Sound Controlled Rover
In this project, you will control your rover by whistling!
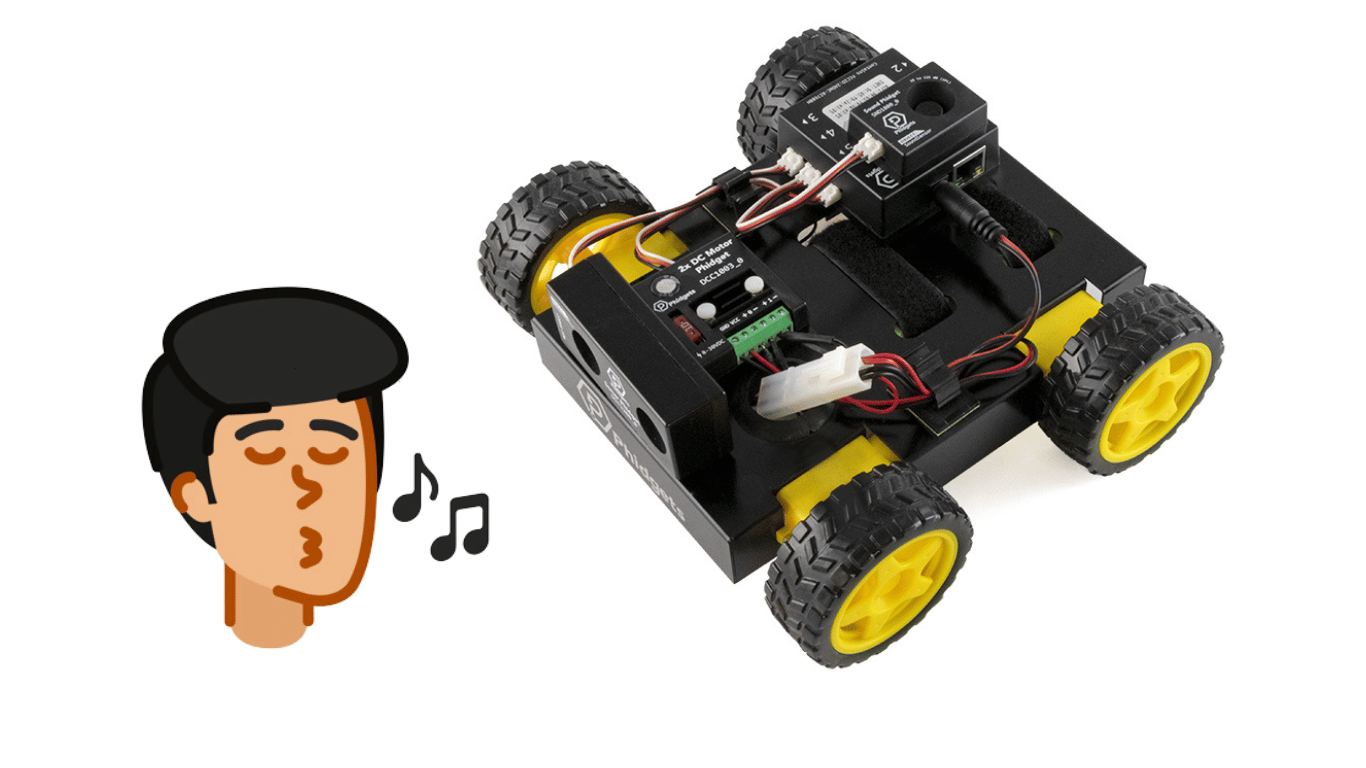
Prerequisites
Make sure you have completed the following projects before starting
Parts
Make sure you have all the parts before moving on.
Note: you can use any Phidget with an accelerometer for this project.
Setup
Step 1
You should have an assembled rover.
