Language - LabVIEW
Quick Downloads
Documentation
- Documentation is included in the LabVIEW examples in the form of vi trees. See the next section for details.
Example Code
Libraries
Getting Started with LabVIEW
Welcome to using Phidgets with LabVIEW! By using LabVIEW, you will have access to the complete Phidget22 API, including events. We also provide example code in LabVIEW for all Phidget devices.
Windows
If you haven't already, please visit the Windows page before you continue reading. There you will be instructed on how to properly set up your Windows machine so you can follow the guides below!
Use our examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. In order to run the examples, you will need to download and install the LabVIEW from National Instruments.
Next, download and unpack the Phidgets LabVIEW library:
Rename the unpacked folder to Phidgets
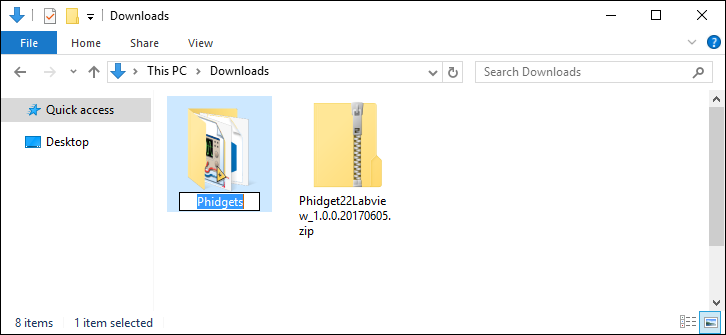
Navigate to the following directory:
- For 32-bit LabVIEW -> C:/Program Files (x86)/National Instruments/LabVIEW 20xx/instr.lib
- For 64-bit LabVIEW -> C:/Program Files/National Instruments/LabVIEW 20xx/instr.lib
Place the renamed folder at this location:
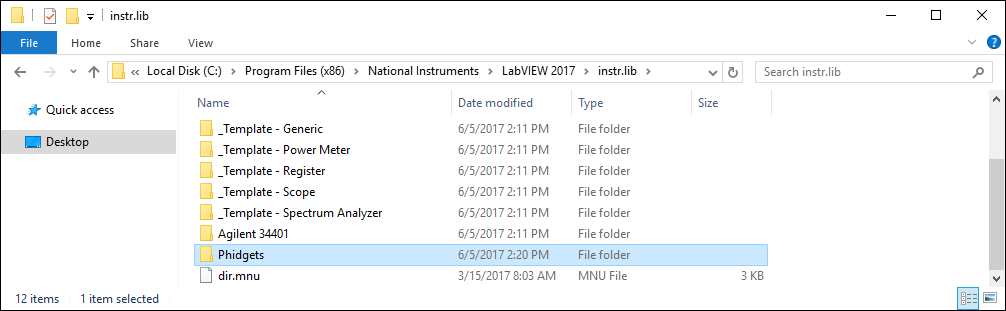
Next, open LabVIEW and create a new VI:
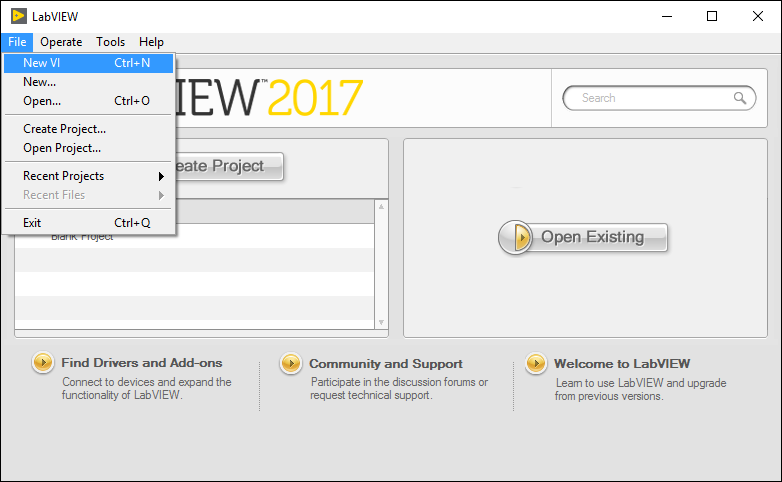
Navigate to the block diagram window that was generated and open the functions palette (View -> Functions Palette). Next, navigate to the Phidgets palette (Instrument I/O -> Instrument Drivers -> Phidgets):
Next, select a palette that will work for your Phidget and drag the VI Tree.vi onto your block diagram:
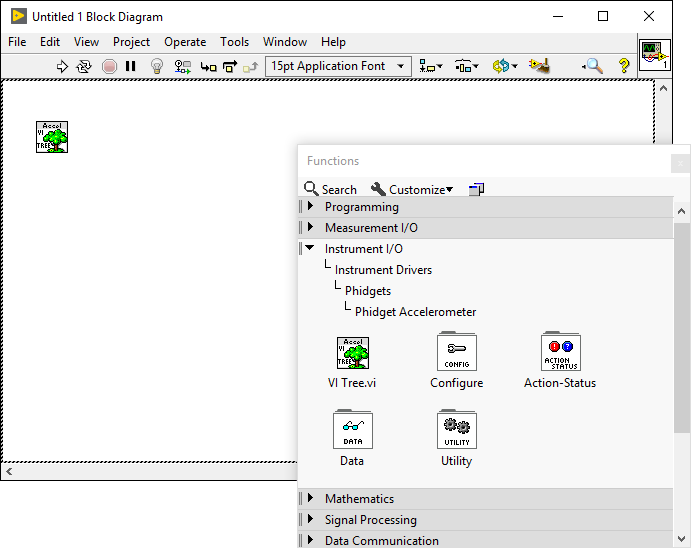
