Linear Encoder Guide
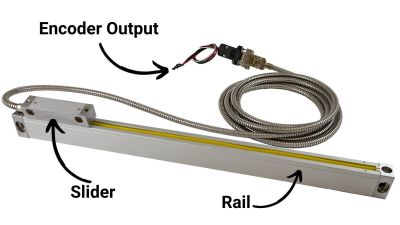
What Is a Linear Encoder?
Linear encoders provide an extremely accurate, repeatable, and cost-effective method of measuring an object's linear position and/or speed.
As the slider moves along the rail, a digital signal is generated which provides precise information about the position of the slider.
Example - Length Measurement
Which Linear Encoder Should I Use?
Phidgets Inc. currently offers eight types of linear encoders:
Product ID | Travel | Resolution | Max Pull Speed | Index Signal | ||
---|---|---|---|---|---|---|
ENC4110_0 ENC4111_0 ENC4112_0 ENC4113_0 ENC4114_0 | 300mm - 1400mm | 5µm | 1m/s | Yes | ![]() | |
ENC4115_0 ENC4116_0 ENC4117_0 | 1400mm - 2000mm | 5µm | 1m/s | Yes | ![]() |
How Do I Get Data From My Linear Encoder?
All linear encoders sold by Phidgets Inc. connect directly to our encoder interfaces.
For new designs, we recommend using the Quadrature Encoder Phidget - ENC1000_0 (pictured above). The Quadrature Encoder Phidget will monitor the output of the linear encoder and update your software with an easy-to-use encoder count value.
There are also encoder interfaces built into many of our Motor Controllers. If your project includes a motor controller, the onboard encoder interface is a great option for our linear encoders.
Converting Encoder Count to Length
TODO: Update when resolution has been updated
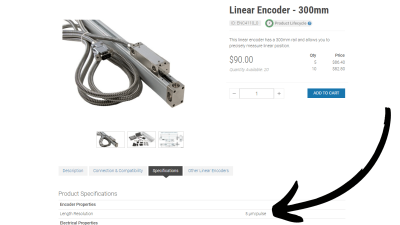
To convert from encoder pulses to length, use the resolution specification that is provided on the product page of your linear encoder.
This value can be found on the Specifications tab and is presented as micrometers per pulse. For more information, review the example code below.
Example Code
The code below is derived from the Phidget Code Sample Generator. It shows how to convert position data from an encoder interface into a length measurement.
Click on your preferred programming language to view the code example.
from Phidget22.Phidget import *
from Phidget22.Devices.Encoder import *
import time
def onPositionChange(self, positionChange, timeChange, indexTriggered):
resolution = 5E-6 #The ENC4110_0 has a resolution of 5um/pulse, replace with your product's resolution
meters = self.getPosition() * resolution
print("Length: " + str(round(meters, 3)) + " m")
def main():
encoder0 = Encoder()
encoder0.setOnPositionChangeHandler(onPositionChange)
encoder0.openWaitForAttachment(5000)
time.sleep(5)
encoder0.close()
main()
import com.phidget22.*;
import java.lang.InterruptedException;
public class Java_Example {
public static void main(String[] args) throws Exception {
Encoder encoder0 = new Encoder();
encoder0.addPositionChangeListener(new EncoderPositionChangeListener() {
public void onPositionChange(EncoderPositionChangeEvent e) {
try {
Encoder evChannel = (Encoder) e.getSource();
double resolution = 5E-6; //The ENC4110_0 has a resolution of 5um/pulse, replace with your product's resolution
double meters = evChannel.getPosition() * resolution;
System.out.println("Length: " + Math.round(meters * 1000.0) / 1000.0 + " m");
} catch (PhidgetException ex) {
ex.printStackTrace();
System.out.println("PhidgetException " + ex.getErrorCode() + " (" + ex.getDescription() + "): " + ex.getDetail());
}
}
});
encoder0.open(5000);
Thread.sleep(5000);
encoder0.close();
}
}
using System;
using Phidget22;
namespace ConsoleApplication
{
class Program
{
private static void Encoder0_PositionChange(object sender, Phidget22.Events.EncoderPositionChangeEventArgs e)
{
Phidget22.Encoder evChannel = (Phidget22.Encoder)sender;
double resolution = 5E-6; //The ENC4110_0 has a resolution of 5um/pulse, replace with your product's resolution
double meters = evChannel.Position * resolution;
Console.WriteLine("Length: " + Math.Round(meters, 3) + " m ");
}
static void Main(string[] args)
{