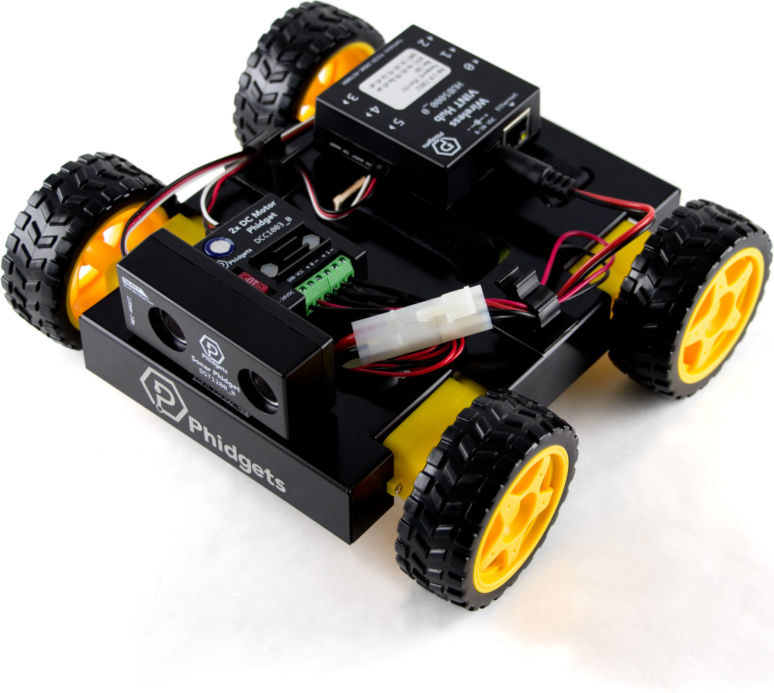
Determine the Frequency of Your Whistle
The Sound Phidget can detect the following frequencies: 31.5Hz, 63Hz, 125Hz, 250Hz, 500Hz, 1kHz, 2kHz, 4kHz, 8kHz, 16kHz.
Run the program below to determine the primary frequency of your whistle.
Code (Java)
Copy the code below into a new Java project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
import com.phidget22.*;
public class WhistleDetector {
public static double max(double[] vals){
double max = vals[0];
for(int i = 1; i < vals.length; i++){
if(vals[i] > max){
max = vals[i];
}
}
return max;
}
public static int indexof(double val, double[] arr){
for(int i = 0; i < arr.length; i++){
if(val == arr[i]){
return i;
}
}
return 0;
}
public static void main(String[] args) throws Exception{
//Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
double[] octaves_val = {31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000};
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
//Use your Phidgets
while (true) {
//Retrieve frequency band information
double[] octaves = soundSensor.getOctaves();
//Determine the dominate frequency, and return the index
int max_index = indexof(max(octaves), octaves);
System.out.println("Frequency: " + octaves_val[max_index] + " Hz");
Thread.sleep(250);
}
}
}
package whistledetector;
//Add Phidgets Library
import com.phidget22.*;
public class WhistleDetector {
public static double max(double[] vals){
double max = vals[0];
for(int i = 1; i < vals.length; i++){
if(vals[i] > max){
max = vals[i];
}
}
return max;
}
public static int indexof(double val, double[] arr){
for(int i = 0; i < arr.length; i++){
if(val == arr[i]){
return i;
}
}
return 0;
}
public static void main(String[] args) throws Exception{
//Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
double[] octaves_val = {31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000};
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
//Use your Phidgets
while (true) {
//Retrieve frequency band information
double[] octaves = soundSensor.getOctaves();
//Determine the dominate frequency, and return the index
int max_index = indexof(max(octaves), octaves);
System.out.println("Frequency: " + octaves_val[max_index] + " Hz");
Thread.sleep(250);
}
}
}
Code not available.
