Slider Phidget
The Slider Phidget is ideal for user input. It has 60mm (2.4") of travel and connects directly to your VINT Hub.
This Phidget returns a value between 0 and 1 depending on the position of the slider.
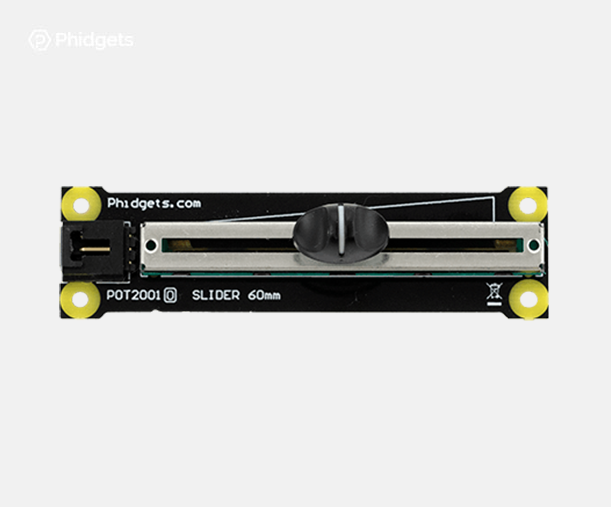
Code (Java)
Create a file called Slider and add the following code. Run your code. Move the slider to see the output change.
Not your programming language? Set my language and IDE.
package slider;
//Add Phidgets Library
import com.phidget22.*;
public class Slider {
public static void main(String[] args) throws Exception{
//Create
VoltageRatioInput slider = new VoltageRatioInput();
//Address
slider.setHubPort(0);
slider.setIsHubPortDevice(true);
//Open
slider.open(1000);
//Use your Phidget
while (true) {
System.out.println("Slider Value: " + slider.getVoltageRatio());
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class Slider {
public static void main(String[] args) throws Exception{
//Create
VoltageRatioInput slider = new VoltageRatioInput();
//Address
slider.setHubPort(0);
slider.setIsHubPortDevice(true);
//Open
slider.open(1000);
//Use your Phidget
while (true) {
System.out.println("Slider Value: " + slider.getVoltageRatio());
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
VoltageRatioInput slider;
void setup(){
try{
//Create
slider = new VoltageRatioInput();
//Address
slider.setHubPort(0);
slider.setIsHubPortDevice(true);
//Open
slider.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
System.out.println("Slider Value: " + slider.getVoltageRatio());
delay(250);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called Slider and add the following code. Run your code. Move the slider to see the output change.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.VoltageRatioInput import *
#Required for sleep statement
import time
#Create
slider = VoltageRatioInput()
#Address
slider.setHubPort(0)
slider.setIsHubPortDevice(True)
#Open
slider.openWaitForAttachment(5000)
#Use your Phidgets
while(True):
print("Slider Value: " + str(slider.getVoltageRatio()))
time.sleep(0.25)
Code (C#)
Create a file called Slider and add the following code. Run your code. Move the slider to see the output change.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace Slider
{
class Program
{
static void Main(string[] args)
{
//Create
VoltageRatioInput slider = new VoltageRatioInput();
//Address
slider.HubPort = 0;
slider.IsHubPortDevice = true;
//Open
slider.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("Slider Value: " + slider.VoltageRatio);
System.Threading.Thread.Sleep(250);
}
}
}
}
Code (Swift)
Create a file called Slider and add the following code. Run your code. Move the slider to see the output change.
Not your programming language? Set my language and IDE.
Add two buttons.
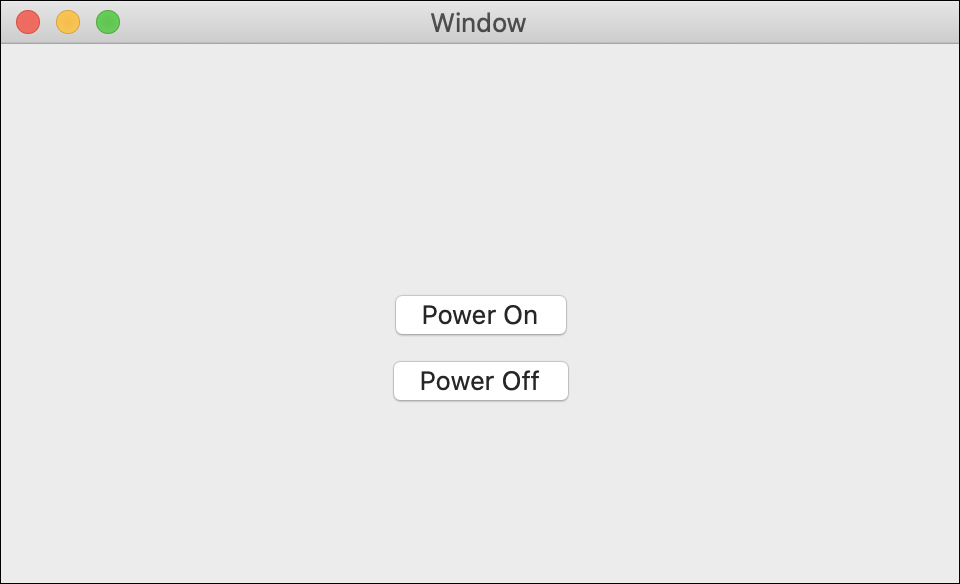
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
//Create
let powerPlug = DigitalOutput()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Address
try powerPlug.setHubPort(0)
try powerPlug.setIsHubPortDevice(true)
//Open
try powerPlug.open()
}catch{
print(error)
}
}
@IBAction func turnPowerOn(_ sender: Any) {
do{
try powerPlug.setState(true)
}catch{
print(error)
}
}
@IBAction func turnPowerOff(_ sender: Any) {
do{
try powerPlug.setState(false)
}catch{
print(error)
}
}
}
Applications
The Slider Phidget is a linear potentiometer. Potentiometers are commonly used to control a variety of electrical devices. For example, you have likely adjusted a radio using a potentiometer.
