Prerequisites
This project assumes you are familiar with the following
Setup
Make sure you have all the parts before moving on.
VINT Hub
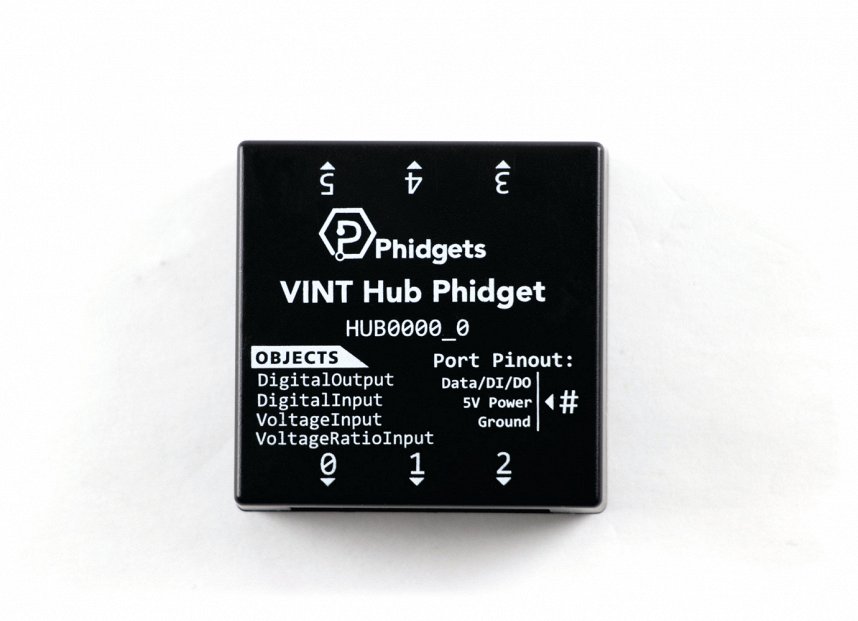
Install pynput
In order to use pynput, you first have to install it. You do this in the same way you previously installed the Phidget22 library. Simply navigate to your package manager, search for pynput and press install!
PyCharm
If you're using PyCharm, select File > Settings > Python Interpreter and use the + symbol to install pynput.
PyScripter
If you're using PyScripter, select Tools > Tools > Install Packages with pip and enter pynput.
Light Sensor Placement
The goal of this project is to create a Python script that detects when an obstacle is present and uses pynput to simulate a keyboard press. In order to detect the obstacles, you will be using a Light Phidget. You should position the Light Phidget slightly in front of your dinosaur. Some trial and error will give you a better idea of the best placement for you!
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.LightSensor import *
from pynput.keyboard import Key, Controller
#Required for sleep statement
import time
#Light Sensor Event
def onIlluminanceChange(self, illuminance):
if(illuminance < 110): #modify this value based on your screen brightness
print("Jumping")
keyboard.press(Key.space)
keyboard.release(Key.space)
print("Jumped\n")
#Create
keyboard = Controller() #this is from the pynput library
lightSensor = LightSensor()
#Subscribe to Events
lightSensor.setOnIlluminanceChangeHandler(onIlluminanceChange)
#Open
lightSensor.openWaitForAttachment(1000)
#Set Data Interval to minimum | This will increase the data rate from the sensor and make your dinosaur more responsive
lightSensor.setDataInterval(lightSensor.getMinDataInterval())
#Use your Phidgets
while (True):
time.sleep(0.1)
Run Your Program
Navigate to the dinogame, you can do this in a few ways:
- Go to https://chromedino.com/
- If you're using Chrome, paste chrome://dino into your search bar and press enter
Run your Python program and watch your dinosaur automatically jump over the obstacles!
Calibrating Your Program
In the code provided, the dinosaur will jump if the brightness is less than 110lx. This value will change depending on your screen. To figure out the best value for your program, try printing out the illuminance and watching the value as obstacles pass by. Adjust the value of 110 accordingly.
Practice
- Try adding another light sensor that works when flying obstacles are present (usually at scores of 400+).