Read Temperature
In this lesson, you’ll learn how to write a program that gets data from a temperature sensor!
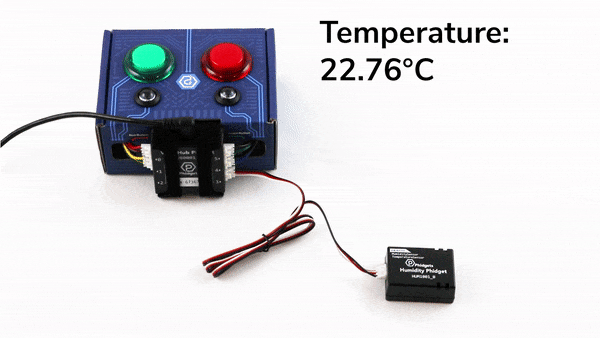
Write Code (Java)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
package gettingstarted;
//Add Phidgets Library | You added a file called phidget22 when configuring your project. Import gives you access to the Phidgets library code inside that file.
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args) throws Exception{
//Create | Here you have created a TemperatureSensor object. TemperatureSensor is a class in your Phidgets library that gathers temperature data from your Phidget.
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open | Open establishes a connection between your object and your physical Phidget. You provide a timeout value of 1000 to give the program 1000 milliseconds (1 second) to locate your Phidget. If your Phidget can't be found, an exception will be thrown.
temperatureSensor.open(1000);
//Use your Phidgets | This code will print the temperature every 150ms
while (true) {
System.out.println("Temperature: " + temperatureSensor.getTemperature() + " °C" );
Thread.sleep(150);
}
}
}
//Add Phidgets Library | You added a file called phidget22 when configuring your project. Import gives you access to the Phidgets library code inside that file.
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args) throws Exception{
//Create | Here you have created a TemperatureSensor object. TemperatureSensor is a class in your Phidgets library that gathers temperature data from your Phidget.
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open | Open establishes a connection between your object and your physical Phidget. You provide a timeout value of 1000 to give the program 1000 milliseconds (1 second) to locate your Phidget. If your Phidget can't be found, an exception will be thrown.
temperatureSensor.open(1000);
//Use your Phidgets | This code will print the temperature every 150ms
while (true) {
System.out.println("Temperature: " + temperatureSensor.getTemperature() + " °C" );
Thread.sleep(150);
}
}
}
//Add Phidgets Library | You added a file called phidget22 when configuring your project. Import gives you access to the Phidgets library code inside that file.
import com.phidget22.*;
//Define
TemperatureSensor temperatureSensor;
void setup(){
try{
//Create | Here you've created a DigitalOutput object for your LED. An object represents how you interact with your device. DigitalOutput is a class from the Phidgets library that's used to provide a voltage to things like LEDs.
temperatureSensor = new TemperatureSensor();
//Open | Open establishes a connection between your object and your physical Phidget. You provide a timeout value of 1000 to give the program 1000 milliseconds (1 second) to locate your Phidget. If your Phidget can't be found, an exception will be thrown.
temperatureSensor.open(1000);
}catch(Exception e){
//Handle Exceptions | Exceptions will happen in your code from time to time. These are caused by unexpected things happening. Make sure you’ve surrounded your code with a try-catch.
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets | Here is where you can have some fun and use your Phidgets! You can turn your LED on/off by setting the state to true/false. The sleep command keeps your LED on by letting 1000 milliseconds pass before turning the LED off.
println("Temperature: " + temperatureSensor.getTemperature() + " °C" );
delay(150);
}catch(Exception e){
//Handle Exceptions | Exceptions will happen in your code from time to time. These are caused by unexpected things happening. Make sure you’ve surrounded your code with a try-catch.
e.printStackTrace();
}
}
Write Code (Python)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library | You used Python's package manager to install the Phidget libraries on your computer. The import statements below give your program access to that code.
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
#Required for sleep statement
import time
#Create | Here you have created a TemperatureSensor object. TemperatureSensor is a class in your Phidgets library that gathers temperature data from your Phidget.
temperatureSensor = TemperatureSensor()
#Open | Open establishes a connection between your object and your physical Phidget. You provide a timeout value of 1000 to give the program 1000 milliseconds (1 second) to locate your Phidget. If your Phidget can't be found, an exception will be thrown.
temperatureSensor.openWaitForAttachment(1000)
#Use your Phidgets | This code will print the temperature every 150ms
while(True):
print(" Temperature: " + str(temperatureSensor.getTemperature()) + " °C" )
time.sleep(0.15)
Write Code (C#)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library | You used NuGet to install the Phidget package. The statement below give your program access to that package.
using Phidget22;
namespace GettingStarted{
class Program{
public static void Main(string[] args){
//Create | Here you have created a TemperatureSensor object. TemperatureSensor is a class in your Phidgets library that gathers temperature data from your Phidget.
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open | Open establishes a connection between your object and your physical Phidget. You provide a timeout value of 1000 to give the program 1000 milliseconds (1 second) to locate your Phidget. If your Phidget can't be found, an exception will be thrown.
temperatureSensor.Open(1000);
//Use your Phidgets | This code will print the temperature every 150ms
while (true){
System.Console.WriteLine("Temperature: " + temperatureSensor.Temperature + "°C");
System.Threading.Thread.Sleep(150);
}
}
}
}
Write Code (Swift)
Create a label in your window and copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
Create a label
import Cocoa
//Add Phidgets Library | You used cocoapods to install the Phidget library. The statement below give your program access to that code.
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var temperatureLabel: NSTextField!
//Create | Here you have created a TemperatureSensor object. TemperatureSensor is a class in your Phidgets library that gathers temperature data from your Phidget.
let temperatureSensor = TemperatureSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = temperatureSensor.temperatureChange.addHandler(onTemperatureChange)
//Open | Open establishes a connection between your object and your physical Phidget.
try temperatureSensor .open()
}catch{
print(error)
}
}
func onTemperatureChange(sender:TemperatureSensor, temperature: Double){
DispatchQueue.main.async {
//Use your Phidget | Update the label with the latest temperature data.
self.temperatureLabel.stringValue = String(temperature) + "°C"
}
}
}
Run Your Program
You will see the temperature displayed in degrees Celsius.
What about Addressing the Phidget?
The Humidity Phidget is a Smart Phidget. Smart Phidgets communicate to the VINT Hub and relay some basic information like what they are and where they are connected. In your previous lessons, you had to set the HubPort and the IsHubPortDevice property. With a Smart Phidget, you don't have to worry about setting those.
Practice
- Convert temperature data from degrees Celsius to degrees Fahrenheit. Hint: °F = (°C × 1.8) + 32
Troubleshoot
I am getting a "Timed Out" exception.
- Make sure the USB cable from your VINT Hub to your computer is attached properly.
- Make the Phidget cable (the black, red and white one) is connected to your VINT Hub and to your Humidity Phidget properly).
- Make sure no other program is running that uses Phidgets. If a Phidget is already in use in another program, it will be busy and won't respond to this one.
Still having issues?
Visit the Advanced Troubleshooting Page or contact us (education@phidgets.com).