Language - Visual Basic: Difference between revisions
No edit summary |
|||
Line 90: | Line 90: | ||
Now that you have Mono installed, download and unpack the HelloWorld example for C#: | Now that you have Mono installed, download and unpack the HelloWorld example for C#. This example uses the [[Phidget Manager]] to list all Phidget channels that can be accessed by your computer: | ||
*[{{SERVER}}/downloads/phidget22/examples/dotnet/csharp/Manager/Phidget22_HelloWorld_CSharp_Windows_Ex.zip HelloWorld example] | *[{{SERVER}}/downloads/phidget22/examples/dotnet/csharp/Manager/Phidget22_HelloWorld_CSharp_Windows_Ex.zip HelloWorld example] | ||
Note: The HelloWorld example is compatible with Mono because it does not use Windows Forms. All other C# examples use Windows Forms. | Note: The HelloWorld example is compatible with Mono because it does not use Windows Forms. All other C# examples use Windows Forms. |
Revision as of 16:24, 9 April 2018
Quick Downloads
Documentation
- Phidget22 API (Select C#/VB.Net from drop-down menu)
Example Code
Libraries
Getting Started with VB.NET
Welcome to using Phidgets with VB.NET! By using VB.NET, you will have access to the complete Phidget22 API, including events. We also provide example code in VB.NET for all Phidget devices.
Windows
If you haven't already, please visit the Windows page before you continue reading. There you will be instructed on how to properly set up your Windows machine so you can follow the guides below!
Visual Studio
Use Our Examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. In order to run the examples, you will need to download and install Microsoft Visual Studio.
Now that you have Microsoft Visual Studio installed, select an example that will work with your Phidget:
Open the example project and start the example by pressing the Start button:

The application will open the Phidget, list basic information about the Phidget, and demonstrate the Phidget's functionality. Here is an example of a Digital Output channel on a RFID Phidget:
You should now have the example up and running for your device. Play around with the device and experiment with some of the functionality. When you are ready, the next step is configuring your project and writing your own code!
VINT Hub
If you are trying to connect to one of the VINT hub ports directly rather than to a VINT device connected through a VINT hub then there is one additional step. There is a isHubPort property of the Phidget class that must be set before calling open. It defaults to false but in this case it will need to be set to true. In our examples this is done via command line though in practice you can just set it before calling open. To add command line parameters to your project in Visual Studio, you can go to the Project->Properties screen. Under the Debug section there is a field to enter command line parameters for startup. Use "-h" to make sure isHubPort is set before open is called and then you should be good to go.
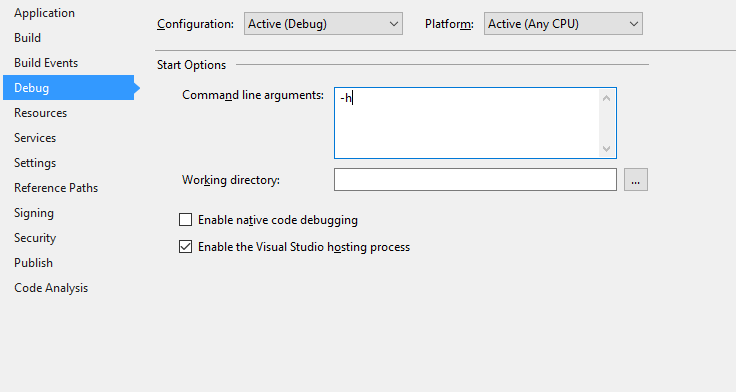
Configure Your Project
When you are building a project from scratch, or adding Phidget functionality to an existing project, you'll need to configure your development environment to properly link the Phidget .NET library. To begin:
Create a new Windows Forms Application project:
Next, right-click to add a reference to the Phidget .NET library:
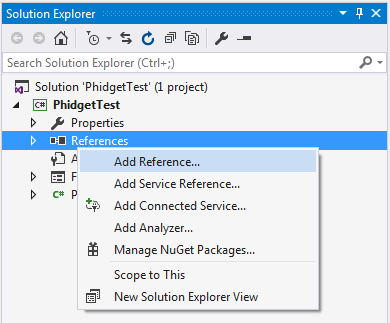
On the following screen, click Browse... and navigate to the location of Phidget22.NET.dll:
- C:\Program Files\Phidgets\Phidget22\Phidget22.NET.dll
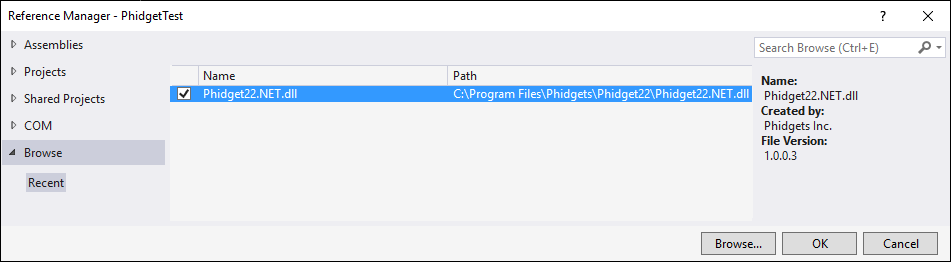
Finally, to include the Phidget .NET library, add the following lines to main window class file:
Imports Phidget22
Imports Phidget22.Events
Success! The project now has access to Phidgets. Next, view the write your own code section located below.
