Draw-Wire Sensor Guide
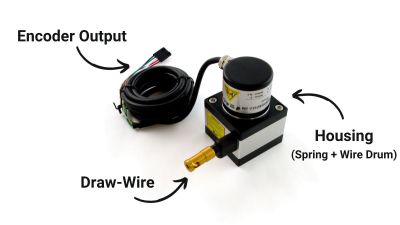
What Is a Draw-Wire Sensor?
Draw-wire sensors provide an accurate and cost-effective method of measuring an object's linear position and/or speed.
As the draw-wire is pulled from the housing, a digital signal is generated which provides precise information about the length of the extended draw-wire.
Example - Length Measurement
Which Draw-Wire Sensor Should I Use?
Phidgets Inc. currently offers two types of draw-wire sensors:
Product ID | Draw-Wire Length | Resolution | Max Pull Speed | Max Wire Load | Index Signal | ||
---|---|---|---|---|---|---|---|
ENC4118_0 | 1m | 10µm | 300mm/s | 500g | Yes | ![]() | |
ENC4119_0 | 3m | 20µm | 300mm/s | 500g | Yes | ![]() |
How Do I Get Data From My Draw-Wire Sensor?
All draw-wire sensors sold by Phidgets Inc. connect directly to our encoder interfaces.
The encoder interface will monitor the output of the draw-wire sensor and update your software with an easy-to-use encoder count value.
There are also encoder interfaces built into many of our Motor Controllers. If your project includes a motor controller, the onboard encoder interface is a great option for our draw-wire sensors.
Converting Encoder Count to Length
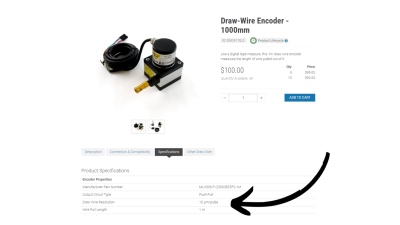
To convert from encoder pulses to length, use the resolution specification that is provided on the product page of your draw-wire sensor.
This value can be found on the Specifications tab and is presented as micrometers per pulse. For more information, review the example code below.
Example Code
The code below is derived from the Phidget Code Sample Generator. It shows how to convert position data from an encoder interface into a length measurement.
Click on your preferred programming language to view the code example.
from Phidget22.Phidget import *
from Phidget22.Devices.Encoder import *
import time
def onPositionChange(self, positionChange, timeChange, indexTriggered):
resolution = 10E-6 #The ENC4118_0 has a resolution of 10um/pulse, replace with your product's resolution
meters = self.getPosition() * resolution
print("Length: " + str(round(meters, 3)) + " m")
def main():
encoder0 = Encoder()
encoder0.setOnPositionChangeHandler(onPositionChange)
encoder0.openWaitForAttachment(5000)
time.sleep(5)
encoder0.close()
main()
import com.phidget22.*;
import java.lang.InterruptedException;
public class Java_Example {
public static void main(String[] args) throws Exception {
Encoder encoder0 = new Encoder();
encoder0.addPositionChangeListener(new EncoderPositionChangeListener() {
public void onPositionChange(EncoderPositionChangeEvent e) {
try {
Encoder evChannel = (Encoder) e.getSource();
double resolution = 10E-6; //The ENC4118_0 has a resolution of 10um/pulse, replace with your product's resolution
double meters = evChannel.getPosition() * resolution;
System.out.println("Length: " + Math.round(meters * 1000.0) / 1000.0 + " m");
} catch (PhidgetException ex) {
ex.printStackTrace();
System.out.println("PhidgetException " + ex.getErrorCode() + " (" + ex.getDescription() + "): " + ex.getDetail());
}
}
});
encoder0.open(5000);
Thread.sleep(5000);
encoder0.close();
}
}
using System;
using Phidget22;
namespace ConsoleApplication
{
class Program
{
private static void Encoder0_PositionChange(object sender, Phidget22.Events.EncoderPositionChangeEventArgs e)
{
Phidget22.Encoder evChannel = (Phidget22.Encoder)sender;
double resolution = 10E-6; //The ENC4118_0 has a resolution of 10um/pulse, replace with your product's resolution
double meters = evChannel.Position * resolution;
Console.WriteLine("Length: " + Math.Round(meters, 3) + " m ");
}
static void Main(string[] args)
{
Encoder encoder0 = new Encoder();
encoder0.PositionChange += Encoder0_PositionChange;
encoder0.Open(5000);
System.Threading.Thread.Sleep(10000);
encoder0.Close();
}
}
}
#include <phidget22.h>
#include <stdio.h>
/* Determine if we are on Windows of Unix */
#ifdef WIN32
#include <windows.h>
#else
#include <unistd.h>
#define Sleep(x) usleep((x) * 1000)
#endif
static void CCONV onPositionChange(PhidgetEncoderHandle ch, void * ctx, int positionChange, double timeChange, int indexTriggered) {
int64_t position;
double resolution = 10E-6; //The ENC4118_0 has a resolution of 10um/pulse, replace with your product's resolution
PhidgetEncoder_getPosition(ch, &position);
double meters = resolution * position;
printf("Length: %.3f\n m", meters);
printf("----------\n");
}
int main() {
PhidgetEncoderHandle encoder0;
PhidgetEncoder_create(&encoder0);
PhidgetEncoder_setOnPositionChangeHandler(encoder0, onPositionChange, NULL);
Phidget_openWaitForAttachment((PhidgetHandle)encoder0, 5000);
Sleep(5000);
Phidget_close((PhidgetHandle)encoder0);
PhidgetEncoder_delete(&encoder0);
}
Speed Calculations
If you are interested in calculating the speed of your draw-wire sensor, a simple Python example is provided below.
In order to implement more accurate speed calculations, review the Encoder Velocity: A Common Miscalculation guide for more information.
from Phidget22.Phidget import *
from Phidget22.Devices.Encoder import *
import time
def onPositionChange(self, positionChange, timeChange, indexTriggered):
resolution = 10E-6 #The ENC4118_0 has a resolution of 10um/pulse, replace with your product's resolution
speed = (positionChange * resolution) / (timeChange/1000.0)
print("Speed: " + str(round(speed, 3)) + " m/s")
def main():
encoder0 = Encoder()
encoder0.setOnPositionChangeHandler(onPositionChange)
encoder0.openWaitForAttachment(5000)
time.sleep(5000)
encoder0.close()
main()
Installation and Mounting
M5 mounting holes are available on the back of the housing and on the end of the draw wire. For more information, view the mechanical drawings for your product which can be found on the product page under the Specifications tab.