Sonar Phidget
The Sonar Phidget measures distance using ultrasonic pulses. It sends out a pulse and waits for a reflection to come back. Distance is calculated by timing how long the pulse took and knowing the speed of sound.
The Sonar Phidget returns distance measured in millimeters (symbol: mm). You can easily convert this measurement into a unit you are familiar with, like meters or feet.
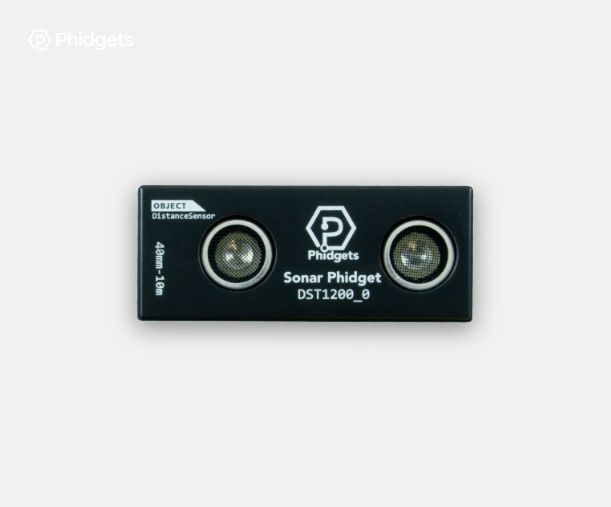
Code (Java)
Create a file called Sonar and insert the following code. Run your code. Place your hand over the sensor to see the distance change.
Not your programming language? Set my language and IDE.
package sonar;
//Add Phidgets Libary
import com.phidget22.*;
public class Sonar{
public static void main(String[] args) throws Exception {
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Distance: " + distanceSensor.getDistance() + " mm");
Thread.sleep(250);
}
}
}
//Add Phidgets Libary
import com.phidget22.*;
public class Sonar{
public static void main(String[] args) throws Exception {
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Distance: " + distanceSensor.getDistance() + " mm");
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
DistanceSensor distanceSensor;
void setup(){
try{
//Create
distanceSensor = new DistanceSensor();
//Open
distanceSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Distance: " + distanceSensor.getDistance() + " mm");
delay(250);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called Sonar and insert the following code. Run your code. Place your hand over the sensor to see the distance change.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.DistanceSensor import *
#Required for sleep statement
import time
#Create
distanceSensor = DistanceSensor()
#Open
distanceSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Distance: " + str(distanceSensor.getDistance()) + " mm")
time.sleep(0.25)
Code (C#)
Create a file called Sonar and insert the following code. Run your code. Place your hand over the sensor to see the distance change.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace Sonar{
class Program{
static void Main(string[] args){
//Create
DistanceSensor distanceSensor = new DistanceSensor();
//Open
distanceSensor.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("Distance: " + distanceSensor.Distance + " mm");
System.Threading.Thread.Sleep(250);
}
}
}
}
Code (Swift)
Create a file called Sonar and insert the following code. Run your code. Place your hand over the sensor to see the distance change.
Not your programming language? Set my language and IDE.
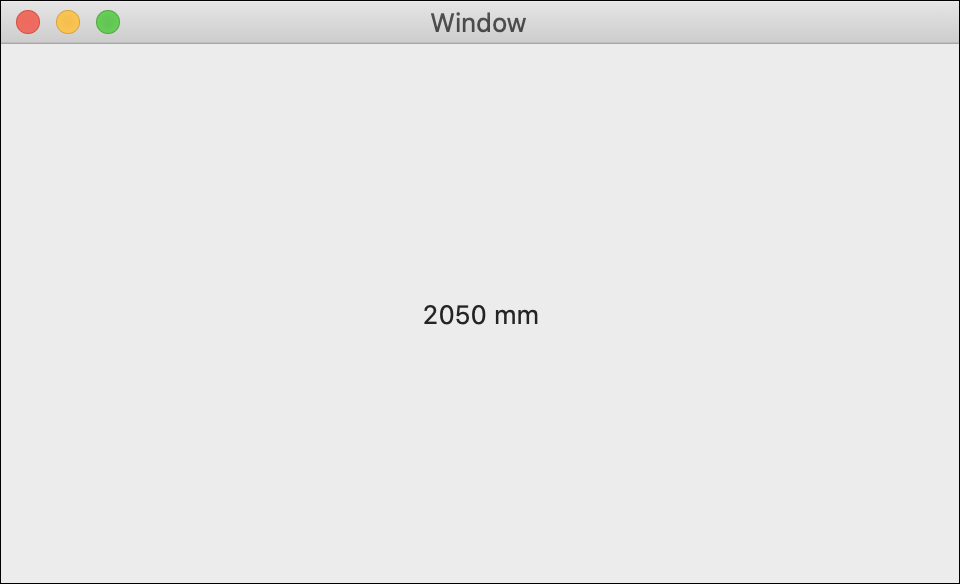
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let distanceSensor = DistanceSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = distanceSensor.distanceChange.addHandler(onDistanceChange)
//Open
try distanceSensor.open()
}catch{
print(error)
}
}
func onDistanceChange(sender:DistanceSensor, distance:UInt32){
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.sensorLabel.stringValue = String(distance) + " mm"
}
}
}
Applications
Sonar sensors are used in a wide variety of applications:
- Robots use sonar sensors to aid in navigation.
- Modern cars use sonar sensors to detect other cars and objects to avoid collisions
- Production lines use sonar sensors to determine when an object is present at a station.
- Museum exhibits use sonar sensors to detect visitors and turn on displays.
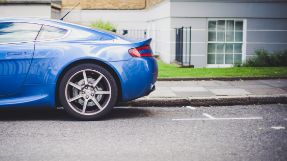
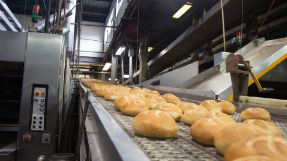
Practice
You can simulate an interactive museum display using your Getting Started Kit and your Sonar Phidget.
- Attach your Sonar Phidget to your VINT Hub in your Getting Started Kit, making sure you can access the Sonar Phidget outside of the box.
- Write a program to activate your LEDs and temperature sensor when an object is within 50 cm of your distance sensor.
- Now, set the box and distance sensor up at the edge of your desk. If someone sits down at your desk, your program will come alive.
Check out the advanced lesson Using the Sensor API before you use the API for the first time.