Language - Python Windows LiClipse: Difference between revisions
No edit summary |
|||
Line 47: | Line 47: | ||
When you are building a project from scratch, or adding Phidget functionality to an existing project, you'll need to configure your development environment to properly link the Phidget Python library. | When you are building a project from scratch, or adding Phidget functionality to an existing project, you'll need to configure your development environment to properly link the Phidget Python library. | ||
To start, | To start, create a new Python project: | ||
[[Image:Python_liclipse_newproject.PNG|link=|center]] | |||
Next, add a new file to the project: | |||
[[Image:Python_liclipse_newfile.PNG|link=|center]] | |||
To include the Phidget Python library, add the following imports to your code: | To include the Phidget Python library, add the following imports to your code: |
Revision as of 20:26, 20 August 2018
Template:Language - Python Dev Environment Table
Language - Python Windows with LiClipse Welcome to using Phidgets with Python! By using Python, you will have access to the complete Phidget22 API, including events. LiClipse is a closed-source development environment based on Eclipse, with support for Python. |
Install Phidget Drivers for Windows
Before getting started with the guides below, ensure you have the following components installed on your machine:
- You will need the Phidgets Windows Drivers
- You will need a version of Python installed on your machine (2.7 and 3.6+ are compatible with Phidgets).
The recommended way to install the Phidget22 Python module is using the PIP package manager.
Python versions 2.7.9+ and 3.4+ include PIP by default.
To install the Phidget22 Python module with PIP, simply open the Command Prompt (press the Windows key and search for "cmd"), and enter the command:
python -m pip install Phidget22
To install the Phidget22 libraries to a specific Python version, you can use the Python Windows Launcher from the Command Prompt as follows (replace -X.X with your Python version, e.g. -2.7 or -3.6):
py -X.X -m pip install Phidget22
After unpacking the Phidget22 Python module, open the Command Prompt (press the Windows key and search for "cmd") Locate the folder where you downloaded the Python module and enter the following command:
python setup.py install
This will build the module and install the Python module files into your site-packages directory.
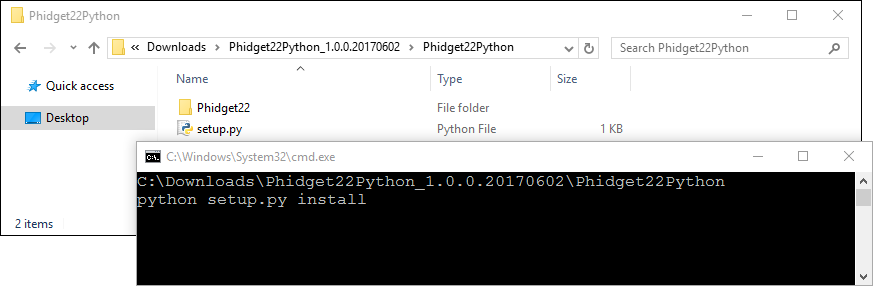
Use our examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. Before we get started, make sure you have read how to install the Phidget Python module section above. You will also need to download LiClipse if you have not already.
Now that you have Python and the Phidget Python module installed, as well as LiClipse, select an example that will work with your Phidget:
Next, create a new Python project:
Add the example you just downloaded by dragging it into the project:
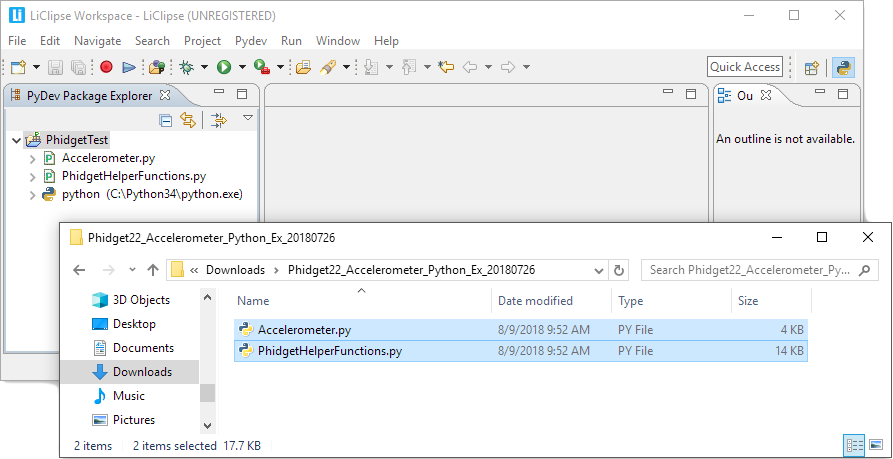
Add PhidgetHelperFunctions.py to the project by dragging it into the project.