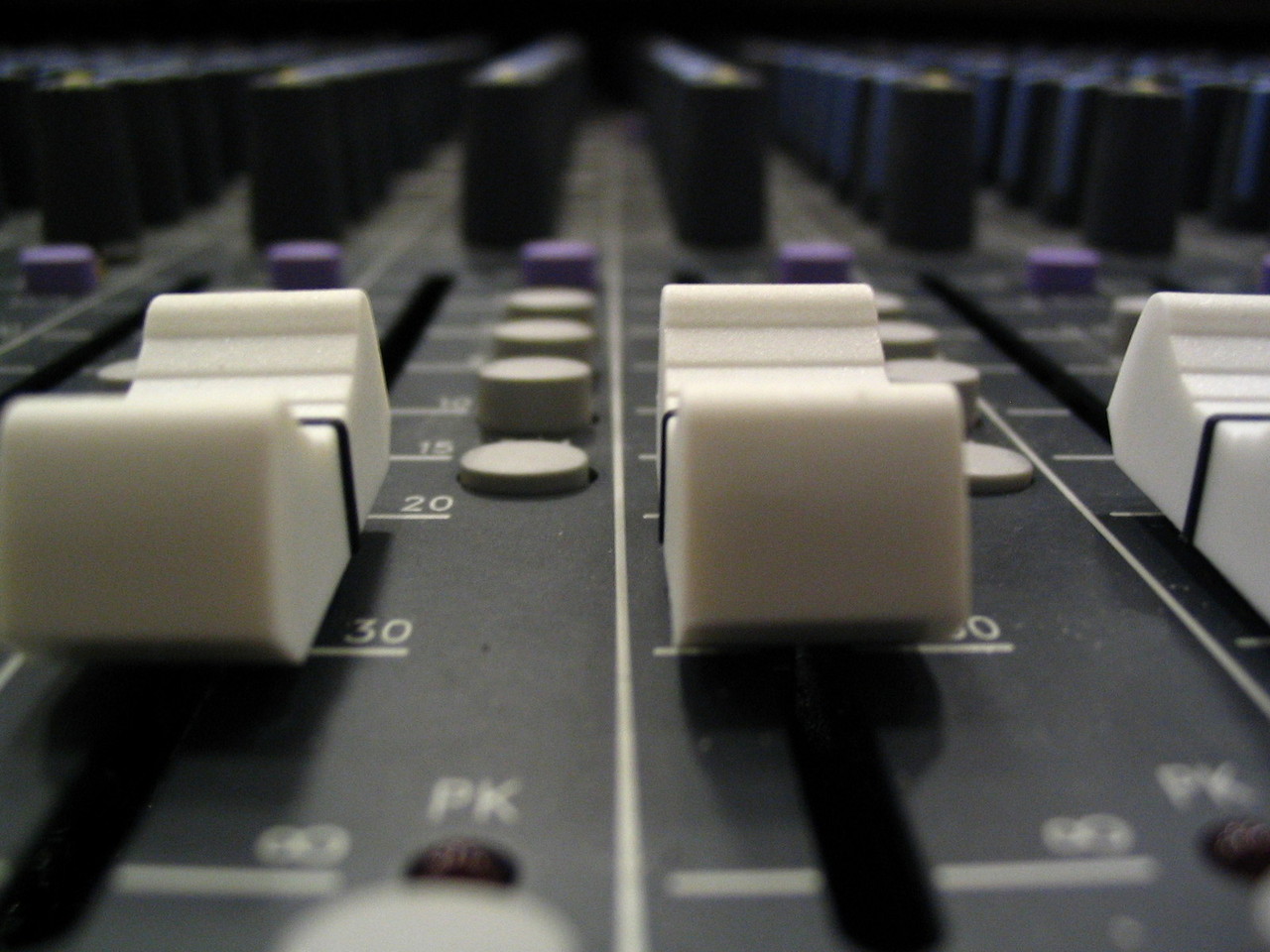
Practice
Use the Slider Phidget to control the LEDs on your Getting Started Kit. Modify the brightness of the LEDs based on the position of the slider. For more information, visit the Advanced Lesson on LED Brightness.
Check out the advanced lesson Using the Sensor API before you use the API for the first time.
API
This device doesn’t have an API of its own. It is controlled by opening a VoltageRatioInput on the a VINT Hub.