Tank Level Monitoring
Whether in food production or fuel/water usage, its good to know the volume of a liquid is in a tank. You may be surprised to learn you can do this with a Sonar Phidget! By mounting the sensor looking into the top of the tank, you can measure the distance to the tank's contents. If you know the dimension of the tank, you can calculate the volume.
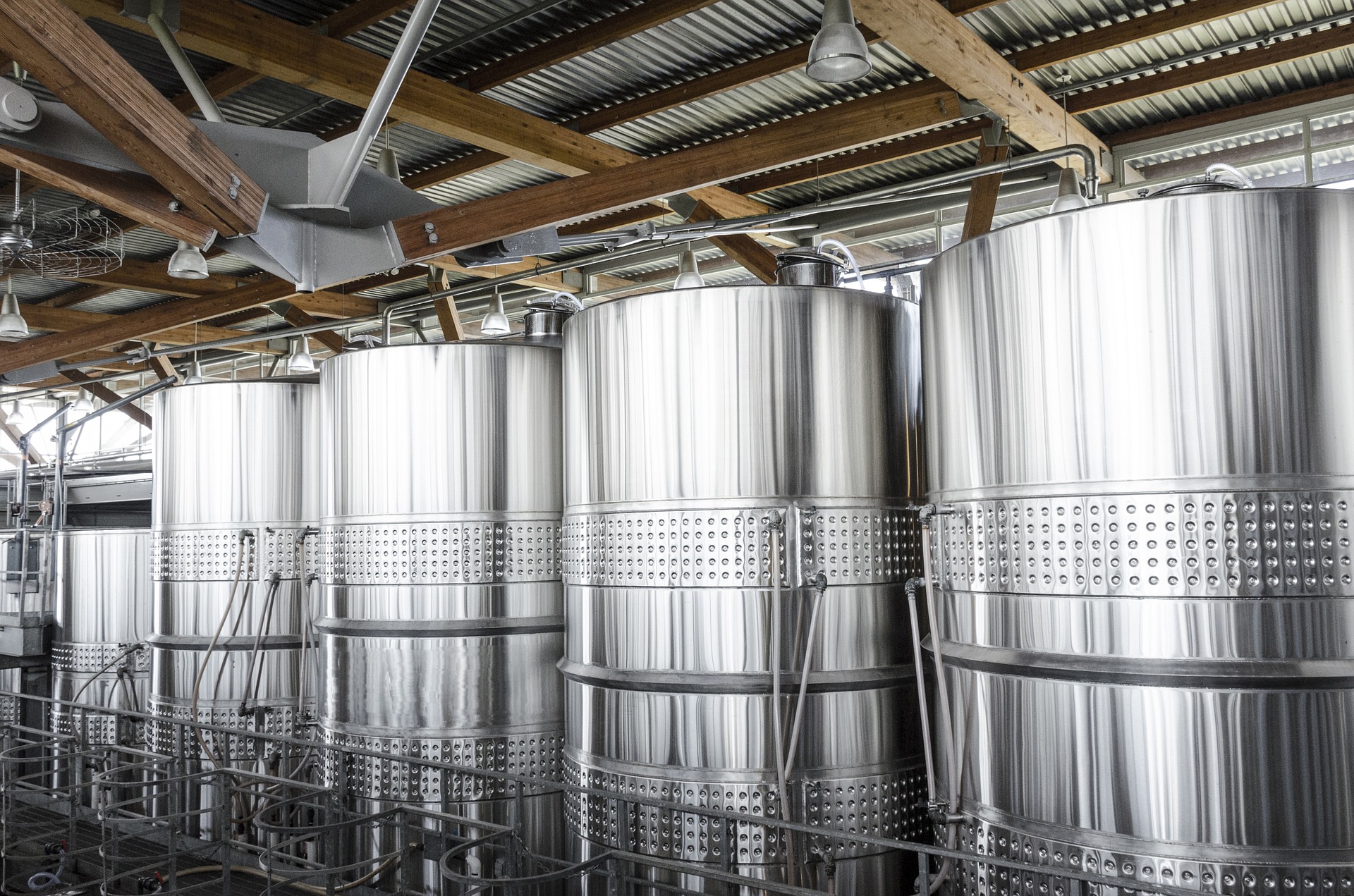
Setup
Before getting started, make sure you have the following parts.