Finally, run the project:
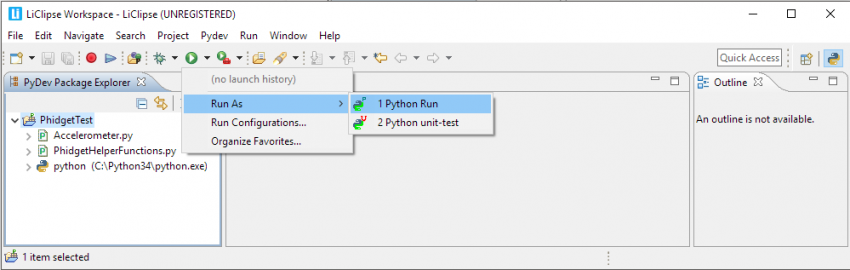
If prompted, select the example file to be run:
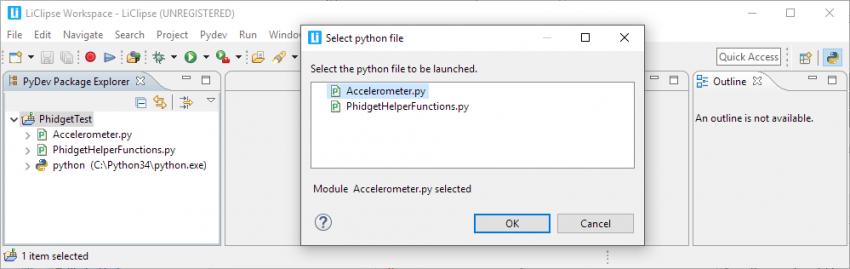
You should now have the example up and running for your device. Your next step is to look at the Editing the Examples section below for information about the example and important concepts for programming Phidgets. This would be a good time to play around with the device and experiment with some of its functionality.
Editing the Examples
To get our example code to run in a custom application, simply remove the calls to AskForDeviceParameters and PrintEventDescriptions, and hard-code the addressing parameters for your application.
If you are unsure what values to use for the addressing parameters, check the Finding The Addressing Information page.
For instance:
channelInfo = AskForDeviceParameters(ch)
ch.setDeviceSerialNumber(channelInfo.deviceSerialNumber)
ch.setHubPort(channelInfo.hubPort)
ch.setIsHubPortDevice(channelInfo.isHubPortDevice)
ch.setChannel(channelInfo.channel)
if(channelInfo.netInfo.isRemote):
ch.setIsRemote(channelInfo.netInfo.isRemote)
if(channelInfo.netInfo.serverDiscovery):
Net.enableServerDiscovery(PhidgetServerType.PHIDGETSERVER_DEVICEREMOTE)
else:
Net.addServer("Server", channelInfo.netInfo.hostname,
channelInfo.netInfo.port, channelInfo.netInfo.password, 0)
Might become:
ch.setDeviceSerialNumber(370114)
ch.setHubPort(2)
ch.setIsHubPortDevice(1)
Notice that you can leave out any parameter not relevant to your application for simplicity.
You can then manipulate the rest of the code as your application requires. A more in-depth description of programming with Phidgets can be found in our guide on Phidget Programming Basics.
Write Code
By following the instructions for your operating system and compiler above, you now have working examples and a project that is configured. This teaching section will help you understand how the examples were written so you can start writing your own code.
Remember: your main reference for writing Python code will be the Phidget22 API Manual and the example code.
