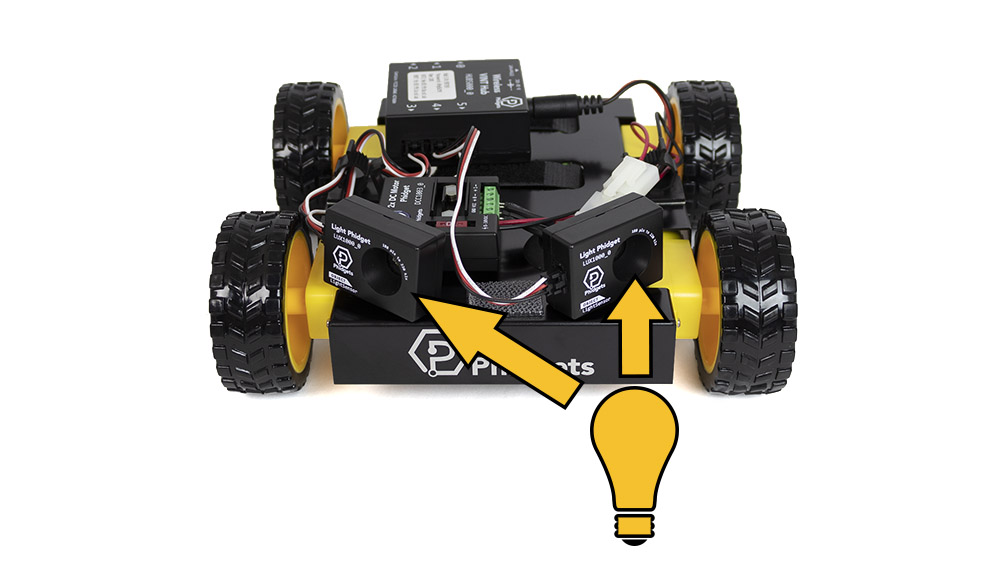
Prerequisites
Make sure you have completed the following projects before starting
Parts
Make sure you have all the parts before moving on.
Note: you can use any Phidget with an accelerometer for this project.
Setup
Step 1
You should have an assembled rover.
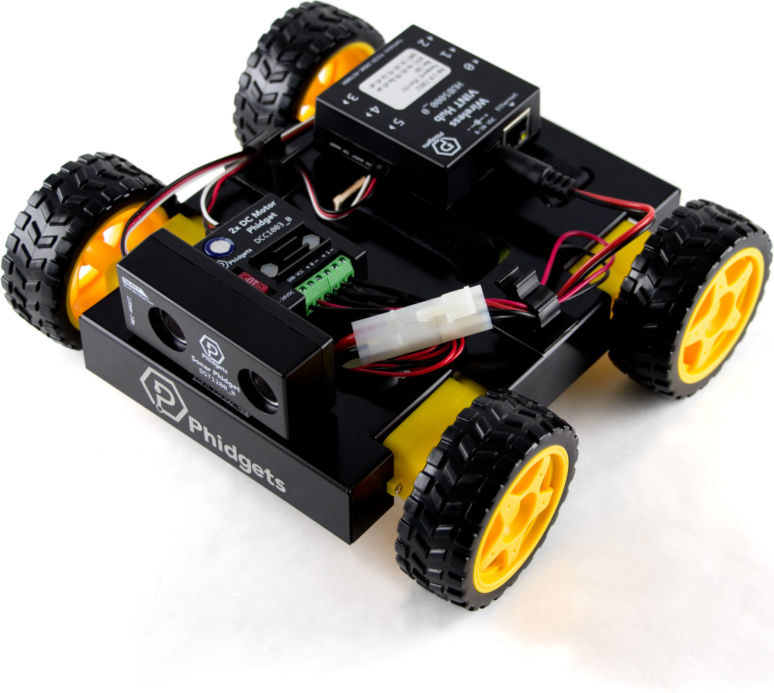
Step 2
Remove the Sonar Phidget and mount the two Light Phidgets on an angle, facing outwards.
The Light Phidget pointing to the left should be on Hub Port 4 and the other Light Phidget should be on Hub Port 3.
Move
To create an autonomous vehicle, the rover must receive all of its directions from the light sensors. By measuring the difference between the light intensity of each sensor, you can turn the rover towards the brighter side.
