Language - Swift: Difference between revisions
(→iOS) |
|||
Line 79: | Line 79: | ||
Now that your project is created, you need to add the Phidget libraries. In order to do this, you must have CocoaPods installed on your computer (this is covered in detail above in the [[#Use | Now that your project is created, you need to add the Phidget libraries. In order to do this, you must have CocoaPods installed on your computer (this is covered in detail above in the [[#Use Our Examples |use our examples]] section). Open a terminal at the example location and enter the following command: | ||
<syntaxhighlight lang='bash'> | <syntaxhighlight lang='bash'> | ||
pod init | pod init |
Revision as of 23:01, 6 February 2018
Quick Downloads
Already know what you're doing? Here you go:
Documentation
- Phidget22 API (select Swift from the drop-down menu)
Example Code
Libraries
Getting Started with Swift
Welcome to using Phidgets with Swift! By using Swift, you will have access to the complete Phidget22 API, including events. We also provide example code in Swift for multiple Phidget classes.
iOS
If you haven't already, please visit the iOS page before you continue reading. There you will be instructed on how to properly set up your development machine so you can follow the guides below!
Xcode
Use Our Examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. In order to run the examples for iOS you will need to download Xcode from the Mac App Store.
You will also need to install CocoaPods in order to access the Phidget libraries for Swift. You can do this by opening the terminal and entering the following command:
sudo gem install cocoapods
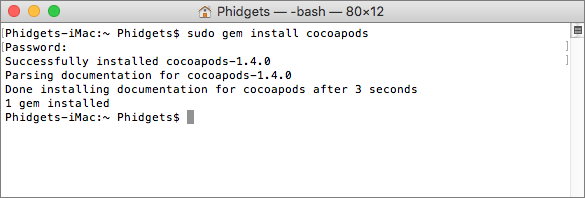
Now that you have Xcode and CocoaPods installed, download a Swift example that will work with your Phidget:
After opening the example, you will notice that there is a file called Podfile
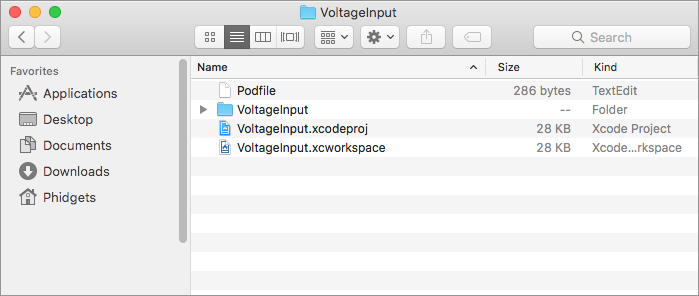
If you open this file, you can see that there is a reference to the Phidget22Swift pod. Note that no version number is included, so the newest available version of the Phidget22Swift pod will be installed:
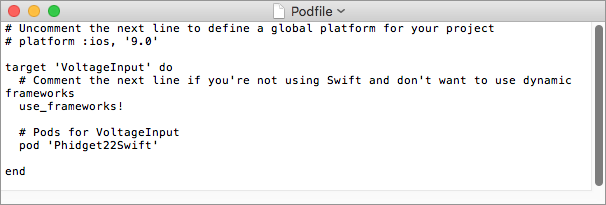
To install the Phidget libraries, open a terminal at the example location and enter the following command:
pod install
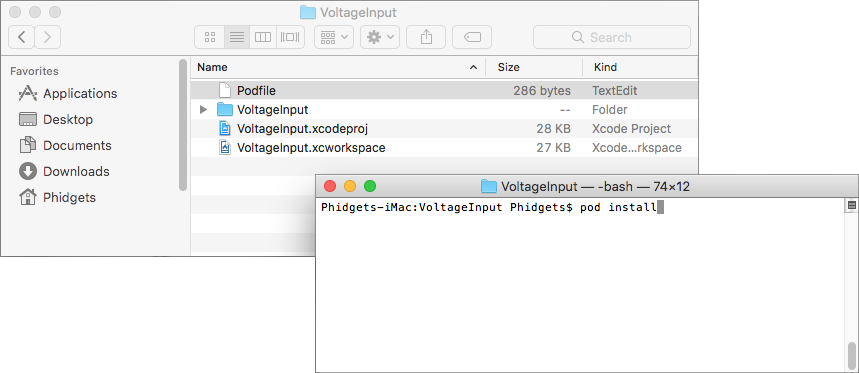
After the libraries are installed, open the generated .xcworkspace file:
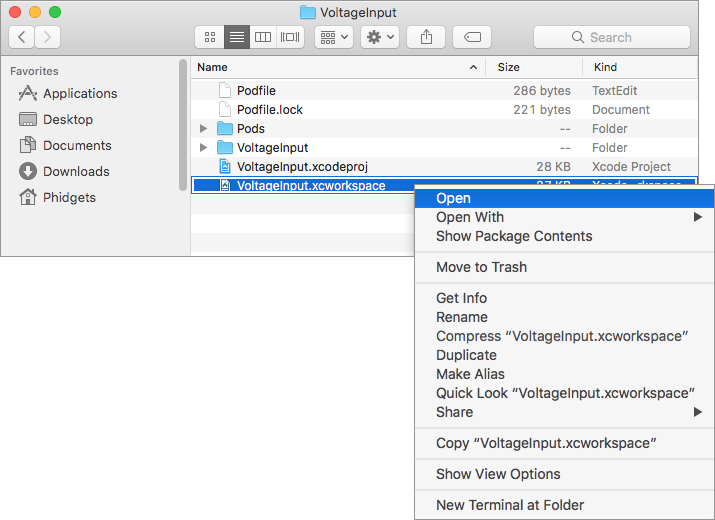
Next simply select the type of device you would like the application to run on and press play:
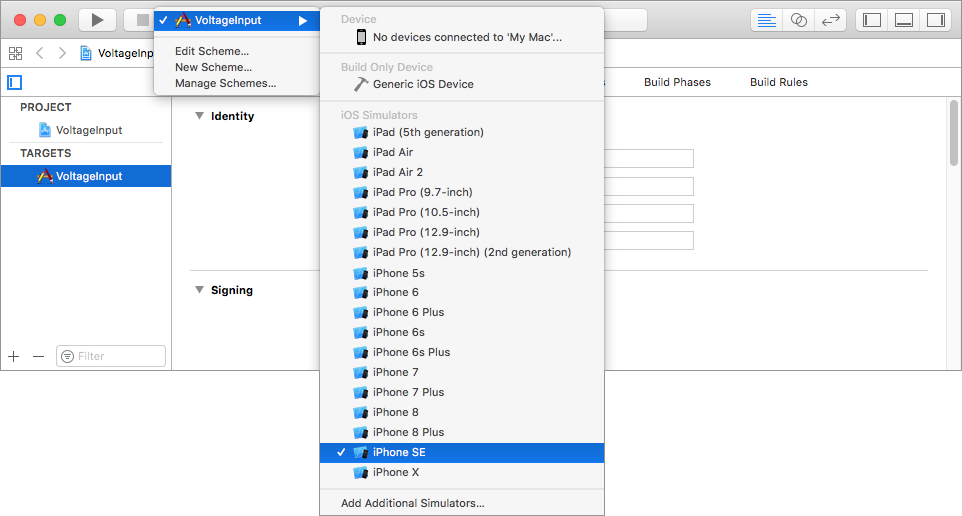
Here is an example output:
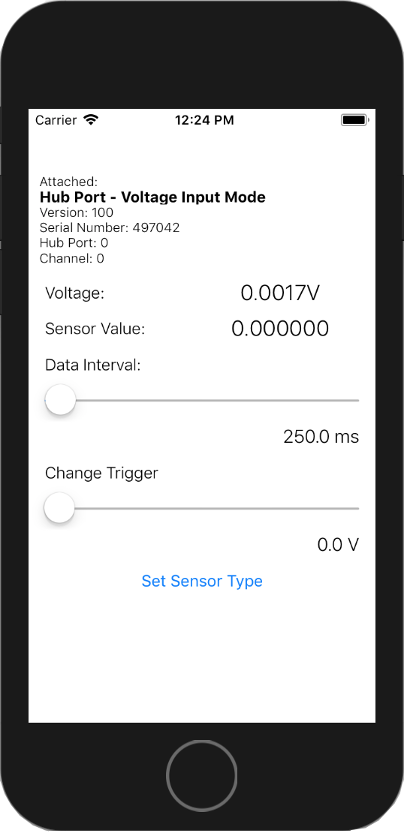
Configure Your Project
Whether you are building a project from scratch, or adding Phidget functionality to an existing project, you will need to configure your development environment to properly link the Phidget libraries. To begin:
Create a new Xcode project:
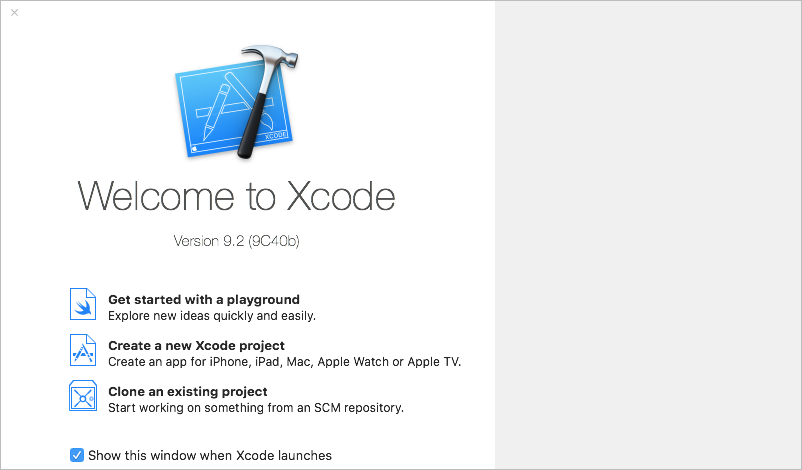
Next, select an iOS application. For this tutorial's purposes, we will use a Single View Application:
