Logging Sensor Data To A File
It is often useful to log data from sensors so that the data can later be reviewed and analyzed. For example, you could log the pH of a solution during a titration and analyze the graph later to find the equivalence point. Or, you could log the temperature of a server room to make sure the air conditioning system is working adequately, even when no one is there to verify.
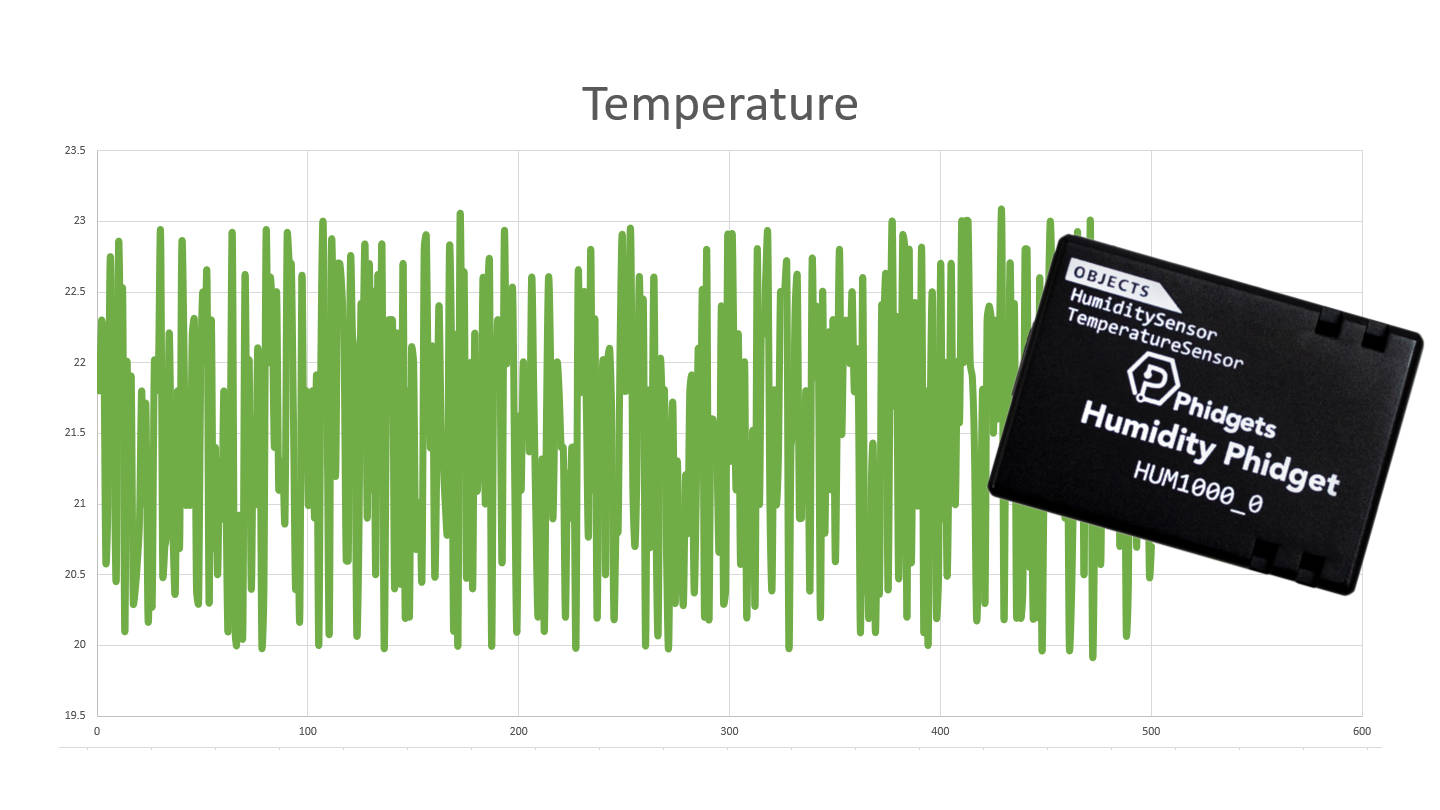
Setup
Before getting started, make sure you have the following parts.
