Security System
Have you ever been working with headphones and surprised by someone suddenly beside you? Well, you can avoid this using your Sonar Phidget to create your very own security warning system. With a Sonar Phidget, you can monitor doorways, protect an object, and prevent surprises.
You can also complete this project as a museum display activator! When a visitor comes close to your exhibit, activate audio/ visual features!
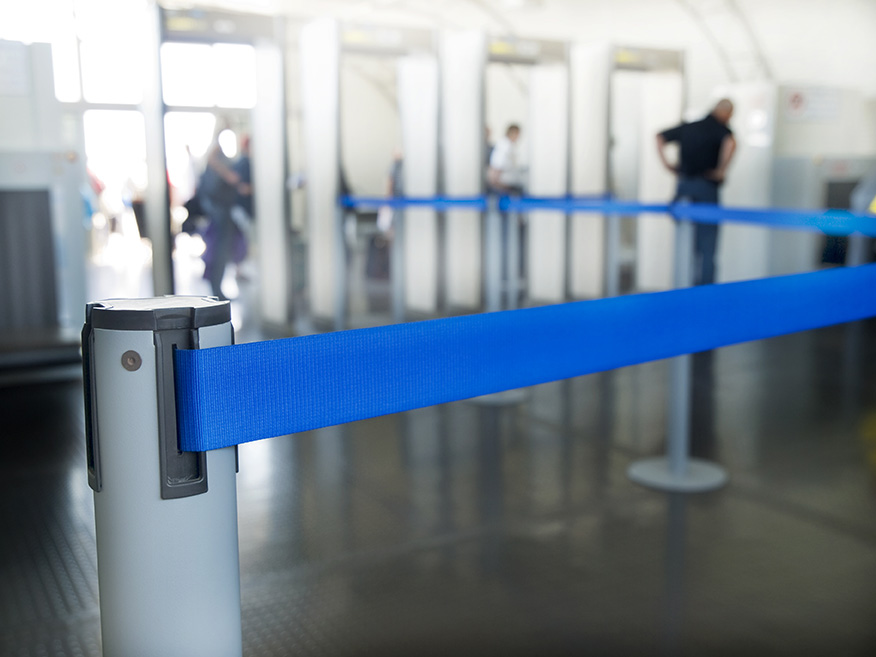
Setup
Before getting started, make sure you have the following parts.
VINT hub
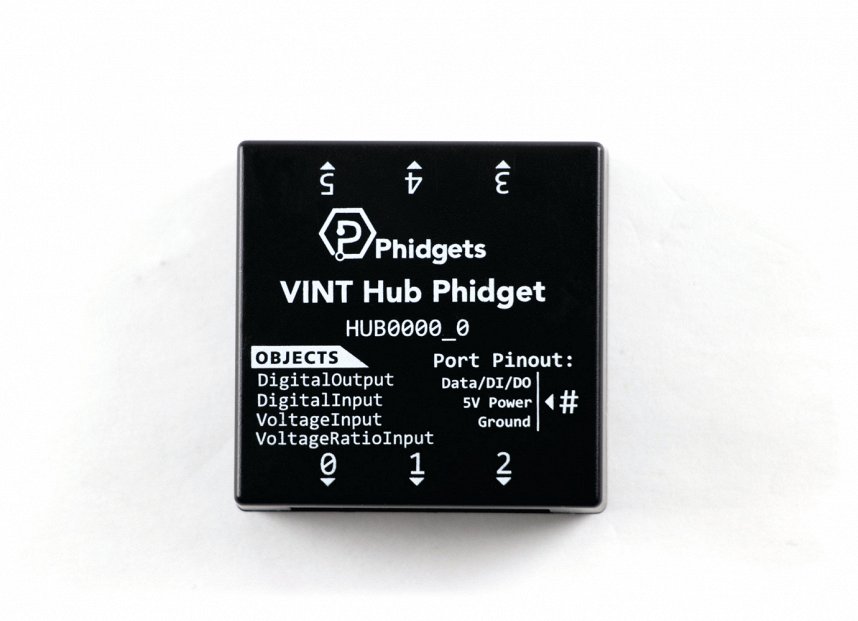
Write code (Java)
Copy the code below into a new Java project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
import com.phidget22.*;
public class SecuritySystem {
public static void main(String[] args) throws Exception {
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Distance: " + distanceSensor.getDistance() + " mm");
Thread.sleep(150);
}
}
}
package securitysystem;
//Add Phidgets Library
import com.phidget22.*;
public class SecuritySystem {
public static void main(String[] args) throws Exception {
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Distance: " + distanceSensor.getDistance() + " mm");
Thread.sleep(150);
}
}
}
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.DistanceSensor import *
#Required for sleep statement
import time
#Create
distanceSensor = DistanceSensor()
#Open
distanceSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Distance: " + str(distanceSensor.getDistance()) + " mm")
time.sleep(0.15)
Write code (C#)
Copy the code below into a new C# project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
namespace SecuritySystem{
class Program{
static void Main(string[] args){
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.Open(1000);
//Use your Phidgets
while (true){
System.Console.WriteLine("Distance: " + distanceSensor.Distance + " mm");
System.Threading.Thread.Sleep(150);
}
}
}
}
Write code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
import Cocoa
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var distanceLabel: NSTextField!
