Barometer Phidget
The Barometer Phidget measures the absolute pressure of its surroundings. The Phidget measures pressure in kilopascals (kPa). The device would return about 101 kPA at sea level.
See tutorial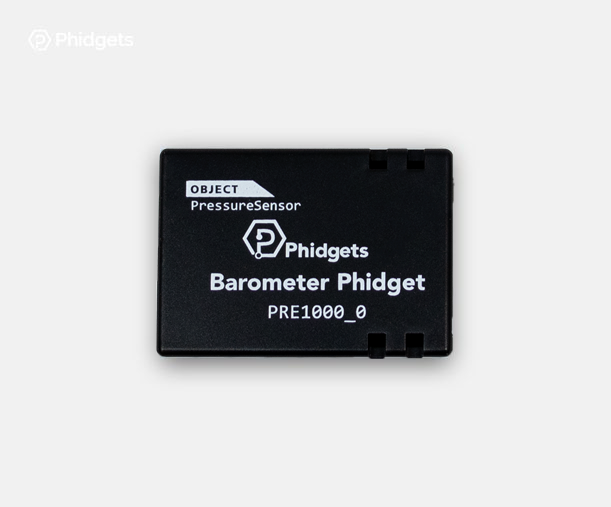
Code (Java)
Create a file called Pressure and insert the following code. Run your code.
Not your programming language? Set my language and IDE.
package pressure;
//Add Phidgets Library
import com.phidget22.*;
public class Pressure {
public static void main(String[] args) throws Exception {
//Create
PressureSensor pressureSensor = new PressureSensor();
//Open
pressureSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Pressure: " + pressureSensor.getPressure() + " kPa");
Thread.sleep(500);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class Pressure {
public static void main(String[] args) throws Exception {
//Create
PressureSensor pressureSensor = new PressureSensor();
//Open
pressureSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Pressure: " + pressureSensor.getPressure() + " kPa");
Thread.sleep(500);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
PressureSensor pressureSensor;
void setup(){
try{
//Create
pressureSensor = new PressureSensor();
//Open
pressureSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Pressure: " + pressureSensor.getPressure() + " kPa");
delay(500);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called Pressure and insert the following code. Run your code.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.PressureSensor import *
import time
#Create
pressureSensor = PressureSensor()
#Open
pressureSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Pressure: " + str(pressureSensor.getPressure()) +" kPa")
time.sleep(0.5)
Code (C#)
Create a file called Pressure and insert the following code. Run your code.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace Pressure
{
class Program
{
static void Main(string[] args)
{
//Create
PressureSensor pressureSensor = new PressureSensor();
//Open
pressureSensor.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("Pressure: " + pressureSensor.Pressure + " kPa");
System.Threading.Thread.Sleep(500);
}
}
}
}
Code (Swift)
Create a file called Pressure and insert the following code. Run your code.
Not your programming language? Set my language and IDE.
You will need to add a Label.
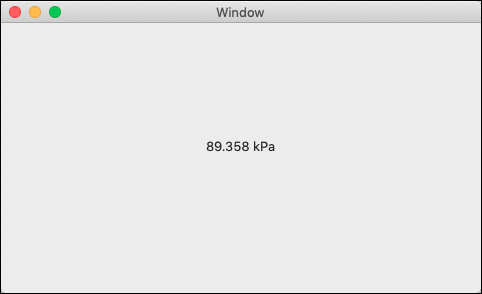
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let pressureSensor = PressureSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = pressureSensor.pressureChange.addHandler(onPressureChange)
//Open
try pressureSensor.open()
}catch{
print(error)
}
}
func onPressureChange(sender: PressureSensor, pressure: Double) {
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.sensorLabel.stringValue = String(pressure) + " kPa"
}
}
}
Applications
Pressure sensors are used in many applications. For example, science labs often use pressure sensors to monitor conditions in experiments and weather forecasting uses barometers to help predict weather events.
