Prerequisites
You should review the following before moving on:
Setup
Note: you can also use a Sonar Phidget, or any distance sensing Phidget for this project.
VINT Hub
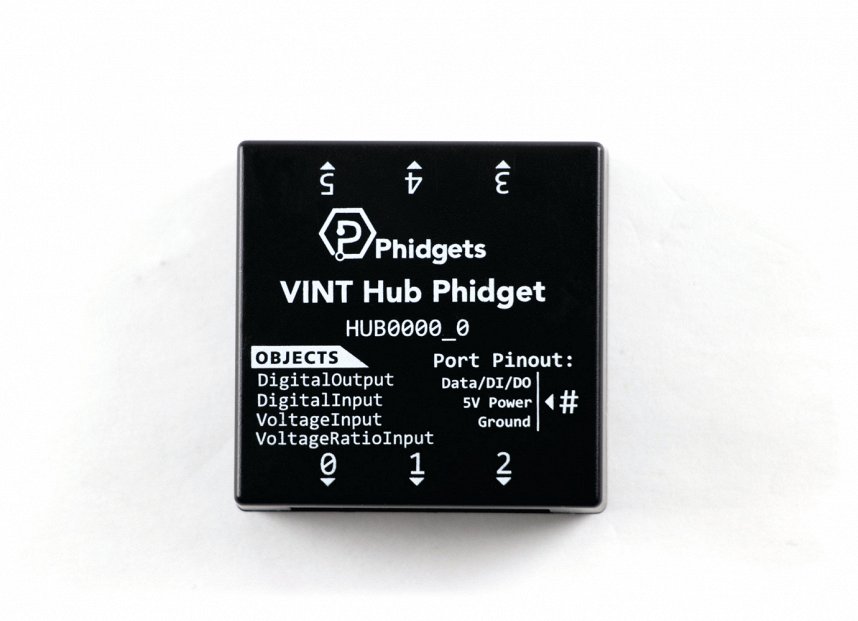
Install Pygame Zero
In order to use Pygame Zero, you first have to install it. You do this in the same way you previously installed the Phidget22 library. Simply navigate to your package manager, search for pgzero and press install!
PyCharm
If you're using PyCharm, select File > Settings > Python Interpreter and use the + symbol to install pgzero.
PyScripter
If you're using PyScripter, select Tools > Tools > Install Packages with pip and enter pgzero.
Create Project Structure
Create the following project structure on your computer in the location of your choice.
Download the following files and place them in the appropriate location.
Finally, create a new python file named phidgets_pygame.py and save it in the same location
Write Code (Python)
Copy the code below into phidgets_pygame.py.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.DistanceSensor import *
import pgzrun
WIDTH = 500
HEIGHT = 500
alien = Actor('alien')
alien.pos = WIDTH/2, HEIGHT-20
def draw():
screen.clear()
alien.draw()
def update():
#Map distance to window size
ypos = mapDistance(distanceSensor)
#Map alien to thumbstick
alien.pos = alien.pos[0], ypos
#Phidgets Code Start
def mapDistance(sensor):
try:
val = sensor.getDistance()
minD = sensor.getMinDistance()
#divide max range so it is easier to move alien from bottom to top
maxD = sensor.getMaxDistance() / 5
return HEIGHT - ((val - minD)/(maxD - minD)) * HEIGHT
except Exception as e:
print("Failure: ", e)
return alien.pos[1]
#Create, Address, Subscribe to Events and Open
distanceSensor = DistanceSensor()
distanceSensor.openWaitForAttachment(1000)
#Set data interval to minimum | This will increase the data rate from the sensor and allow for smoother movement
distanceSensor.setDataInterval(distanceSensor.getMinDataInterval())
#Phidgets Code End
pgzrun.go()
Run Your Program
When you raise and lower your hand above the distance sensor, the alien will also move.
Code Review
As you may have noticed, the program above is based on the program from the Introduction to Pygame Zero. The main difference is that the code to handle mouse clicks has been removed. To replace mouse clicks, a Distance Phidget is used.
When using a Distance Phidget, it's important to set the data interval to the minimum as shown in the code above. This will ensure your sensor is as responsive as possible. In this program, you are controlling the position of the alien with the sensor, so you must map the distance range to the window range. You can do this with the following formula:
Y = (X - A) / (B - A) * (D - C) + C
where:
- A and B are the range of the Distance Phidget (0 to 1300mm)
- C and D are the range of values you want to map to (0 to HEIGHT)
- X is the current value that falls between A and B
- Y is the value you are looking for that falls between C and D
You also may have noticed that in the mapDistance method, error handling is used. This is because distance sensors will throw an error when there is no object in their path. By surrounding the statement with a try-except, you are able to catch the error and move on.
Practice
- Try commenting out the section of your code that sets the data interval. What is the result?
- Try modifying the definition of maxD in the mapDistance method. Instead of diving by 5, try using 1, 2, and 10. What are the results?