconds (4 times a second). Modify your loop delay to reflect the new interval. Visit the Data Interval Advanced Lesson for more information.
Troubleshoot
- Your rover should drive in the direction that the Sonar Phidget is facing. If it isn’t, simply use negative values for your Target Velocity. This will make the motors spin the opposite direction!
If you are still having issues visit Advanced Troubleshooting