Spatial Phidget
The Spatial Phidget contains a 3-axis accelerometer, 3-axis gyroscope and a 3-axis magnetometer.
The accelerometer measures acceleration in units of g-force. A stationary object experiences 1 g of acceleration due to the Earth's gravitational field. A high-speed roller coaster typically develops 4 g to 6 g.
The gyroscope measures rotational motion. Rotational motion (also known as angular velocity) is measured in degrees per second (°/s). The gyroscope will provide angular velocity between -2250 and 2250°/s in the x, y and z-axis. This allows you to track the orientation of an object in 3D space.
The magnetometer measures magnetic fields. The magnetometer will report magnetic field strength along the x, y, and z axes. Magnetic field strength is measured in units of Gauss (G).
See tutorial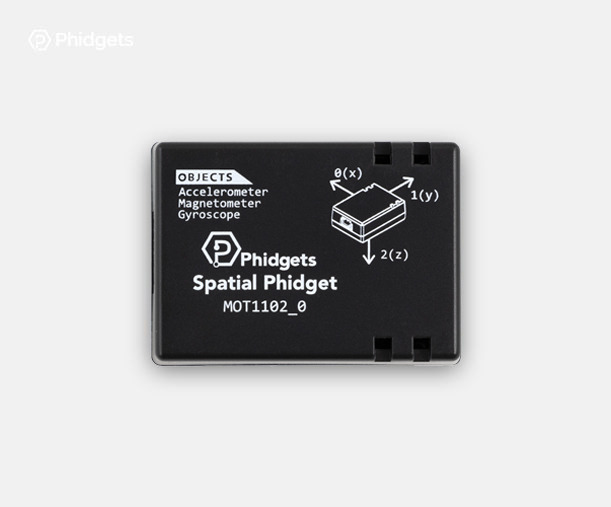
Code (Java)
Create a file called PhidgetSpatial and insert the following code. Run your code. Move your Spatial Phidget to see the output change.
Not your programming language? Set my language and IDE.
package phidgetspatial;
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetSpatial{
public static void main(String[] args) throws Exception {
//Create
Spatial spatial = new Spatial();
//Spatial Event
spatial.addSpatialDataListener(new SpatialSpatialDataListener() {
public void onSpatialData(SpatialSpatialDataEvent e) {
double[] acceleration = e.getAcceleration();
double[] angularRate = e.getAngularRate();
double[] magneticField = e.getMagneticField();
double timestamp = e.getTimestamp();
System.out.println("Acceleration: " + acceleration[0] + "," + acceleration[1] + "," + acceleration[2]);
System.out.println("Angular Rate: " + angularRate[0] + "," + angularRate[1] + "," + angularRate[2]);
System.out.println("Magnetic Field: " + magneticField[0] + "," + magneticField[1] + "," + magneticField[2]);
System.out.println("Timestamp: " + timestamp + "\n");
}
});
//Open
spatial.open(1000);
//Use your Phidgets
while (true) {
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class PhidgetSpatial{
public static void main(String[] args) throws Exception {
//Create
Spatial spatial = new Spatial();
//Spatial Event
spatial.addSpatialDataListener(new SpatialSpatialDataListener() {
public void onSpatialData(SpatialSpatialDataEvent e) {
double[] acceleration = e.getAcceleration();
double[] angularRate = e.getAngularRate();
double[] magneticField = e.getMagneticField();
double timestamp = e.getTimestamp();
System.out.println("Acceleration: " + acceleration[0] + "," + acceleration[1] + "," + acceleration[2]);
System.out.println("Angular Rate: " + angularRate[0] + "," + angularRate[1] + "," + angularRate[2]);
System.out.println("Magnetic Field: " + magneticField[0] + "," + magneticField[1] + "," + magneticField[2]);
System.out.println("Timestamp: " + timestamp + "\n");
}
});
//Open
spatial.open(1000);
//Use your Phidgets
while (true) {
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
Spatial spatial;
void setup(){
try{
//Create
spatial = new Spatial();
//Spatial Event
spatial.addSpatialDataListener(new SpatialSpatialDataListener() {
public void onSpatialData(SpatialSpatialDataEvent e) {
double[] acceleration = e.getAcceleration();
double[] angularRate = e.getAngularRate();
double[] magneticField = e.getMagneticField();
double timestamp = e.getTimestamp();
System.out.println("Acceleration: " + acceleration[0] + "," + acceleration[1] + "," + acceleration[2]);