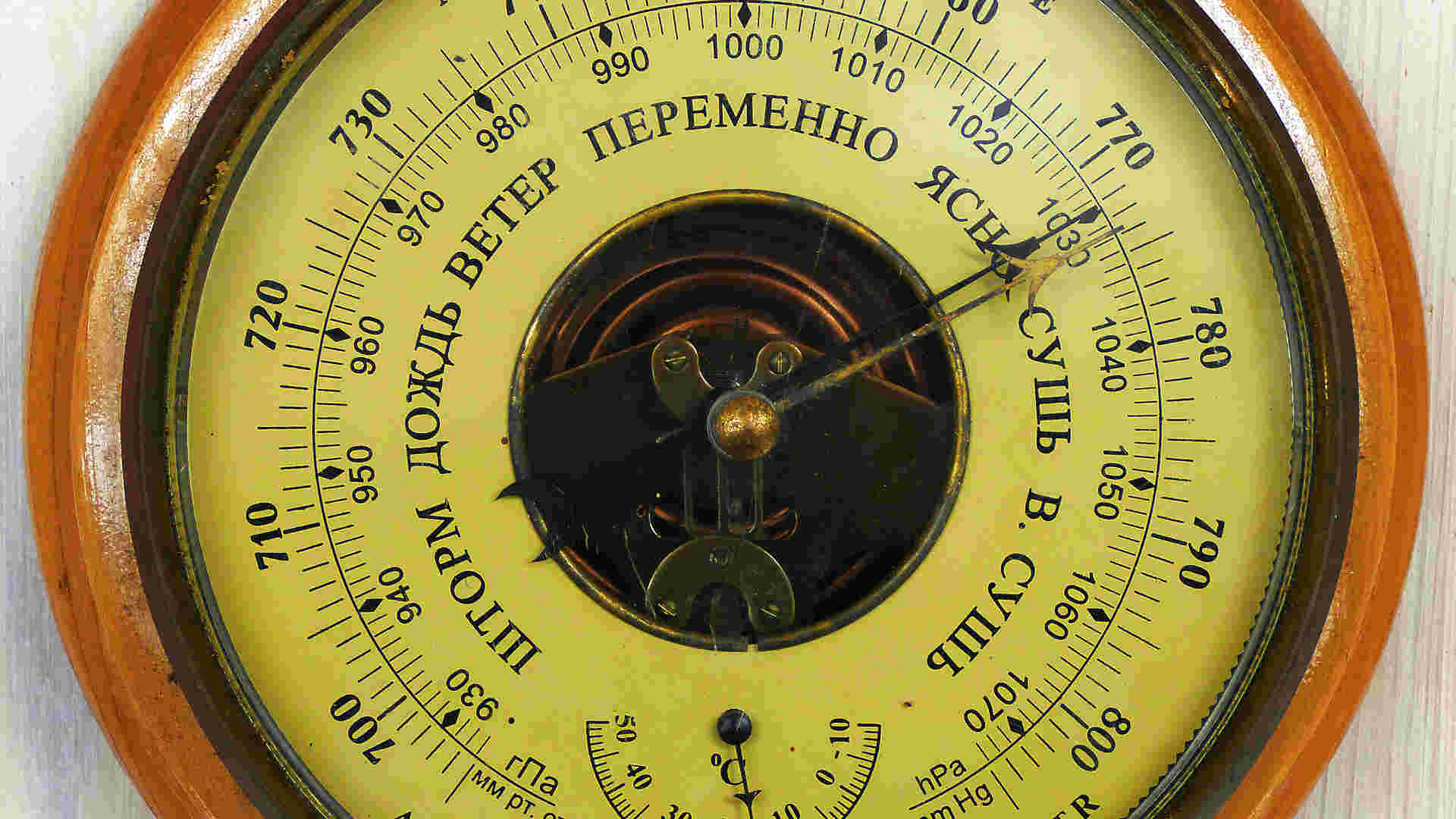
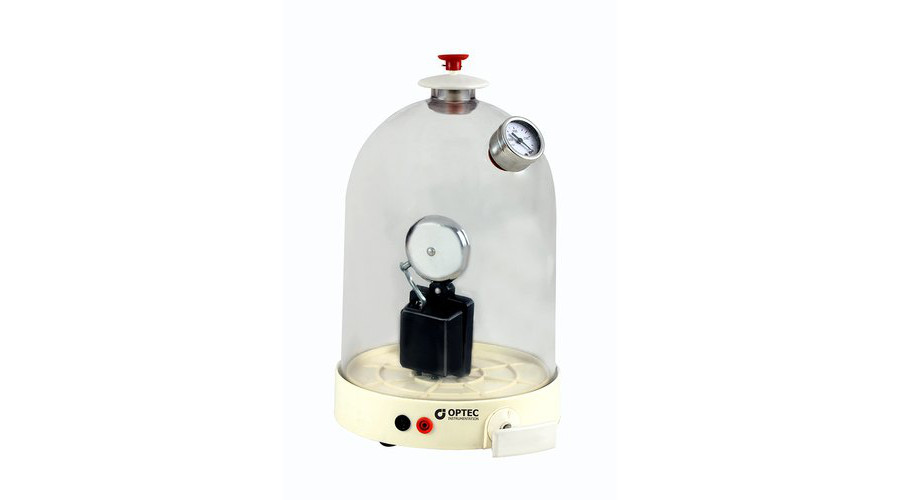
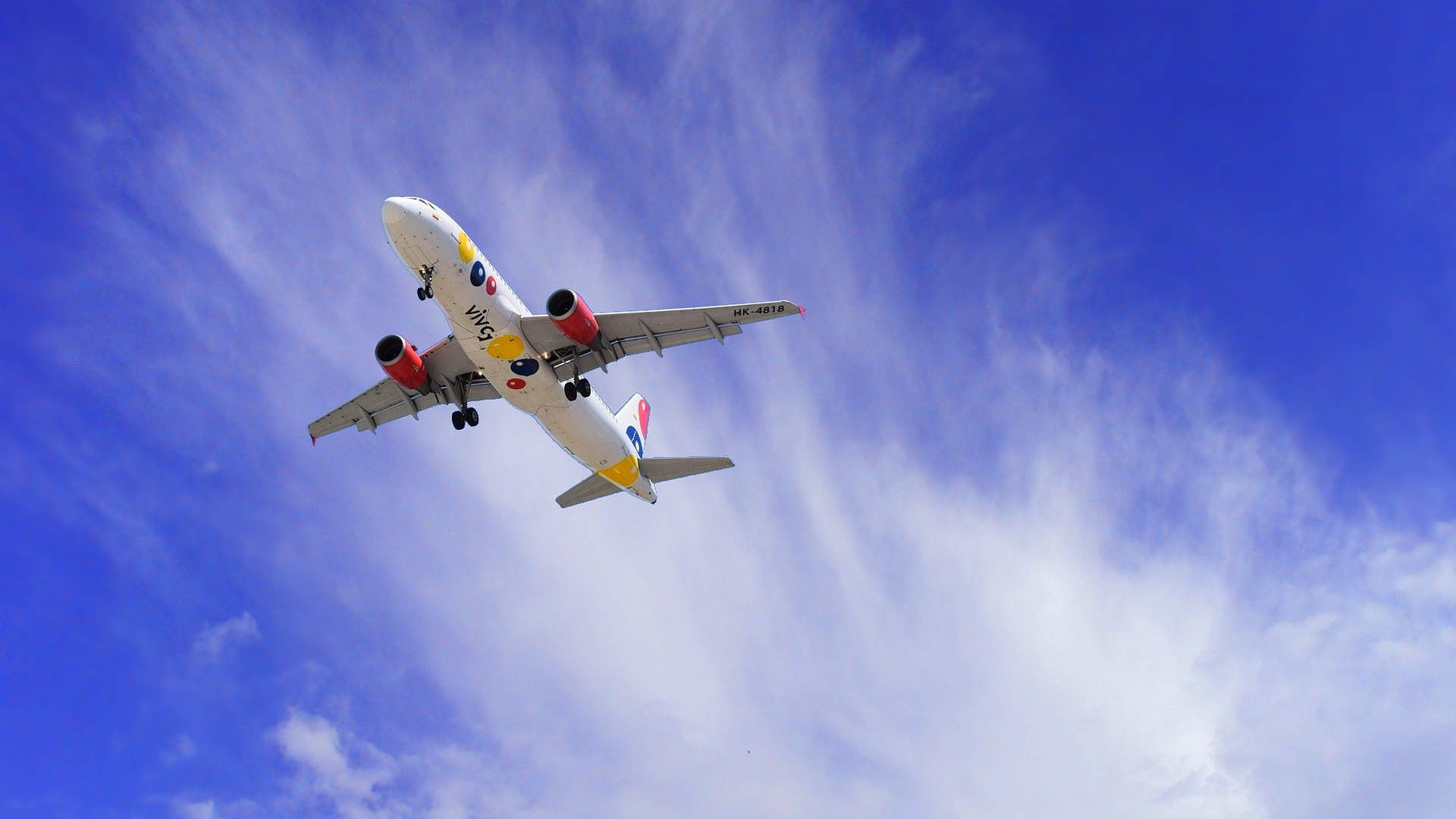
Practice
Write a program using the Barometer Phidget to tell a user their elevation. Use the table below to covert kPa to elevation above sea level.
feet | metres | kPA |
---|---|---|
0 | 0 | 101.3 |
500 | 152 | 99.5 |
1000.0 | 305 | 97.7 |
1500 | 457 | 96.0 |
2000 | 610 | 94.2 |
2500 | 762 | 92.5 |
3000 | 914 | 90.8 |
3500 | 1067 | 89.1 |
4000 | 1219 | 87.5 |
4500 | 1372 | 85.9 |
5000 | 1524 | 84.3 |
6000 | 1829 | 81.2 |
7000 | 2134 | 78.2 |
8000 | 2438 | 75.3 |
9000 | 2743 | 72.4 |
10000 | 3048 | 69.7 |
Check out the advanced lesson Using the Sensor API before you use the API for the first time.