Motion Sensor Phidget
The Motion Sensor Phidget allows you to detect motion up to ten meters away (approximately 30 feet).
This Phidget returns a constant voltage when no objects are detected. When motion is detected, the voltage will increase or decrease.
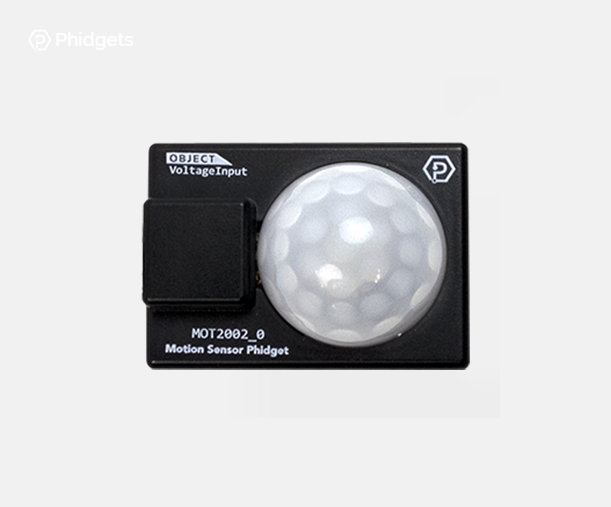
Code (Java)
Create a file called Motion and add the following code. Run your code. Move your hand over the motion sensor to see the value change.
Not your programming language? Set my language and IDE.
package motion;
//Add Phidgets Library
import com.phidget22.*;
public class Motion {
public static void main(String[] args) throws Exception{
//Create
VoltageInput motionSensor = new VoltageInput();
//Address
motionSensor.setHubPort(0);
motionSensor.setIsHubPortDevice(true);
//Open
motionSensor.open(1000);
//Use your Phidget
while (true) {
System.out.println("Motion Value: " + motionSensor.getVoltage());
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class Motion {
public static void main(String[] args) throws Exception{
//Create
VoltageInput motionSensor = new VoltageInput();
//Address
motionSensor.setHubPort(0);
motionSensor.setIsHubPortDevice(true);
//Open
motionSensor.open(1000);
//Use your Phidget
while (true) {
System.out.println("Motion Value: " + motionSensor.getVoltage());
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
VoltageInput motionSensor;
void setup(){
try{
//Create
motionSensor = new VoltageInput();
//Address
motionSensor.setHubPort(0);
motionSensor.setIsHubPortDevice(true);
//Open
motionSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Motion Value: " + motionSensor.getVoltage() + " V");
delay(250);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called Motion and add the following code. Run your code. Move your hand over the motion sensor to see the value change.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.VoltageInput import *
#Required for sleep statement
import time
#Create
motionSensor = VoltageInput()
#Address
motionSensor.setHubPort(0)
motionSensor.setIsHubPortDevice(True)
#Open
motionSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Motion Value: " + str(motionSensor.getVoltage()) + " V")
time.sleep(0.25)
Code (C#)
Create a file called Motion and add the following code. Run your code. Move your hand over the motion sensor to see the value change.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace Motion
{
class Program
{
static void Main(string[] args)
{
//Create
VoltageInput motionSensor= new VoltageInput();
//Address
motionSensor.HubPort = 0;
motionSensor.IsHubPortDevice = true;
//Open
motionSensor.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("Motion Value: " + motionSensor.Voltage);
System.Threading.Thread.Sleep(250);
}
}
}
}
Code (Swift)
Create a file called Motion and add the following code. Run your code. Move your hand over the motion sensor to see the value change.
Not your programming language? Set my language and IDE.
Add two buttons.
Coming Soon!
Applications
The Motion Sensing Phidget uses infrared light to detect motion. Motion sensors commonly used as an integrated component of security systems and lighting control systems.