System.out.println("Angular Rate: " + angularRate[0] + "," + angularRate[1] + "," + angularRate[2]);
System.out.println("Magnetic Field: " + magneticField[0] + "," + magneticField[1] + "," + magneticField[2]);
System.out.println("Timestamp: " + timestamp + "\n");
}
});
//Open
spatial.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
delay(250);
}
Code (Python)
Create a file called PhidgetSpatial and insert the following code. Run your code. Move your Spatial Phidget to see the output change.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.Spatial import *
#Required for sleep statement
import time
def onSpatialData(self, acceleration, angularRate, magneticField, timestamp):
print("Acceleration: " + str(acceleration))
print("Angular Rate: " + str(angularRate))
print("Magnetic Field: " + str(magneticField))
print("Timestamp: " + str(timestamp) + "\n")
#Create
spatial = Spatial()
#Subscribe to event
spatial.setOnSpatialDataHandler(onSpatialData)
#Open
spatial.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
time.sleep(0.25)
Code (C#)
Create a file called PhidgetSpatial and insert the following code. Run your code. Move your Spatial Phidget to see the output change.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace PhidgetSpatial
{
class Program
{
//Spatial Data Event
private static void SpatialSensor_SpatialData(object sender, Phidget22.Events.SpatialSpatialDataEventArgs e)
{
System.Console.WriteLine("Acceleration: " + e.Acceleration[0] + "," + e.Acceleration[1] + "," + e.Acceleration[2]);
System.Console.WriteLine("AngularRate: " + e.AngularRate[0] + "," + e.AngularRate[1] + "," + e.AngularRate[2]);
System.Console.WriteLine("MagneticField: " + e.MagneticField[0] + "," + e.MagneticField[1] + "," + e.MagneticField[2]);
System.Console.WriteLine("Timestamp: " + e.Timestamp + "\n");
}
static void Main(string[] args)
{
//Create
Spatial spatial = new Spatial();
//Subscribe to event
spatial.SpatialData += SpatialSensor_SpatialData;
//Open
spatial.Open(1000);
//Use your Phidgets
while (true)
{
System.Threading.Thread.Sleep(250);
}
}
}
}
Code (Swift)
Create a file called Spatial and insert the following code. Run your code. Move your Spatial Phidget to see the output change.
Not your programming language? Set my language and IDE.
You will need to add three Labels.
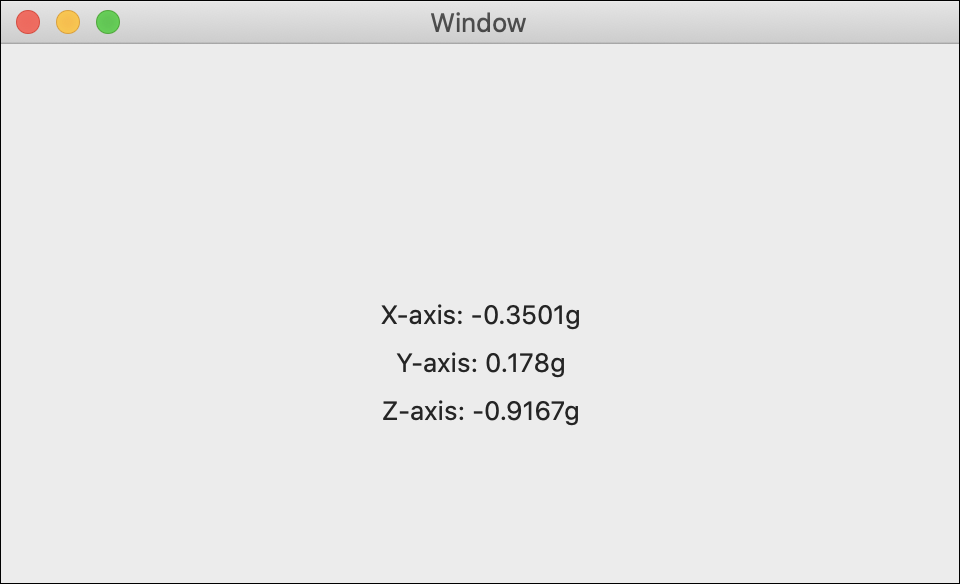
import Cocoa
//Add Phidget Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var xLabel: NSTextField!
@IBOutlet weak var yLabel: NSTextField!
@IBOutlet weak var zLabel: NSTextField!
//Create
let accelerometer = Accelerometer()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = accelerometer.accelerationChange.addHandler(onAccelerationChange)
//Open
try accelerometer.open()
}catch{
print(error)
}
}
func onAccelerationChange(sender:Accelerometer, data: (acceleration:[Double], timestamp: Double)){
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.xLabel.stringValue = "X-axis: " + String(data.acceleration[0]) + "g"
self.yLabel.stringValue = "Y-axis: " + String(data.acceleration[1]) + "g"
self.zLabel.stringValue = "Z-axis: " + String(data.acceleration[2]) + "g"
}
}
}
Applications
Spatial devices (accelerometer, gyroscope, magnetometer) are used in an incredibly wide range of applications. From monitoring orientation in smart phones, to detecting seismic activity in skyscrapers, to keeping drones flying level, you are likely interacting with spatial devices every single day!