VINT Hub
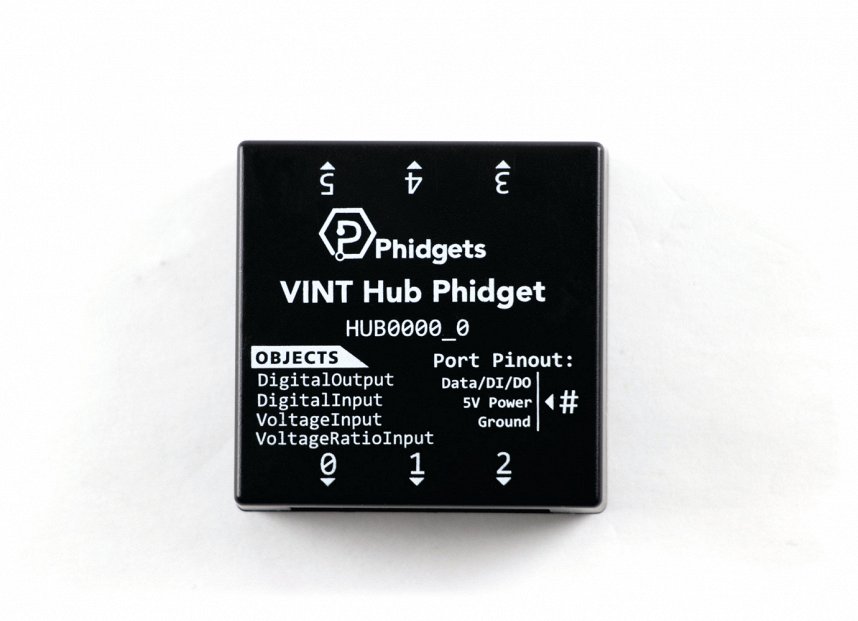
Container *
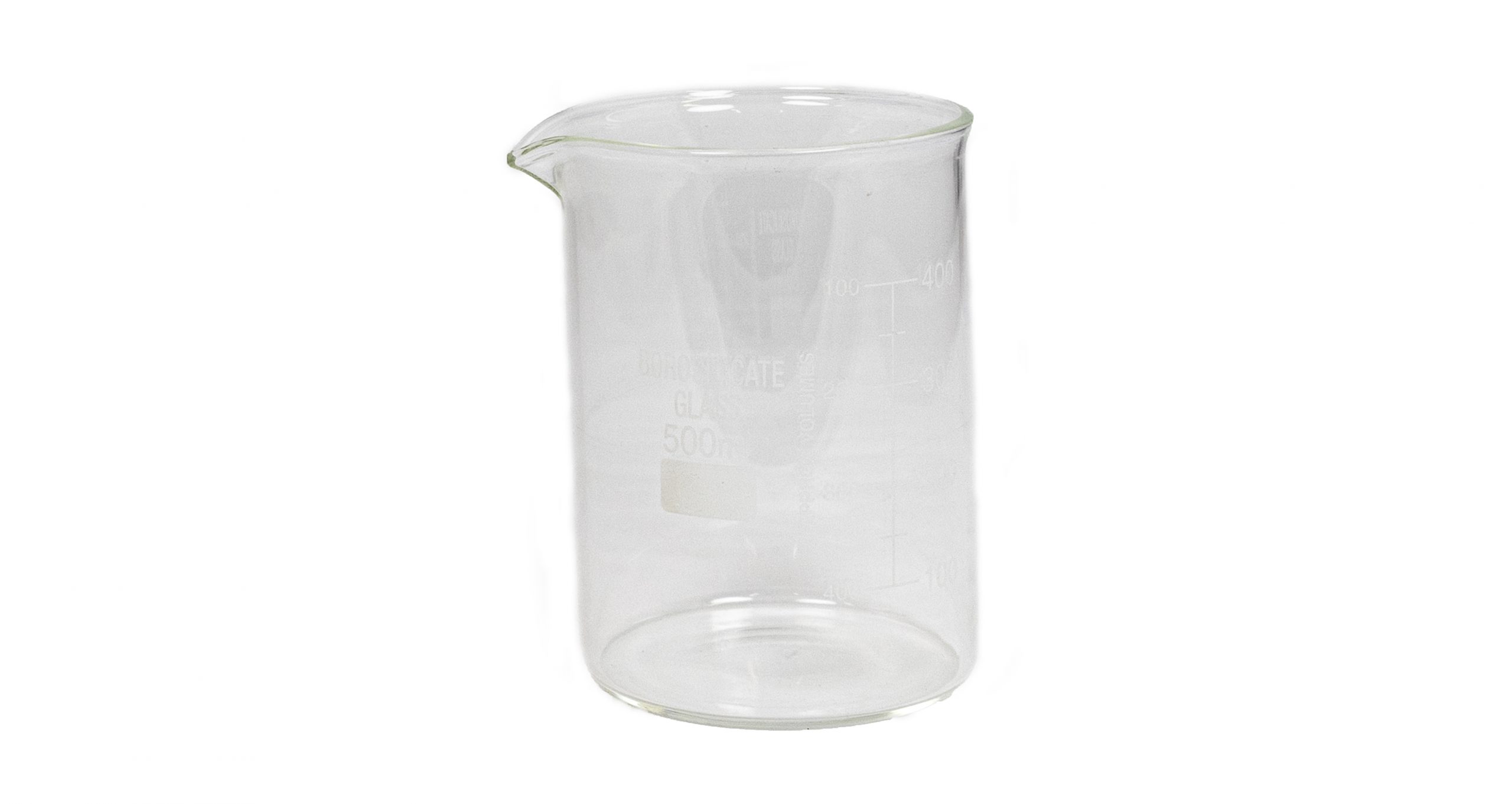
NOTE:
* Depending on the size of your container you may meed some cardboard/tape to secure the Sonar Phidget.
** Rice is easier to use than a liquid in the classroom because you do not need to worry about the Phidget or computers getting wet
Step 3
Place the sensor on top of container (do not secure the cardboard as it needs to be mobile so you can add rice to the container)
Write code (Java)
Copy the code below into a new Java project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
import com.phidget22.*;
public class TankLevel {
public static void main(String[] args) throws Exception {
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Distance: " + distanceSensor.getDistance() + " mm");
Thread.sleep(150);
}
}
}
package tanklevel;
//Add Phidgets Library
import com.phidget22.*;
public class TankLevel {
public static void main(String[] args) throws Exception {
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Distance: " + distanceSensor.getDistance() + " mm");
Thread.sleep(150);
}
}
}
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.DistanceSensor import *
#Required for sleep statement
import time
#Create
distanceSensor = DistanceSensor()
#Open
distanceSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Distance: " + str(distanceSensor.getDistance()) + " mm")
time.sleep(0.15)
Write code (C#)
Copy the code below into a new C# project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
namespace TankLevel{
class Program{
static void Main(string[] args){
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.Open(1000);
//Use your Phidgets
while(true){
System.Console.WriteLine("Distance: " + distanceSensor.Distance + " mm");
System.Threading.Thread.Sleep(150);
}
}
}
}
Write code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
import Cocoa
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var distanceLabel: NSTextField!
//Create
let distanceSensor = DistanceSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = distanceSensor.distanceChange.addHandler(distance_change)
//Open
try distanceSensor.open()
} catch {
print(error)
}
}
func distance_change(sender:DistanceSensor, distance: UInt32) {
DispatchQueue.main.async {
// Use information from your Phidget to change label
self.distanceLabel.stringValue = String(distance) + " mm"
}
}
}