VINT Hub
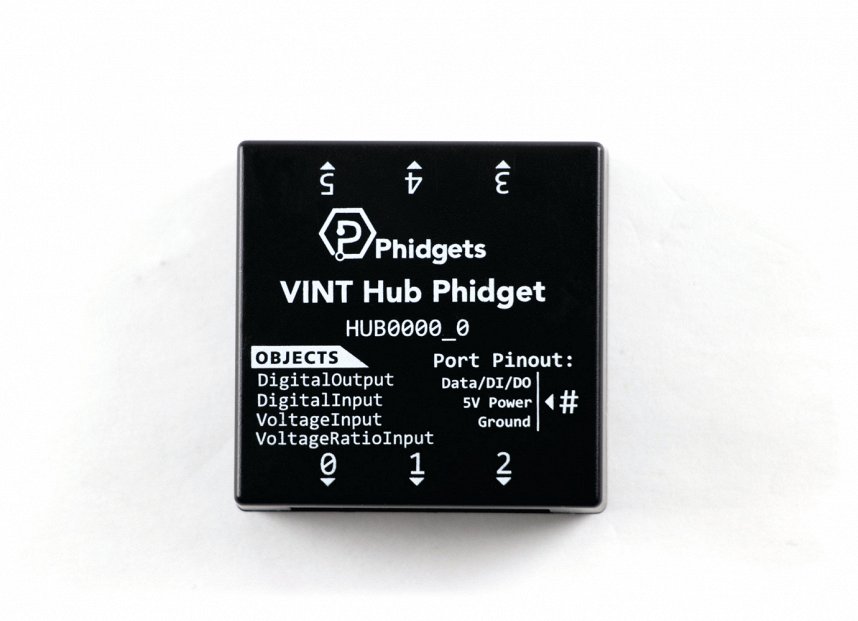
Write code (Java)
Copy the code below into a new Java project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
import com.phidget22.*;
import java.io.FileWriter;
public class LogData {
public static void main(String[] args) throws Exception{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
//Create data file
FileWriter outfile = new FileWriter("phidgets_temperature.csv");
//Record data points
int count = 0;
//Use your Phidgets
while (true) {
//Update user
System.out.println("Logging data...");
//Write data to file in CSV format
outfile.write(Double.toString(temperatureSensor.getTemperature()) + "\n");
//Increment count
count += 1;
//If 10 data points have been recorded, close file and exit program
if(count == 10){
outfile.close();
System.out.println("Logging complete, exiting program");
System.exit(0);
}
Thread.sleep(500);
}
}
}
package logdata;
import com.phidget22.*;
import java.io.FileWriter;
public class LogData {
public static void main(String[] args) throws Exception{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
//Create data file
FileWriter outfile = new FileWriter("phidgets_temperature.csv");
//Record data points
int count = 0;
//Use your Phidgets
while (true) {
//Update user
System.out.println("Logging data...");
//Write data to file in CSV format
outfile.write(Double.toString(temperatureSensor.getTemperature()) + "\n");
//Increment count
count += 1;
//If 10 data points have been recorded, close file and exit program
if(count == 10){
outfile.close();
System.out.println("Logging complete, exiting program");
System.exit(0);
}
Thread.sleep(500);
}
}
}
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets library
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
#Required for sleep statement
import time
#Create
temperatureSensor = TemperatureSensor()
#Open
temperatureSensor.openWaitForAttachment(1000)
#Record data points
count = 0
#Use your Phidgets
while (True):
#Update user
print("Logging data...")
#Write data to file in CSV format
with open ('phidgets_temperature.csv','a') as datafile:
datafile.write(str(temperatureSensor.getTemperature()) + "\n")
#Increment count
count += 1
#If 10 data points have been recorded, close file and exit program
if(count == 10):
print("Logging complete, exiting program")
exit()
time.sleep(0.5)
Write code (C#)
Copy the code below into a new C# project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
using System.IO;
namespace GettingStarted
{
class Program
{
public static void Main(string[] args)
{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.Open(1000);
//Create data file
StreamWriter outfile = new StreamWriter(@"phidgets_temperature.csv");
//Record data points
int count = 0;
//Use your Phidgets
while (true)
{
//Update user
System.Console.WriteLine("Logging data...");
//Write data to file in CSV format
outfile.WriteLine(temperatureSensor.Temperature.ToString() + "\n");
//Increment count
count += 1;
//If 10 data points have been recorded, close file and exit program
if(count == 10)
{
outfile.Close();
System.Console.WriteLine("Logging complete, exiting program");
System.Environment.Exit(0);
}
System.Threading.Thread.Sleep(500);
}
}
}
}
Write code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
Coming soon!
Run your program. The program will log 10 data points and create a new file that you can open and view afterwards. The file is generally located at same location your script is (this will vary based on language/environment).
What is a CSV and Why Use It?
CSV stands for Comma-separated Values. As the name suggests, you create a CSV file by simply writing data to a file as a string, and using commas to separate values. The benefit of using CSV files is that they are easy to generate (as shown in the code above) and you can open them with any spreadsheet program, like Microsoft Excel, to view and manipulate your data.
For example, here is a CSV file opened using Excel:
And here is the same file opened using Notepad:
As you can see, the values are simply separated with commas. When writing these files from your code, you can move to a new line by adding the newline character “\n” which is shown in the code above.
Practice
- Try opening the generated file in a spreadsheet viewing program like Microsoft Excel or Google Sheets. Next, open the file in a text editing program like Notepad on windows or TextEdit on macOS.
- Modify your program to log the time in another column next to the temperature. Open the file in a spreadsheet viewing program and generate a graph that shows the temperature data vs time. Your file should look like this:
- Create a CSV file that stores 1 data point from the temperature sensor and the humidity sensor every minute. Include a timestamp for each row. Run the program for 24 hours (or as long as you can). Do you notice any trends in the data (heating/cooling cycles, lower/higher humidity)?