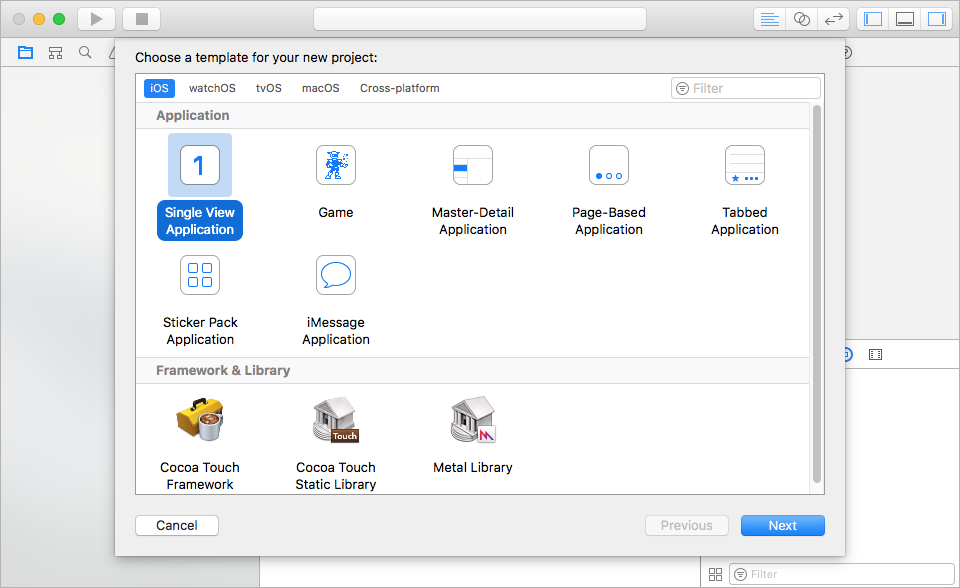
Name the project, select Swift as the language, and choose which devices will be supported:
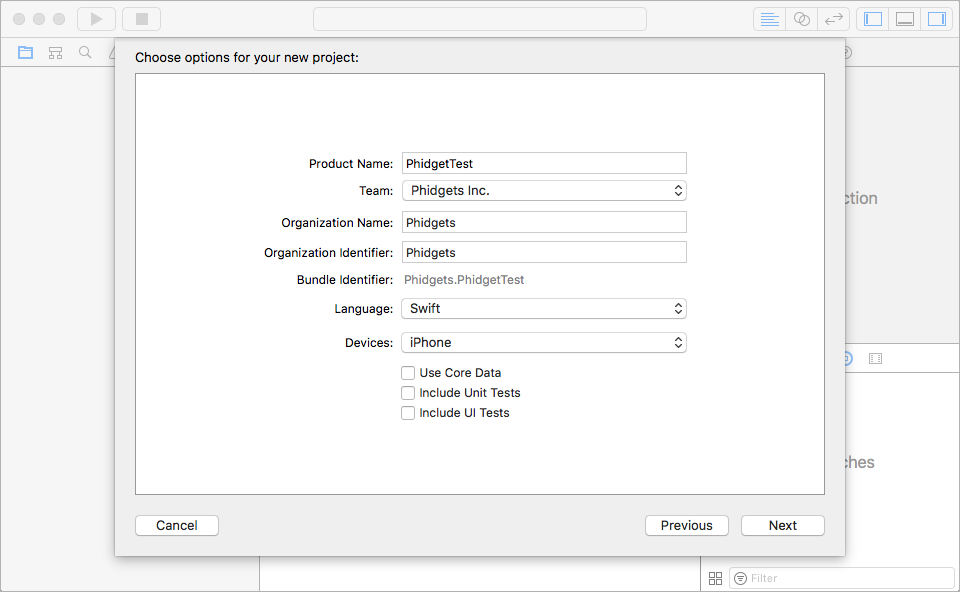
Now that your project is created, you need to add the Phidget libraries. In order to do this, you must have CocoaPods installed on your computer (this is covered in detail above in the use our examples section). Open a terminal at the example location and enter the following command:
pod init
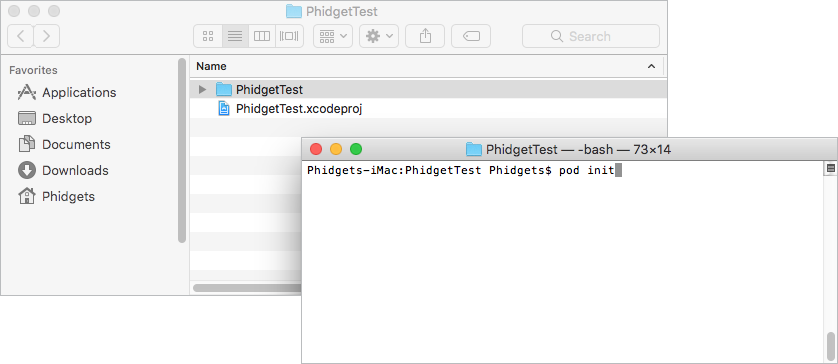
This will create a new Podfile. Open the Podfile in your favorite text editor and add a reference to the Phidget22Swift pod:
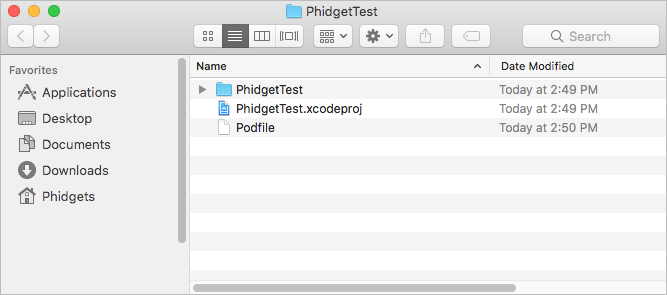
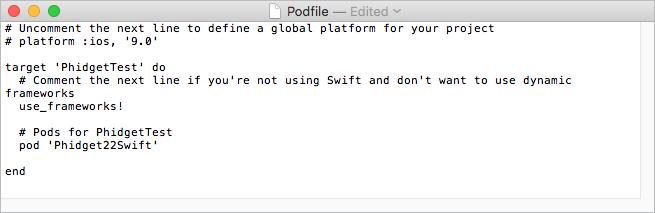
Save your edit to the Podfile, and then enter the following command in the terminal which was opened at the example location:
pod install
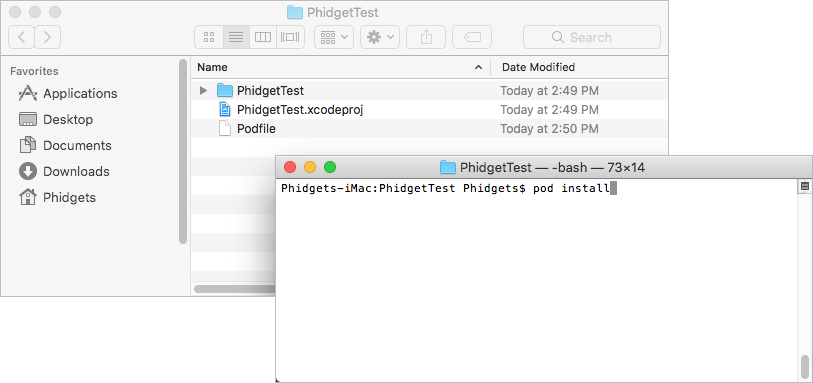
After running the command, open the xcworkspace file and access the Phidget libraries by adding the following line to the top of your files:
import Phidget22Swift
Success! The project now has access to Phidgets and we are ready to begin coding.
Write Code
By following the instructions for your operating system and compiler above, you now have working examples and a project that is configured. This teaching section will help you understand how the examples were written so you can start writing your own code.
Remember: your main reference for writing Swift code will be the Phidget22 API Manual and the example code.