Step One: Create and Address
You will need to declare your Phidget object in your code. For example, we can declare a digital input object like this:
ch = DigitalInput()
Next, we can address which Phidget we want to connect to by setting parameters such as DeviceSerialNumber.
ch.setDeviceSerialNumber(496911)
Although we are not including it on this page, you should handle exceptions of all Phidget functions. Here is an example of the previous code with error handling:
try:
ch = DigitalInput()
except RuntimeError as e:
print("Runtime Error: %s" % e.message)
try:
ch.setDeviceSerialNumber(496911)
except PhidgetException as e:
print (“Phidget Exception %i: %s” % (e.code, e.details))
Step Two: Open and Wait for Attachment
After we have specified which Phidget to connect to, we can open the Phidget object like this:
ch.openWaitForAttachment(5000)
To use a Phidget, it must be plugged in (attached). We can handle this by calling openWaitForAttachment, which will block indefinitely until a connection is made, or until the timeout value is exceeded. Simply calling open does not guarantee you can use the Phidget immediately.
Alternately, you could verify the device is attached by using event driven programming and tracking the attach events.
To use events to handle attachments, we have to modify our code slightly:
ch = DigitalInput()
ch.setOnAttachHandler(onAttachHandler)
ch.openWaitForAttachment(5000)
Next, we have to declare the function that will be called when an attach event is fired - in this case the function onAttachHandler will be called:
def onAttachHandler(e):
print("Phidget attached!")
We recommend using this attach handler to set any initialization parameters for the channel such as DataInterval and ChangeTrigger from within the AttachHandler, so the parameters are set as soon as the device becomes available.
Step Three: Do Things with the Phidget
We recommend the use of event driven programming when working with Phidgets. In a similar way to handling an attach event as described above, we can also add an event handler for a state change event:
ch = DigitalInput()
ch.setOnAttachHandler(onAttachHandler)
ch.setOnStateChangeHandler(onStateChangeHandler)
ch.openWaitForAttachment(5000)
This code will connect a function to an event. In this case, the onStateChangeHandler function will be called when there has been a change to the channel's input. Next, we need to create the onStateChangeHandler function:
def onStateChangeHandler(self, state):
print("State %f" % state)
return 0
If you are using multiple Phidgets in your program, check out our page on Using Multiple Phidgets for information on how to properly address them and use them in events.
If events do not suit your needs, you can also poll the device directly for data using code like this:
state = ch.getState()
print("State %f" % state)
Important Note: There will be a period of time between the attachment of a Phidget sensor and the availability of the first data from the device. Any attempts to get this data before it is ready will result in an exception. See more information on this on our page for Unknown Values.
Enumerations
Some Phidget devices have functions that deal with specific predefined values called enumerations. Enumerations commonly provide readable names to a set of numbered options.
Enumerations with Phidgets in Python will take the form of EnumerationType.ENUMERATION_NAME.
For example, specifying a SensorType to use the 1142 for a voltage input would look like:
VoltageSensorType.SENSOR_TYPE_1142
and specifying a K-Type thermocouple for a temperature sensor would be:
ThermocoupleType.THERMOCOUPLE_TYPE_K
The Phidget error code for timing out could be specified as:
ErrorCode.EPHIDGET_TIMEOUT
You can find the Enumeration Type under the Enumerations section of the Phidget22 API for your device, and the Enumeration Name in the drop-down list within.
Step Four: Close and Delete
At the end of your program, be sure to close and delete your device:
ch.close()
Setting Up a New Project
When you are building a project from scratch, or adding Phidget functionality to an existing project, you'll need to configure your development environment to properly link the Phidget Python library.
To start, create a new Python project:
Next, add a new file to the project:
To include the Phidget Python library, add the following imports to your code:
from Phidget22.PhidgetException import *
from Phidget22.Phidget import *
Then, you will also have to import the class for your particular Phidget. For example, you would include the following line for a DigitalInput:
from Phidget22.Devices.DigitalInput import *
The project now has access to Phidgets.
Further Reading
Phidget Programming Basics - Here you can find the basic concepts to help you get started with making your own programs that use Phidgets.
Data Interval/Change Trigger - Learn about these two properties that control how much data comes in from your sensors.
Using Multiple Phidgets - It can be difficult to figure out how to use more than one Phidget in your program. This page will guide you through the steps.
Polling vs. Events - Your program can gather data in either a polling-driven or event-driven manner. Learn the difference to determine which is best for your application.
Logging, Exceptions, and Errors - Learn about all the tools you can use to debug your program.
Phidget Network Server - Phidgets can be controlled and communicated with over your network- either wirelessly or over ethernet.