Code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.SoundSensor import *
#Required for sleep statement
import time
#Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
octaves_val = [31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000]
#Create
soundSensor = SoundSensor()
#Open
soundSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
#Retrieve frequency band information
octaves = soundSensor.getOctaves()
#Determine the dominant frequency, and return the index
max_index = octaves.index(max(octaves))
#Print the corresponding frequency
print("Frequency: " + str(octaves_val[max_index]) + " Hz")
time.sleep(0.25)
Code (C#)
Copy the code below into a new C# project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Phidget22;
namespace WhistleDetector
{
internal class Program
{
static void Main(string[] args)
{
//Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
double[] octaves_val = {31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000};
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.Open(1000);
//Use your Phidgets
while (true)
{
//Retrieve frequency band information
double[] octaves = soundSensor.Octaves;
//Determine the dominate frequency, and return the index
int max_index = octaves.ToList().IndexOf(octaves.Max());
System.Console.WriteLine("Frequency: " + octaves_val[max_index] + " Hz");
System.Threading.Thread.Sleep(250);
}
}
}
}
Code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
Code not available.
Control Your Rover With Your Whistle
Now that you know the frequency of your whistle, you can use it to control your rover!
Write Code (Java)
Not your programming language? Set your preferences so we can display relevant code examples
package phidgetsrover;
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsRover {
public static double max(double[] vals){
double max = vals[0];
for(int i = 1; i < vals.length; i++){
if(vals[i] > max){
max = vals[i];
}
}
return max;
}
public static int indexof(double val, double[] arr){
for(int i = 0; i < arr.length; i++){
if(val == arr[i]){
return i;
}
}
return 0;
}
public static void main(String[] args) throws Exception{
//Define
double[] octaves_val = {31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000};
//Define YOUR whistling frequency
double whistleFreq = 1000.0;
//Connect to wireless rover
Net.addServer("", "192.168.100.1", 5661, "", 0);
//Create
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
SoundSensor soundSensor = new SoundSensor();
//Address
leftMotors.setChannel(0);
rightMotors.setChannel(1);
//Open
leftMotors.open(5000);
rightMotors.open(5000);
soundSensor.open(5000);
//Increase acceleration
leftMotors.setAcceleration(leftMotors.getMaxAcceleration());
rightMotors.setAcceleration(rightMotors.getMaxAcceleration());
//Use your Phidgets
while (true){
//Retrieve frequency band information
double[] octaves = soundSensor.getOctaves();
//Determine the dominate frequency, and return the index
int max_index = indexof(max(octaves), octaves);
//If the dominant frequency matches your whistle, perform a special movement
if(whistleFreq == octaves_val[max_index]){
leftMotors.setTargetVelocity(-0.25);
rightMotors.setTargetVelocity(0.25);
Thread.sleep(1000);
leftMotors.setTargetVelocity(0);
rightMotors.setTargetVelocity(0);
}
//Wait for 250 milliseconds
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsRover {
public static double max(double[] vals){
double max = vals[0];
for(int i = 1; i < vals.length; i++){
if(vals[i] > max){
max = vals[i];
}
}
return max;
}
public static int indexof(double val, double[] arr){
for(int i = 0; i < arr.length; i++){
if(val == arr[i]){
return i;
}
}
return 0;
}
public static void main(String[] args) throws Exception{
//Define
double[] octaves_val = {31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000};
//Define YOUR whistling frequency
double whistleFreq = 1000.0;
//Connect to wireless rover
Net.addServer("", "192.168.100.1", 5661, "", 0);
//Create
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
SoundSensor soundSensor = new SoundSensor();
//Address
leftMotors.setChannel(0);
rightMotors.setChannel(1);
//Open
leftMotors.open(5000);
rightMotors.open(5000);
soundSensor.open(5000);
//Increase acceleration
leftMotors.setAcceleration(leftMotors.getMaxAcceleration());
rightMotors.setAcceleration(rightMotors.getMaxAcceleration());
//Use your Phidgets
while (true){
//Retrieve frequency band information
double[] octaves = soundSensor.getOctaves();
//Determine the dominate frequency, and return the index
int max_index = indexof(max(octaves), octaves);
//If the dominant frequency matches your whistle, perform a special movement
if(whistleFreq == octaves_val[max_index]){
leftMotors.setTargetVelocity(-0.25);
rightMotors.setTargetVelocity(0.25);
Thread.sleep(1000);
leftMotors.setTargetVelocity(0);
rightMotors.setTargetVelocity(0);
}
//Wait for 250 milliseconds
Thread.sleep(250);
}
}
}
Code sample not available.