Right-click on VI Tree.vi and select Open Front Panel:
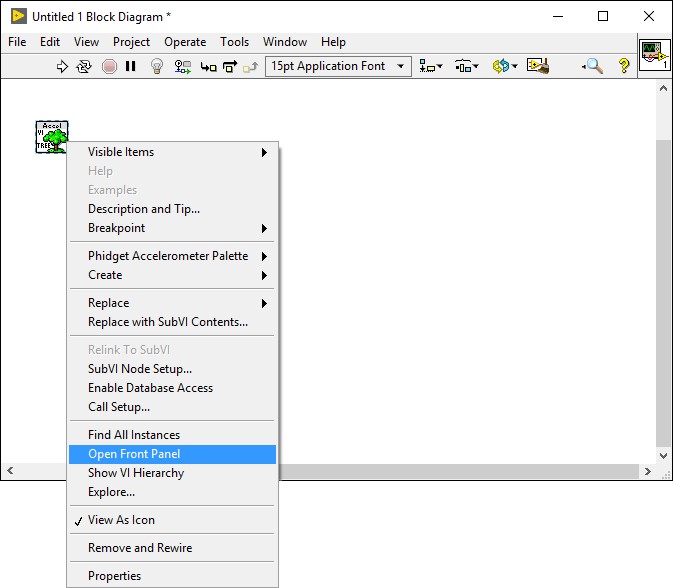
From the front panel, navigate to the block diagram (Window -> Block Diagram):
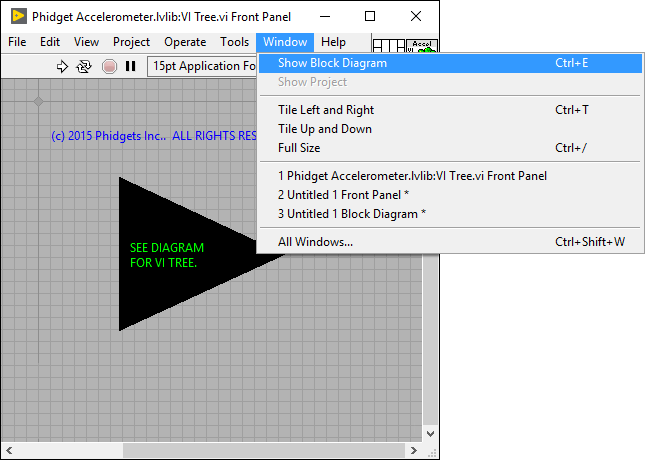
The examples are located near the bottom of the block diagram. Right-click the example you would like to use and select Open Front Panel:
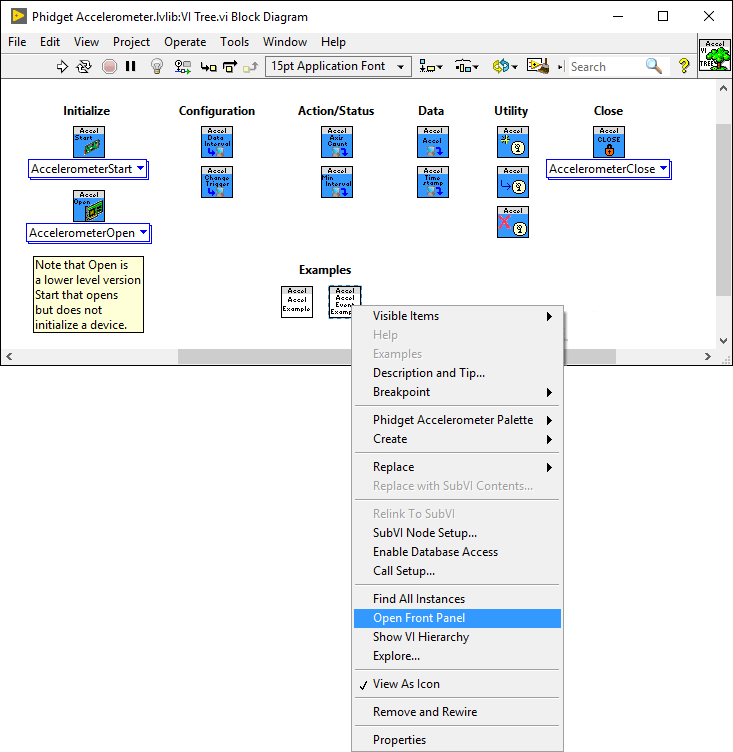
When you are ready, press run and the application will demonstrate the Phidget's functionality. Here is an example of an Accelerometer channel on a Spatial Phidget:
You should now have the example up and running for your device. Play around with the device and experiment with some of the functionality. When you are ready, the next step is configuring your environment and writing your own code!
Configure your environment
