Sound Level Meter
The Sound Phidget can be used to create a sound level meter. Sound level meters are important devices that provide useful information about noise in an environment. For example, many workplaces with noisy conditions must monitor the sound level to ensure their workers’ safety.
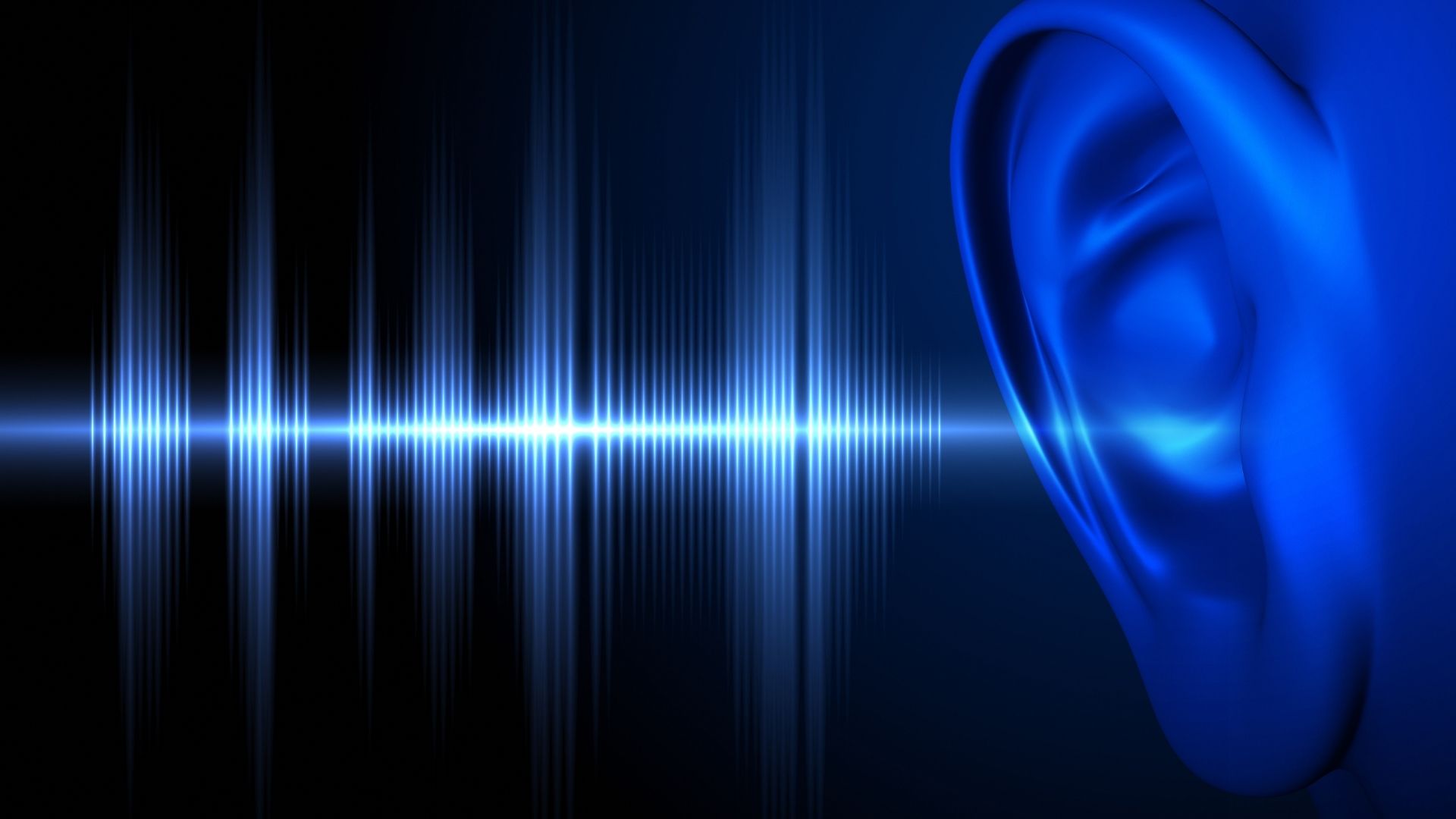
Setup
Before getting started, make sure you have the following parts.
