Setup
All you need for this project is the Getting Started Kit.
VINT Hub
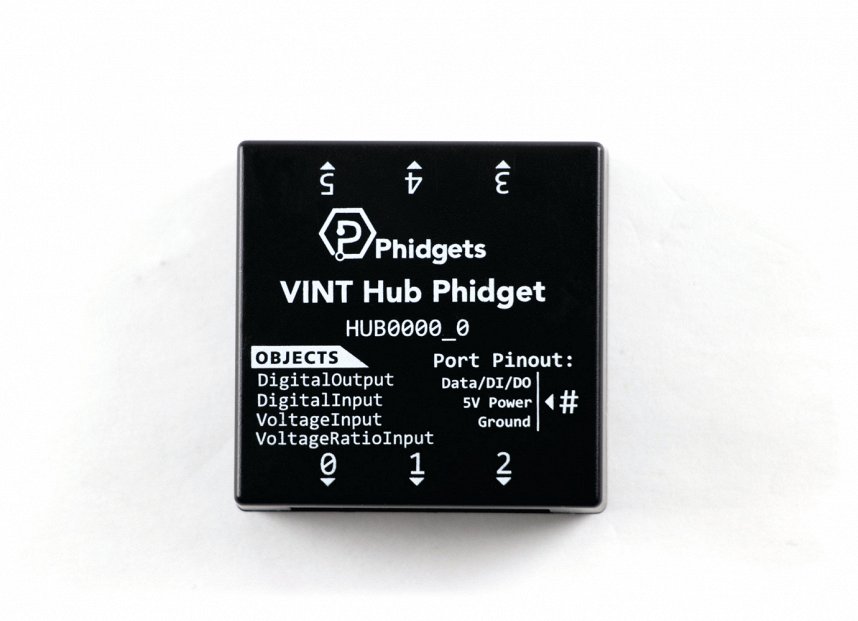
Install Pygame Zero
In order to use Pygame Zero, you first have to install it. You do this in the same way you previously installed the Phidget22 library. Simply navigate to your package manager, search for pgzero and press install!
PyCharm
If you're using PyCharm, select File > Settings > Python Interpreter and use the + symbol to install pgzero.
PyScripter
If you're using PyScripter, select Tools > Tools > Install Packages with pip and enter pgzero.
Write code (Python)
Copy the code below into your python script brick.py.
from Phidget22.Phidget import *
from Phidget22.Devices.VoltageRatioInput import *
from collections import namedtuple
from sys import exit
from random import randint
import pgzrun
import math
W = 800
H = 600
RED = 200, 0, 0
BLUE = 0, 0, 200
GREEN = 0, 200, 0
ball = Rect((W/2, H/2), (30, 30))
Direction = namedtuple('Direction', 'x y')
ball_dir = Direction(5, -5)
bat = Rect((W/2, 0.96 * H), (150, 15))
N_BLOCKS = 8
BLOCK_W = W / N_BLOCKS
BLOCK_H = BLOCK_W / 4
BLOCK_COLOURS = RED, GREEN, BLUE
class Block(Rect):
def __init__(self, colour, rect):
Rect.__init__(self, rect)
self.colour = colour
blocks = []
for n_block in range(N_BLOCKS):
colour = BLOCK_COLOURS[n_block % len(BLOCK_COLOURS)]
block = Block(colour, ((n_block * BLOCK_W, 0), (BLOCK_W, BLOCK_H)))
blocks.append(block)
def draw_blocks():
for block in blocks:
screen.draw.filled_rect(block, block.colour)
def draw():
screen.clear()
screen.draw.filled_rect(ball, RED)
screen.draw.filled_rect(bat, RED)
draw_blocks()
def on_key_down():
sys.exit()
def move(ball):
"""returns a new ball at a new position
"""
global ball_dir
ball.move_ip(ball_dir)
if ball.x > W or ball.x <= 0:
ball_dir = Direction(-1 * ball_dir.x, ball_dir.y)
if ball.y <= 0:
ball_dir = Direction(ball_dir.x, ball_dir.y * -1)
if ball.colliderect(bat):
ball_dir = Direction(ball_dir.x, - abs(ball_dir.y))
to_kill = ball.collidelist(blocks)
# # print(to_kill)
if to_kill >= 0:
ball_dir = Direction(ball_dir.x, abs(ball_dir.y))
blocks.pop(to_kill)
if not blocks:
time.sleep(1)
sys.exit()
if ball.y > H:
time.sleep(1)
sys.exit()
def update():
bat.center = (slider.getVoltageRatio()*W, bat.center[1])
move(ball)
#Phidgets Code Start
#Create
slider = VoltageRatioInput()
#Address
slider.setHubPort(0)
slider.setIsHubPortDevice(True)
#Open
slider.openWaitForAttachment(1000)
#Set Data Interval to minimum | This will increase data rate and allow for smoother movement
slider.setDataInterval(slider.getMinDataInterval())
#Phidgets Code End
pgzrun.go()
Code Review
In this example, the Slider Phidget is being used instead of the mouse. You can view the original code here to compare
Practice
- Try adding new levels to the game.
- Try adding the buttons from your Getting Started Kit to implement pause/start functionality.