For example, in the image above, sensor on the right receives a greater share of light. With this input, the rover should turn to the left.
Write Code (Java)
Not your programming language? Set your preferences so we can display relevant code examples
package phidgetsrover;
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsRover {
public static void main(String[] args) throws Exception{
//Connect to wireless rover
Net.addServer("", "192.168.100.1", 5661, "", 0);
//Create
LightSensor leftLight = new LightSensor();
LightSensor rightLight = new LightSensor();
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
//Address
leftMotors.setChannel(0);
rightMotors.setChannel(1);
rightLight.setHubPort(3);
leftLight.setHubPort(4);
//Open
leftLight.open(5000);
rightLight.open(5000);
leftMotors.open(5000);
rightMotors.open(5000);
//Increase acceleration
leftMotors.setAcceleration(leftMotors.getMaxAcceleration());
rightMotors.setAcceleration(rightMotors.getMaxAcceleration());
//Use your Phidgets
while (true){
//Determine which direction to move
double lightDiff = leftLight.getIlluminance() - rightLight.getIlluminance();
System.out.println("Light Diff: " + lightDiff);
//If difference is low, do nothing
if(Math.abs(lightDiff) < 10){
leftMotors.setTargetVelocity(0);
leftMotors.setTargetVelocity(0);
}
//If there is more light to the left, go left
else if(lightDiff > 0){
leftMotors.setTargetVelocity(0.25);
rightMotors.setTargetVelocity(-0.25);
}
//If there is more light to the right, go right
else{
leftMotors.setTargetVelocity(-0.25);
rightMotors.setTargetVelocity(0.25);
}
//Wait for 250 milliseconds
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetsRover {
public static void main(String[] args) throws Exception{
//Connect to wireless rover
Net.addServer("", "192.168.100.1", 5661, "", 0);
//Create
LightSensor leftLight = new LightSensor();
LightSensor rightLight = new LightSensor();
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
//Address
leftMotors.setChannel(0);
rightMotors.setChannel(1);
rightLight.setHubPort(3);
leftLight.setHubPort(4);
//Open
leftLight.open(5000);
rightLight.open(5000);
leftMotors.open(5000);
rightMotors.open(5000);
//Increase acceleration
leftMotors.setAcceleration(leftMotors.getMaxAcceleration());
rightMotors.setAcceleration(rightMotors.getMaxAcceleration());
//Use your Phidgets
while (true){
//Determine which direction to move
double lightDiff = leftLight.getIlluminance() - rightLight.getIlluminance();
System.out.println("Light Diff: " + lightDiff);
//If difference is low, do nothing
if(Math.abs(lightDiff) < 10){
leftMotors.setTargetVelocity(0);
leftMotors.setTargetVelocity(0);
}
//If there is more light to the left, go left
else if(lightDiff > 0){
leftMotors.setTargetVelocity(0.25);
rightMotors.setTargetVelocity(-0.25);
}
//If there is more light to the right, go right
else{
leftMotors.setTargetVelocity(-0.25);
rightMotors.setTargetVelocity(0.25);
}
//Wait for 250 milliseconds
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
DCMotor leftMotors;
DCMotor rightMotors;
LightSensor leftLight;
LightSensor rightLight;
void setup(){
try{
//Connect to wireless rover
Net.addServer("","192.168.100.1", 5661,"",0);
//Create
leftMotors = new DCMotor();
rightMotors = new DCMotor();
leftLight = new LightSensor();
rightLight = new LightSensor();
//Address
leftMotors.setChannel(0);
rightMotors.setChannel(1);
rightLight.setHubPort(3);
leftLight.setHubPort(4);
//Open
leftMotors.open(5000);
rightMotors.open(5000);
leftLight.open(5000);
rightLight.open(5000);
//Increase acceleration
leftMotors.setAcceleration(leftMotors.getMaxAcceleration());
rightMotors.setAcceleration(rightMotors.getMaxAcceleration());
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
void draw(){
try{
//Determine which direction to move
double lightDiff = leftLight.getIlluminance() - rightLight.getIlluminance();
System.out.println("Light Diff: " + lightDiff);
//If difference is low, do nothing