VINT Hub
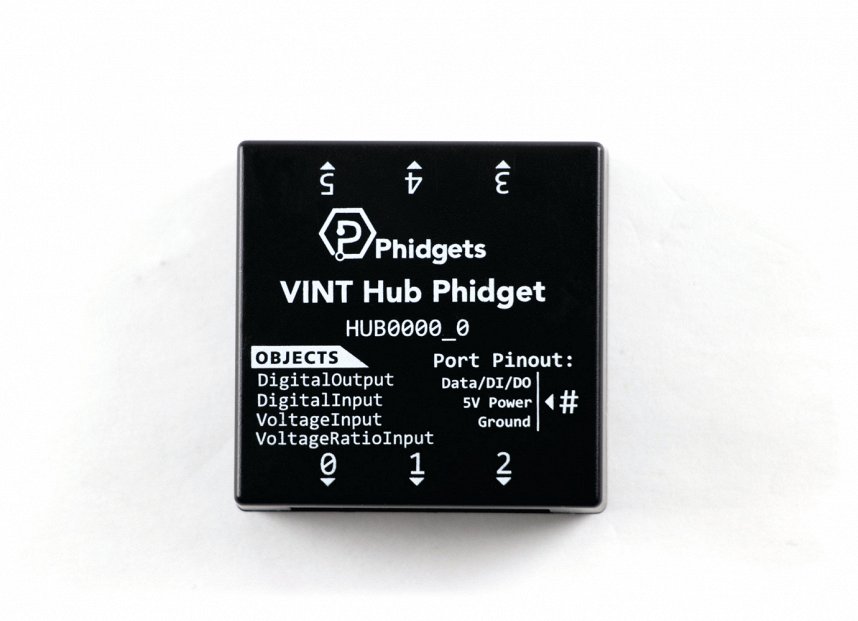
Write code (Java)
Copy the code below into a new Java project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
import com.phidget22.*;
public class SoundLevelMeter {
public static void main(String[] args) throws Exception {
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Sound pressure level: " + soundSensor.getdBA() + " dBA");
Thread.sleep(250);
}
}
}
package soundlevelmeter;
//Add Phidgets Library
import com.phidget22.*;
public class SoundLevelMeter {
public static void main(String[] args) throws Exception {
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Sound pressure level: " + soundSensor.getdBA() + " dBA");
Thread.sleep(250);
}
}
}
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.SoundSensor import *
#Required for sleep statement
import time
#Create
soundSensor = SoundSensor()
#Open
soundSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Sound pressure level: " + str(soundSensor.getdBA()) + " dBA")
time.sleep(0.25)
Write code (C#)
Copy the code below into a new C# project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
namespace SoundLevelMeter
{
class Program
{
static void Main(string[] args)
{
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("Sound pressure level: " + soundSensor.dBA + " dBA");
System.Threading.Thread.Sleep(250);
}
}
}
}
Write code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
Coming Soon!
Run your program. You will see the dBA value printed to the screen. If you make a noise near the sensor, you will see the value increase!>
What is dBA?
You may have noticed that the code above uses getdBA() instead of getdB(). Sound level meters use different frequency weightings to better understand the noise and how it impacts humans. The Sound Phidget provides the following weightings:
- dB - No weighting
- dBA - A weighting (most similar to the human ear)
- dBC - C weighting (useful for understanding loud, low frequency noises)
Practice
- Modify the above code to show the following based on the current sound level.
Sound Level (dBA) | Comparison | Program Output |
---|---|---|
<50 | Quiet Room | Sound Level: Quiet |
50-70 | Average Classroom | Sound Level: Average |
70-90 | Noisy Restaurant | Sound Level: Noisy |
90-110 | Busy Road/Highway | Sound Level: Loud |
110-130 | Rock Concert | Sound Level: Extreme |
130+ | Jet Engine | Sound Level: Dangerous! |
2. Modify your code to store the minimum and the maximum sound level. When there is a new minimum or a new maximum, print it to the screen and print the related Program Output shown above, if there is not a new minimum or maximum, show nothing. Run your program in your classroom and identify the noise range.