If you haven't already, jump back and take a look at the use our examples section above. There you will be instructed on how to properly set up LabVIEW so you can follow the guides below. If you are ready, keep reading.
To begin configuring your environment, we recommend enabling the Show constant labels setting in LabVIEW . This setting will reduce complexity when developing, and is especially recommended for beginners. To enable the setting, first navigate to Tools -> Options on your block diagram:
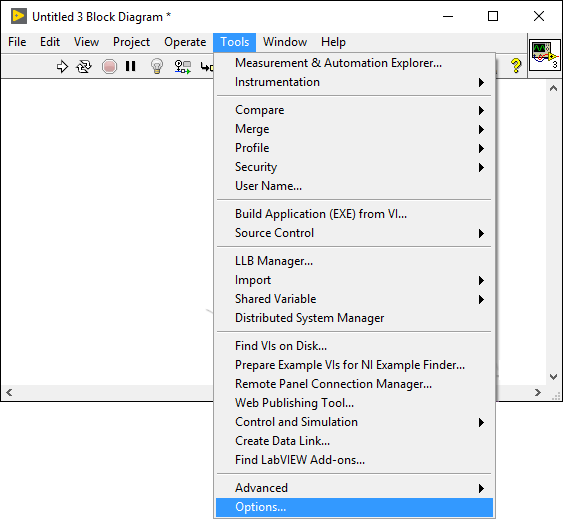
Select the Environment category and enable Show created constant labels located at the bottom of the screen:
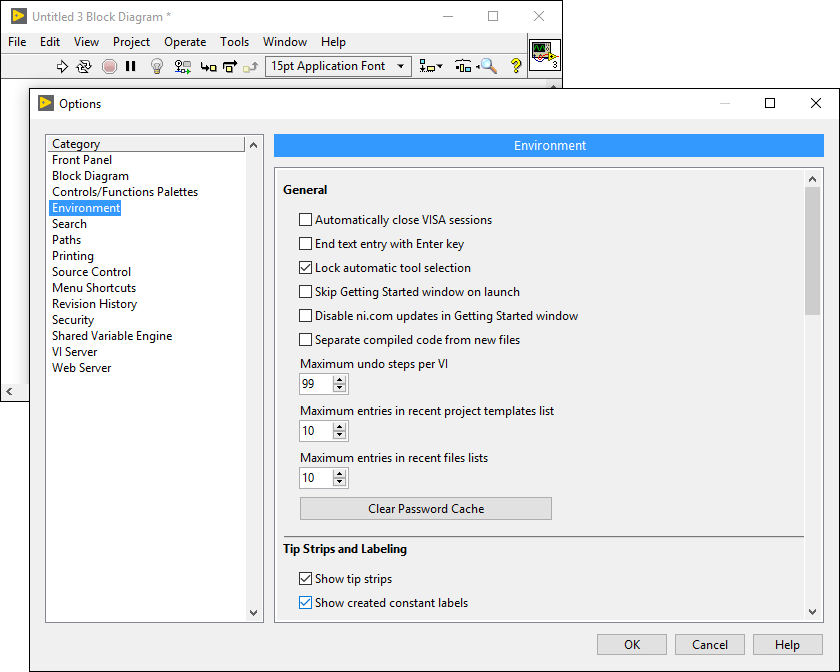
To begin working with Phidgets, you will need both a StartPhidget VI and a ClosePhidget VI:
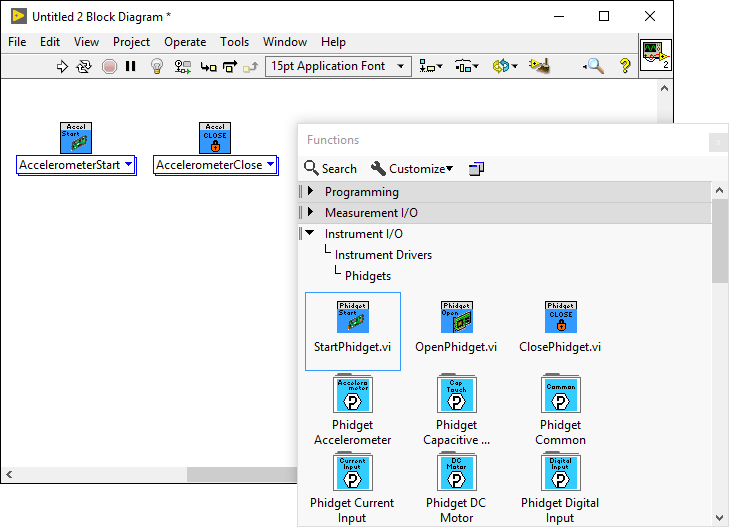