//Create
let distanceSensor = DistanceSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = distanceSensor.distanceChange.addHandler(distance_change)
//Open
try distanceSensor.open()
} catch {
print(error)
}
}
func distance_change(sender:DistanceSensor, distance: UInt32) {
DispatchQueue.main.async {
// Use information from your Phidget to change label
self.distanceLabel.stringValue = String(distance) + " mm"
}
}
}
Try running your program. You will see the distance to the closest object.
Practice
- Choose a distance to monitor -- maybe it is the width of a doorway -- and modify your code to print when an object is detected. Test the distance you set so that you are happy with the alerts.
- Modify your code so that you only receive one alert when someone is present and an alert when the area has cleared.
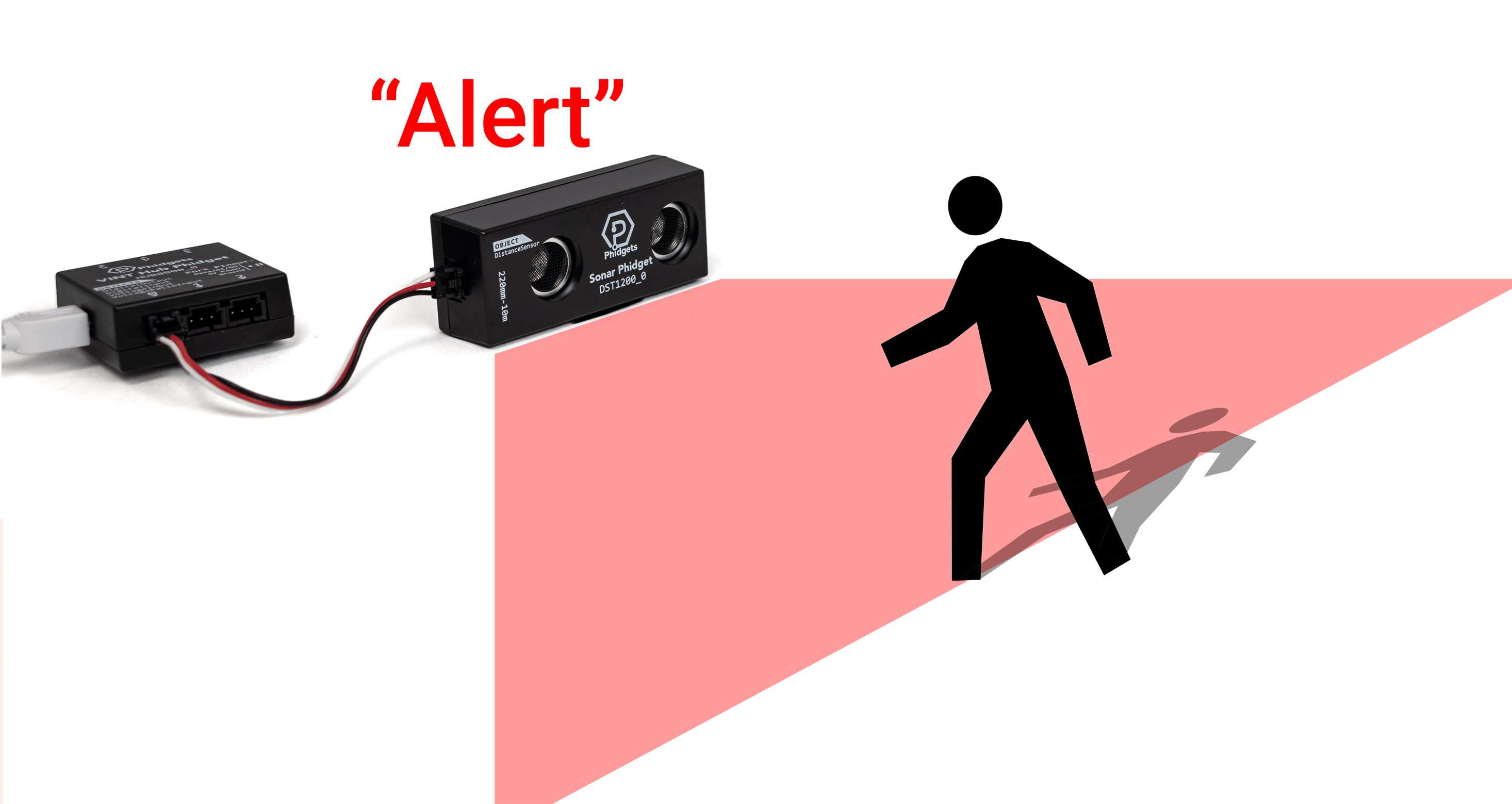