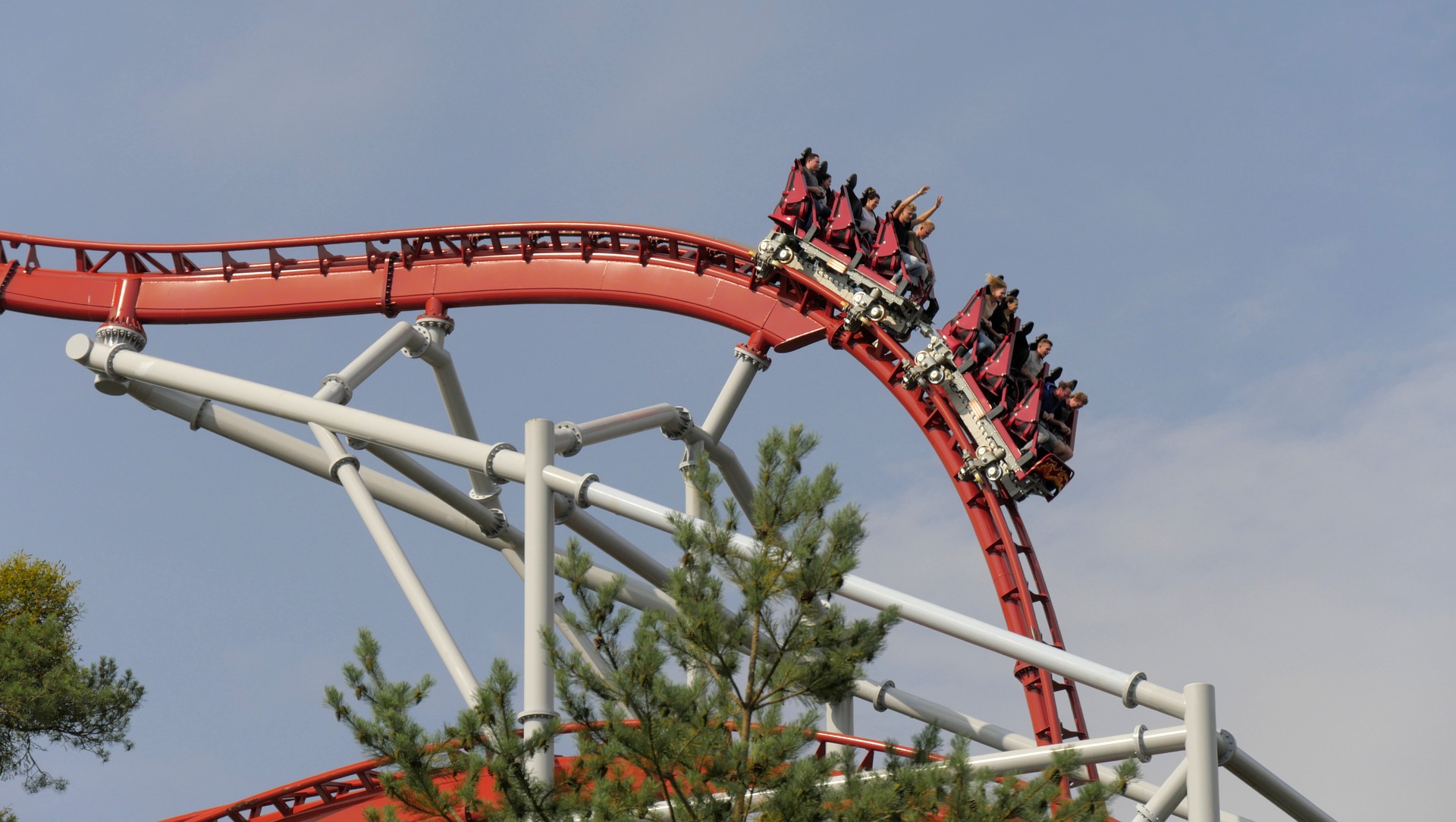
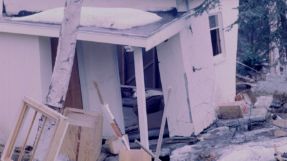
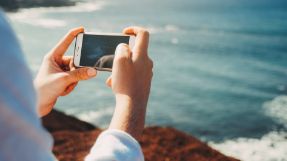
Practice
As you may have noticed, the Spatial Phidget reports data in 3 axes (x, y and z). Look at the Accelerometer data and try to understand how gravity relates to acceleration.
Check out the advanced lesson Using the Sensor API before you use the API for the first time.