Select a class that will work with your Phidget from the drop-down menu:
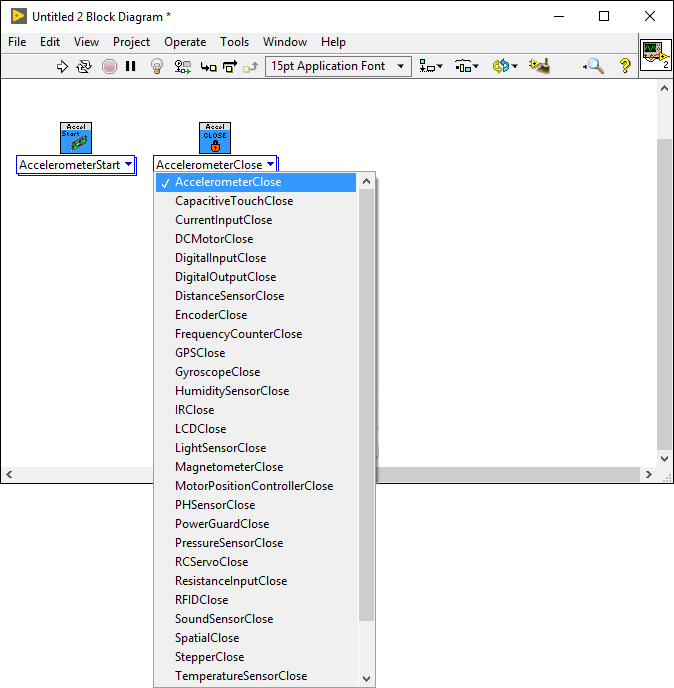
You can now add device information or any initialization parameters to the StartPhidget VI. Using your mouse, hover over the connections to see information about it:
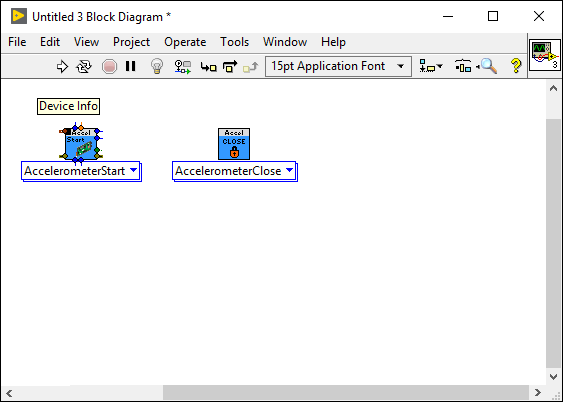
If you would like to add device information or initialization parameters, right-click the connection and navigate to one of the following:
- Create -> Constant
- Create -> Control
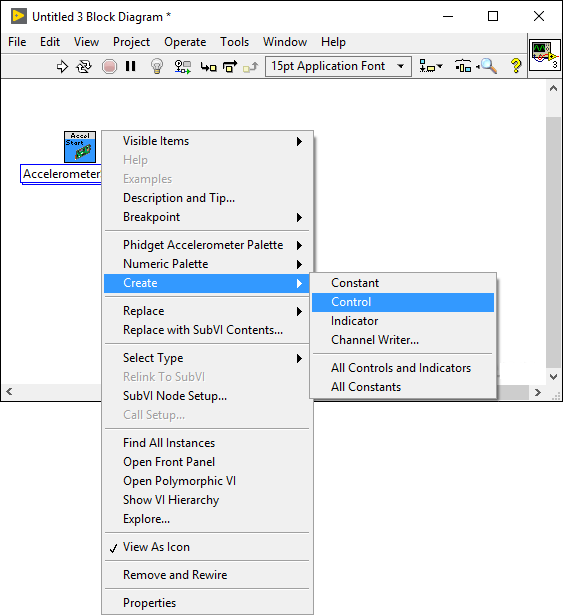
Creating a constant will allow you to modify device information from the block diagram:
Creating a control will allow you to modify device information from the front panel:
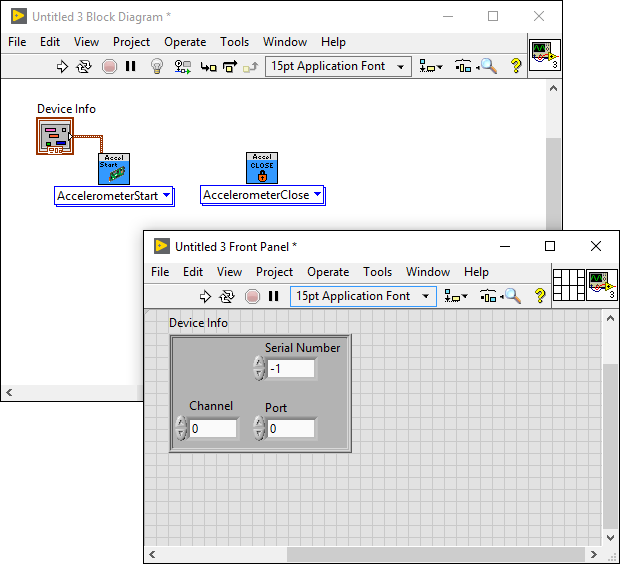
The environment now has access to Phidgets. Next, view the write your own code section located below.
