Prerequisites
This project assumes you are familiar with the basic functionality of Unity. You should also review the following before moving on:
Setup
Make sure you have the following parts before getting started.
VINT Hub
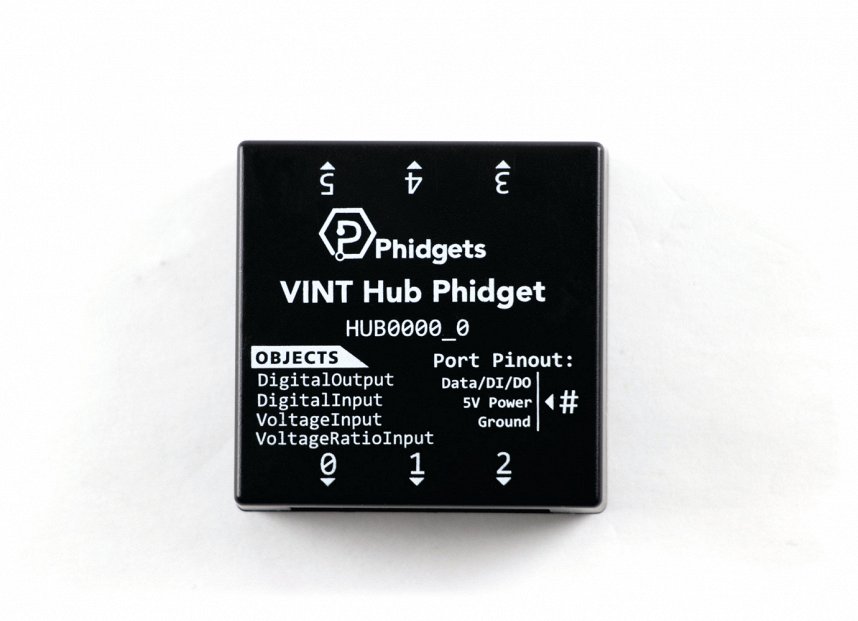
Configure Unity
In order to use Phidgets with Unity, you have to configure your project. Follow the instructions below.
Step 1
Download a copy of the Phidget libraries and unzip it as shown.
Step 2
Next, open Unity and create a new 3D project. Name it Phidgets_Unity and place it in the location of your choice.
Step 3
Navigate to the file you previously unzipped and import Phidget22.NET.dll and phidget22.dll as shown.
Note: If you are developing on macOS, you do not need to import phidget22.dll
Create Environment
Now that your project is configured, you can create your environment.
Write Code (C#)
Copy the code below into your BallScript file.
using UnityEngine;
using Phidget22;
public class BallScript : MonoBehaviour
{
private Rigidbody rb;
DigitalInput button;
Encoder dial;
bool shouldJump = false;
float x;
//Button Event
void Button_StateChange(object sender, Phidget22.Events.DigitalInputStateChangeEventArgs e)
{
shouldJump = e.State;
}
//Dial Event
void Dial_PositionChange(object sender, Phidget22.Events.EncoderPositionChangeEventArgs e){
x = e.PositionChange;
}
// Start is called before the first frame update
void Start()
{
//Connect Rigidbody
rb = GetComponent <Rigidbody>();
//Create
button = new DigitalInput();
dial = new Encoder();
//Subscribe to Events
dial.PositionChange += Dial_PositionChange;
button.StateChange += Button_StateChange;
//Open
button.Open(1000);
dial.Open(1000);
//Set data interval to minimum | This will increase the data rate from the sensor and allow for smoother movement
dial.DataInterval = dial.MinDataInterval;
}
// Update is called once per frame
void Update()
{
//Use your Phidgets
float y = shouldJump ? 250.0f : 0.0f;
shouldJump = false; //reset
Vector3 movement = new Vector3(x, y, 0.0f);
rb.AddForce(movement);
}
//Required for Unity and Phidgets
private void OnApplicationQuit()
{
button.Close();
button.Dispose();
dial.Close();
dial.Dispose();
}
}
Run Your Program
When you run your code, you will see the ball move based on the position of the dial. Press the button to make the ball jump.
Code Review
When using the Dial Phidget, you have two objects to create an use:
- Encoder
- Push Button
When using the Encoder, it's important to set the data interval to the minimum as shown in the code above. This will ensure your sensor is as responsive as possible. In this program, you are controlling the ball based on the dial movement (left or right). The easiest way to do this is to subscribe to the position change event and store the position change value as shown in the code above. If the change is positive, the ball will move to the right, if negative, it will move to the left.
When using the push button, it's a good idea to use events. Events will allow you to easily determine when the button has been pushed or released. Polling can also be used, however, extra code will be required.
Practice
- Try adding a multiplier to the position change. This will make your ball more sensitive to movement of the dial.
// Update is called once per frame
void Update()
{
//Use your Phidgets
float multiplier = 3.0f;
float y = shouldJump ? 250.0f : 0.0f;
shouldJump = false; //reset
Vector3 movement = new Vector3(x*multiplier, y, 0.0f);
rb.AddForce(movement);
}