Write Code (Python)
Not your programming language? Set your preferences so we can display relevant code examples.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Net import *
from Phidget22.Devices.SoundSensor import *
from Phidget22.Devices.DCMotor import *
#Required for sleep statement
import time
#Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
octaves_val = [31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000]
#Define YOUR whistling frequency
whistle_frequency = 1000
#Connect to your rover
Net.addServer("", "192.168.100.1", 5661, "", 0)
#Create
leftMotors = DCMotor()
rightMotors = DCMotor()
soundSensor = SoundSensor()
#Address
leftMotors.setChannel(0)
rightMotors.setChannel(1)
#Open
leftMotors.openWaitForAttachment(5000)
rightMotors.openWaitForAttachment(5000)
soundSensor.openWaitForAttachment(5000)
#Increase acceleration
leftMotors.setAcceleration(leftMotors.getMaxAcceleration())
rightMotors.setAcceleration(rightMotors.getMaxAcceleration())
#Use your Phidgets
while (True):
#Retrieve frequency band information
octaves = soundSensor.getOctaves()
#Determine the dominant frequency, and return the index
max_index = octaves.index(max(octaves))
#If the dominant frequency matches your whistle, perform a special movement
if(whistle_frequency == octaves_val[max_index]):
#Perform a special movement
leftMotors.setTargetVelocity(-0.25)
rightMotors.setTargetVelocity(0.25)
time.sleep(1)
leftMotors.setTargetVelocity(0)
rightMotors.setTargetVelocity(0)
#Wait for 250 milliseconds
time.sleep(0.25)
Write Code (C#)
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Phidget22;
namespace PhidgetsRover
{
class Program
{
static void Main(string[] args)
{
//Define
double[] octaves_val = { 31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000 };
//Define YOUR whistling frequency
double whistleFreq = 1000.0;
//Connect to wireless rover
Net.AddServer("", "192.168.100.1", 5661, "", 0);
//Create
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
SoundSensor soundSensor = new SoundSensor();
//Address
leftMotors.Channel = 0;
rightMotors.Channel = 1;
//Open
leftMotors.Open(5000);
rightMotors.Open(5000);
soundSensor.Open(5000);
//Increase acceleration
leftMotors.Acceleration = leftMotors.MaxAcceleration;
rightMotors.Acceleration = rightMotors.MaxAcceleration;
//Use your Phidgets
while (true)
{
//Retrieve frequency band information
double[] octaves = soundSensor.Octaves;
//Determine the dominate frequency, and return the index
int max_index = octaves.ToList().IndexOf(octaves.Max());
//If the dominant frequency matches your whistle, perform a special movement
if (octaves_val[max_index] == whistleFreq)
{
leftMotors.TargetVelocity = -0.25;
rightMotors.TargetVelocity = 0.25;
System.Threading.Thread.Sleep(250);
leftMotors.TargetVelocity = 0;
rightMotors.TargetVelocity = 0;
}
//Wait for 250 milliseconds
System.Threading.Thread.Sleep(250);
}
}
}
}
Write Code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
Code not available.
Run Your Program
Run your program and then whistle in the vicinity of your rover. You will see your rover perform a special movement!
Practice
- The Sound Phidget can detect the following frequencies: 31.5Hz, 63Hz, 125Hz, 250Hz, 500Hz, 1kHz, 2kHz, 4kHz, 8kHz, 16kHz. Try creating special movements for each frequency. You can use an online tone generator to generate different frequencies.
- Modify your program so the rover moves towards you when you whistle. You will need two or more Sound Phidgets for this.