Write Code
By following the instructions for your operating system and compiler above, you probably now have a working example and want to understand it better so you can change it to do what you want. This teaching section has resources for you to learn from the examples and write your own.
Your main reference for writing LabVIEW code will be this page, the examples, the Phidget22 API, and the VI help files.
Examples of more complex general topics such as using multiple Phidgets and connecting to a Phidget over the Network Server can be found under the VI Tree for the Phidget Common palette.
Example Flow
Most LabVIEW examples follow the same basic flow: starting a Phidget, reading some data, and closing the Phidget.
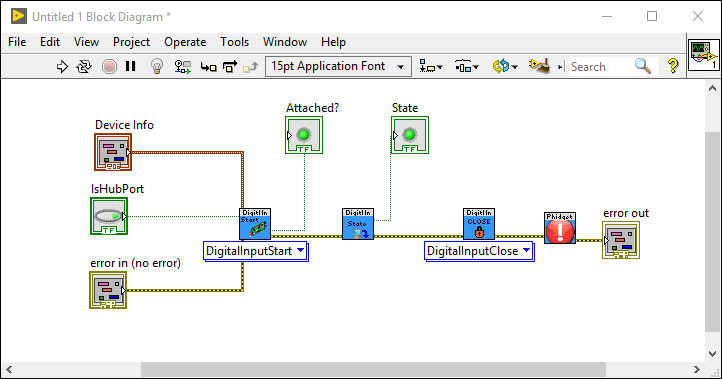
Step One: Initialize, Open and Wait for Attachment of the Phidget
The entire process of opening and initializing a Phidget can be done by using the version of StartPhidget.vi that corresponds to your device. In most cases, StartPhidget.vi will also attempt to wait for the first data to become available from the device for 5 seconds after initialization.
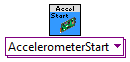
If you'd prefer to initialize the device manually, you can use OpenPhidget.vi for your device and call the individual functions to set up the device.
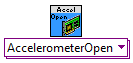
Step Two: Do Things with the Phidget
You can read data and interact with your Phidget both by polling it for its current state (or to set a state), or by catching events that trigger when the data changes.
To poll devices, simply place the corresponding blocks:
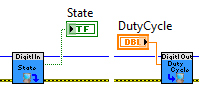
To use events, there are three main blocks for each type, to create, execute, and close the event handler. When creating the event, all devices using an event of the same type must be grouped into an array to ensure the events get processed correctly.
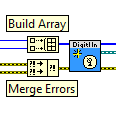
Once created, the events will be processed by [Name]EventExe.vi. When an event occurs, the pertinent information will be output, as well as information to reference which device caused it.
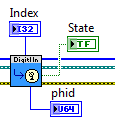
After a program has run its course, the event handler must be closed.

Step Three: Close and Delete
Closing a Phidget is done by using the appropriate version of ClosePhidget.vi
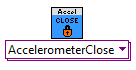
Further Reading
Phidget Programming Basics - Here you can find the basic concepts to help you get started with making your own programs that use Phidgets.
Data Interval/Change Trigger - Learn about these two properties that control how much data comes in from your sensors.
Using Multiple Phidgets - It can be difficult to figure out how to use more than one Phidget in your program. This page will guide you through the steps.
Polling vs. Events - Your program can gather data in either a polling-driven or event-driven manner. Learn the difference to determine which is best for your application.
Logging, Exceptions, and Errors - Learn about all the tools you can use to debug your program.
Phidget Network Server - Phidgets can be controlled and communicated with over your network- either wirelessly or over ethernet.