Mono
Use Our Examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. In order to run the examples, you will need to download and install Mono for Windows.
Now that you have Mono installed, download and unpack the HelloWorld example for C#. This example uses the Phidget Manager to list all Phidget channels that can be accessed by your computer:
Note: The HelloWorld example is compatible with Mono because it does not use Windows Forms. All other C# examples use Windows Forms.
Next, copy Phidget22.NET.dll from type the following location:
- C:\Program Files\Phidgets\Phidget22\Phidget22.NET.dll
Place both the HelloWorld example and the Phidget22.NET.dll file in the same location. Your folder should now look something like this:
Open the command prompt at the folder location and enter the following command:
vbnc /r:Phidget22.NET.dll Module1.vb
This will create an executable file called Module1.exe. Type in the following command to run the example:
mono Module1.exe
You should now have the example up and running. When you are ready, the next step is configuring your project and writing your own code!
Configure Your Project
When you are building a project from scratch, or adding Phidget functionality to an exisiting project, you'll need to configure your development environment to properly link the Phidget .NET library.
To include the Phidget .NET library, simply add the following lines to your code:
Imports Phidget22
Imports Phidget22.Events
The project now has access to Phidgets. Next, view the write your own code section located below.
Write Code
By following the instructions for your operating system and compiler above, you now have working examples and a project that is configured. This teaching section will help you understand how the examples were written so you can start writing your own code.
Remember: your main reference for writing VB.NET code will be the Phidget22 API Manual and the example code.
Step One: Initialize and Open
You will need to declare your Phidget object in your code. For example, we can declare a digital input object like this:
ch = New Phidget22.DigitalInput()
Next, we can open the Phidget object like this:
ch.Open()
Although we are not including it on this page, you should include error handling for all Phidget functions. Here is an example of the previous code with error handling:
Try
ch = New Phidget22.DigitalInput()
ch.Open()
Catch ex As PhidgetException
errorBox.addMessage("Error initializing: " + ex.Message)
End Try
Step Two: Wait for Attachment (Plugging In) of the Phidget
Simply calling open does not guarantee you can use the Phidget immediately. To use a Phidget, it must be plugged in (attached). We can handle this by using event driven programming and tracking the attach events. Alternatively, we can modify our code so we wait for an attachment:
ch = New Phidget22.DigitalInput()
ch.Open(5000);
Waiting for attachment will block indefinitely until a connection is made, or until the timeout value is exceeded
To use events to handle attachments, we have to add the following code:
Private Sub device_Attach(ByVal sender As Object, ByVal e As Phidget22.Events.AttachEventArgs) Handles ch.Attach
Console.WriteLine("Phidget Attached!");
End Sub
Step Three: Do Things with the Phidget
We recommend the use of event driven programming when working with Phidgets. In a similar way to handling an attach event as described above, we can also add an event handler for a state change event:
Private Sub device_DigitalInputChange(ByVal sender As Object, ByVal e As Phidget22.Events.DigitalInputStateChangeEventArgs) Handles ch.StateChange
stateText.Text = "State: " + e.State;
End Sub
If events do not suit your needs, you can also poll the device directly for data using code like this:
state= device.State;
stateText.Text = "State: " + e.State;
Step Four: Close and Delete
At the end of your program, be sure to close and delete your device:
device.Close();
Further Reading
Phidget Programming Basics - Here you can find the basic concepts to help you get started with making your own programs that use Phidgets.
Data Interval/Change Trigger - Learn about these two properties that control how much data comes in from your sensors.
Using Multiple Phidgets - It can be difficult to figure out how to use more than one Phidget in your program. This page will guide you through the steps.
Polling vs. Events - Your program can gather data in either a polling-driven or event-driven manner. Learn the difference to determine which is best for your application.
Logging, Exceptions, and Errors - Learn about all the tools you can use to debug your program.
Phidget Network Server - Phidgets can be controlled and communicated with over your network- either wirelessly or over ethernet.