Encoder encoder0 = new Encoder();
encoder0.PositionChange += Encoder0_PositionChange;
encoder0.Open(5000);
System.Threading.Thread.Sleep(10000);
encoder0.Close();
}
}
}
#include <phidget22.h>
#include <stdio.h>
/* Determine if we are on Windows of Unix */
#ifdef WIN32
#include <windows.h>
#else
#include <unistd.h>
#define Sleep(x) usleep((x) * 1000)
#endif
static void CCONV onPositionChange(PhidgetEncoderHandle ch, void * ctx, int positionChange, double timeChange, int indexTriggered) {
int64_t position;
double resolution = 5E-6; //The ENC4110_0 has a resolution of 5um/pulse, replace with your product's resolution
PhidgetEncoder_getPosition(ch, &position);
double meters = resolution * position;
printf("Length: %.3f\n m", meters);
printf("----------\n");
}
int main() {
PhidgetEncoderHandle encoder0;
PhidgetEncoder_create(&encoder0);
PhidgetEncoder_setOnPositionChangeHandler(encoder0, onPositionChange, NULL);
Phidget_openWaitForAttachment((PhidgetHandle)encoder0, 5000);
Sleep(5000);
Phidget_close((PhidgetHandle)encoder0);
PhidgetEncoder_delete(&encoder0);
}
Speed Calculations
If you are interested in calculating the speed of your linear encoder, a simple Python example is provided below.
In order to implement more accurate speed calculations, review the Encoder Velocity: A Common Miscalculation guide for more information.
from Phidget22.Phidget import *
from Phidget22.Devices.Encoder import *
import time
def onPositionChange(self, positionChange, timeChange, indexTriggered):
resolution = 5E-6 #The ENC4110_0 has a resolution of 5um/pulse, replace with your product's resolution
speed = (positionChange * resolution) / (timeChange/1000.0)
print("Speed: " + str(round(speed, 3)) + " m/s")
def main():
encoder0 = Encoder()
encoder0.setOnPositionChangeHandler(onPositionChange)
encoder0.openWaitForAttachment(5000)
time.sleep(5000)
encoder0.close()
main()
Mounting and Installation
Additional Hardware
ENC4110_0 - ENC4114_0
These linear encoders come with the following hardware:
- 1x Flat carriage mounting plate
- 1x Right-angle carriage mounting plate
- Phidget encoder cable to DB9 adapter
- 1x Debris shield
- Mounting screws and washers.
- M5 Screws (2x 40mm, 2x 30mm, 4x 20mm)
- M4 Screws (4x 20mm, 5x 12mm)
- 3x Cable mounts
- 4x Metal shims
ENC4115_0 - ENC4117_0
These linear encoders come with the following hardware:
- 1x Flat carriage mounting plate
- 1x Right-angle carriage mounting plate
- Phidget encoder cable to DB9 adapter
- 1x Debris shield
- Mounting screws and washers.
- M5 Screws (6x 20mm, 2x 16mm)
- M4 Screws (2x 30mm, 4x 20mm, 4x 16mm, 9x 12mm)
- 1x Small mounting plate
- 3x Cable mounts
Mounting Linear Encoders to a Surface
All of our linear encoders have mounting holes on either end that allow them to be mounted perpendicular or parallel to a surface.
When mounting a linear encoder to a surface, ensure it lies completely flat and parallel to the moving part it will be measuring. Any small misalignment can cause inaccuracy in the measurement and strain on the mechanical parts.
Depending on your model, metal shims or a small mounting plate will be provided to ensure accurate alignment.
ENC4110_0 - ENC4114_0
ENC4115_0 - ENC4117_0
Carriage Mounting Plates
These linear encoders come with two different mounting plates for the carriage. Use the bolts to attach the carriages (washers may be required). The plate can then be attached to the moving part of your system, which should is expected to be self-supporting (don not load the carriage). The carriages can also be connected to eachother using the bolts (nuts not included).
Debris Shield and Cable Mounts
The debris shield does an excellent job of protecting the linear encoders from liquid, dust and debris. The cable mounts can be used to secure any slack in the cables.
Related Links/Guides
- Draw-Wire Sensors
- Linear Potentiometers