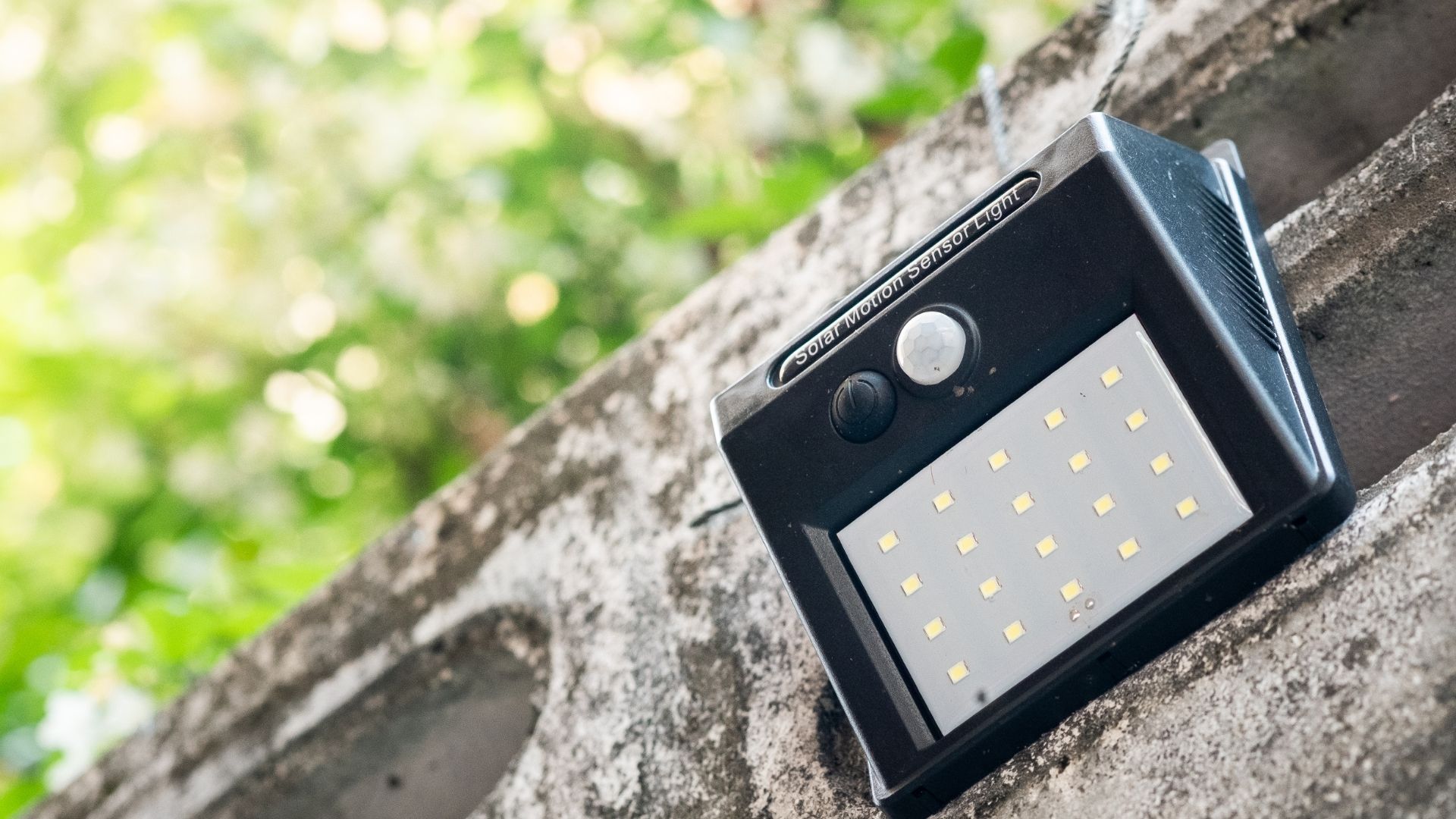
Practice
Use the Motion Sensing Phidget to create a mock security system. If an object is detected, blink the red LED. If there is no movement, the green LED should stay on.
Check out the advanced lesson Using the Sensor API before you use the API for the first time.
API
This device doesn’t have an API of its own. It is controlled by opening a VoltageInput on a VINT Hub.