if(Math.abs(lightDiff) < 10){
leftMotors.setTargetVelocity(0);
leftMotors.setTargetVelocity(0);
}
//If there is more light to the left, go left
else if(lightDiff > 0){
leftMotors.setTargetVelocity(0.25);
rightMotors.setTargetVelocity(-0.25);
}
//If there is more light to the right, go right
else{
leftMotors.setTargetVelocity(-0.25);
rightMotors.setTargetVelocity(0.25);
}
//Wait for 250 milliseconds
delay(250);
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
Write Code (Python)
Not your programming language? Set your preferences so we can display relevant code examples.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Net import *
from Phidget22.Devices.LightSensor import *
from Phidget22.Devices.DCMotor import *
#Required for sleep statement
import time
#Connect to your rover
Net.addServer("", "192.168.100.1", 5661, "", 0)
#Create
leftLight = LightSensor()
rightLight = LightSensor()
leftMotors = DCMotor()
rightMotors = DCMotor()
#Address
leftMotors.setChannel(0)
rightMotors.setChannel(1)
rightLight.setHubPort(3)
leftLight.setHubPort(4)
#Open
leftLight.openWaitForAttachment(5000)
rightLight.openWaitForAttachment(5000)
leftMotors.openWaitForAttachment(5000)
rightMotors.openWaitForAttachment(5000)
#Increase acceleration
leftMotors.setAcceleration(leftMotors.getMaxAcceleration())
rightMotors.setAcceleration(rightMotors.getMaxAcceleration())
#Use your Phidgets
while (True):
#Determine which direction to move
lightDiff = leftLight.getIlluminance() - rightLight.getIlluminance()
print("Light Diff:" + str(lightDiff))
#If difference is low, do nothing
if(abs(lightDiff) < 10):
leftMotors.setTargetVelocity(0)
rightMotors.setTargetVelocity(0)
#If there is more light to the left, go left
elif(lightDiff > 0):
leftMotors.setTargetVelocity(0.25)
rightMotors.setTargetVelocity(-0.25)
#If there is more light to the right, go right
else:
leftMotors.setTargetVelocity(-0.25)
rightMotors.setTargetVelocity(0.25)
#Wait for 250 milliseconds
time.sleep(0.25)
Write Code (C#)
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
namespace PhidgetsRover
{
class Program
{
static void Main(string[] args)
{
//Connect to wireless rover
Net.AddServer("", "192.168.100.1", 5661, "", 0);
//Create
LightSensor leftLight = new LightSensor();
LightSensor rightLight = new LightSensor();
DCMotor leftMotors = new DCMotor();
DCMotor rightMotors = new DCMotor();
//Address
leftMotors.Channel = 0;
rightMotors.Channel = 1;
rightLight.HubPort = 3;
leftLight.HubPort = 4;
//Open
leftLight.Open(5000);
rightLight.Open(5000);
leftMotors.Open(5000);
rightMotors.Open(5000);
//Increase acceleration
leftMotors.Acceleration = leftMotors.MaxAcceleration;
rightMotors.Acceleration = rightMotors.MaxAcceleration;
//Use your Phidgets
while (true)
{
//Determine which direction to move
double lightDiff = leftLight.Illuminance - rightLight.Illuminance;
System.Console.WriteLine("Light Diff: " + lightDiff);
//If difference is low, do nothing
if (System.Math.Abs(lightDiff) < 10)
{
leftMotors.TargetVelocity = 0;
rightMotors.TargetVelocity = 0;
}
//If there is more light to the left, go left
else if (lightDiff > 0)
{
leftMotors.TargetVelocity = 0.25;
rightMotors.TargetVelocity = -0.25;
}
//If there is more light to the right, go right
else
{
leftMotors.TargetVelocity = -0.25;
rightMotors.TargetVelocity = 0.25;
}
//Wait for 250 milliseconds
System.Threading.Thread.Sleep(250);
}
}
}
}
Write Code (Swift)
Swift code sample coming soon. Not your programming language? Set your preferences so we can display relevant code examples>
Run Your Program
Your rover will turn in the direction where more light is present. You can test this by using the flashlight on your phone. Complete the practice problems below to add more functionality to your rover.
Practice
- Adjust the velocity of the rover until you are satisfied with the turning speed.
- Add a forward velocity to your rover so it will follow the light source.
- Add the Sonar Phidget to your rover to avoid obstacles.