Step One: Initialize and Open
You will need to declare your Phidget object in your code. For example, we can declare a digital input object like this:
let ch = DigitalInput()
Next, the Phidget object needs to be opened:
ch.open()
Although we are not including it on this page, you need to include error handling for all Phidget functions. Here is an example of the previous code with error handling:
do{
try ch.open
}catch let error as PhidgetError{
//handle error
}
Step Two: Wait for Attachment (Plugging In) of the Phidget
Simply calling open does not guarantee you can use the Phidget immediately. To use a Phidget, it must be plugged in (attached). We can handle this by using event driven programming and tracking the attach events. Alternatively, we can modify our code so we wait for an attachment:
ch.open(timeout: 5000)
Waiting for attachment will block indefinitely until a connection is made, or until the timeout value is exceeded.
To use events, we have to modify our code slightly:
ch.attach.addHandler(attach_handler)
Phidget_open(ch)
Next, we have to declare the function that will be called when an attach event is fired - in this case the function attach_handler will be called.
func attach_handler(sender: Phidget){
let attachedDevice = sender as! DigitalInput
//configure device here
}
Step Three: Do Things with the Phidget
We recommend the use of event driven programming when working with Phidgets. In a similar way to handling an attach event as described above, we can also add an event handler for a state change event:
ch.attach.addHandler(attach_handler)
ch.stateChange.addhandler(stateChange_handler)
ch.open()
This code will connect a function and an event. In this case, the stateChange_handler function will be called when there has been a change to the devices input. Next, we need to create the stateChange_handler function:
func stateChange_handler(sender: DigitalInput, state: Bool){
if(state){
//state is true
}
else{
//State is false
}
}
If events do not suit your needs, you can also poll the device directly for data using code like this:
var state = ch.getState()
stateLabel.text = state ? "True" : "False"
Step Four: Close
At the end of your program, be sure to close your device.
ch.close()
Further Reading
Phidget Programming Basics - Here you can find the basic concepts to help you get started with making your own programs that use Phidgets.
Data Interval/Change Trigger - Learn about these two properties that control how much data comes in from your sensors.
Using Multiple Phidgets - It can be difficult to figure out how to use more than one Phidget in your program. This page will guide you through the steps.
Polling vs. Events - Your program can gather data in either a polling-driven or event-driven manner. Learn the difference to determine which is best for your application.
Logging, Exceptions, and Errors - Learn about all the tools you can use to debug your program.
Phidget Network Server - Phidgets can be controlled and communicated with over your network- either wirelessly or over ethernet.