Language - C
Quick Downloads
Documentation
- Phidget22 API (select C from the drop-down menu)
Example Code
Libraries
- Windows Drivers Installer (32-Bit)
- Windows Drivers Installer (64-Bit)
- Windows Development Libraries
- macOS Installer
- macOS Standalone Control Panel
- Linux Libraries
- Linux Phidget Network Server
Getting started with C/C++
Welcome to using Phidgets with C/C++! By using C/C++, you will have access to the complete Phidget22 API, including events. We also provide example code in C/C++ for all Phidget devices.
If you are developing for Windows, keep reading. Otherwise, select your operating system to jump ahead:
Windows
If you haven't already, please visit the Windows page before you continue reading. There you will be instructed on how to properly set up your Windows machine so you can follow the guides below!
Visual Studio
Use our examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. In order to run the examples, you will need to download and install Microsoft Visual Studio.
Now that you have Microsoft Visual Studio installed, select an example that will work with your Phidget:
Open the example project and start the example by pressing the Local Windows Debugger button:
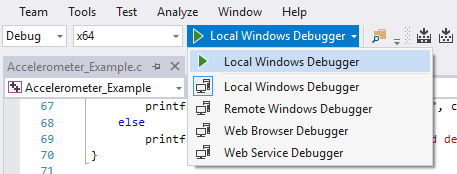
The application will open the Phidget, list basic information about the Phidget, and demonstrate the Phidget's functionality. Here is an example of an Accelerometer channel on a Spatial Phidget:
You should now have the example up and running for your device. Play around with the device and experiment with some of the functionality. When you are ready, the next step is configuring your project and writing your own code!
Configure your project
When you are building a project from scratch, or adding Phidget functionality to an existing project, you'll need to configure your development environment to properly link the Phidget C/C++ library. To begin:
Create a new Win32 Console application:
After creating a project with the default settings, access the project's properties:
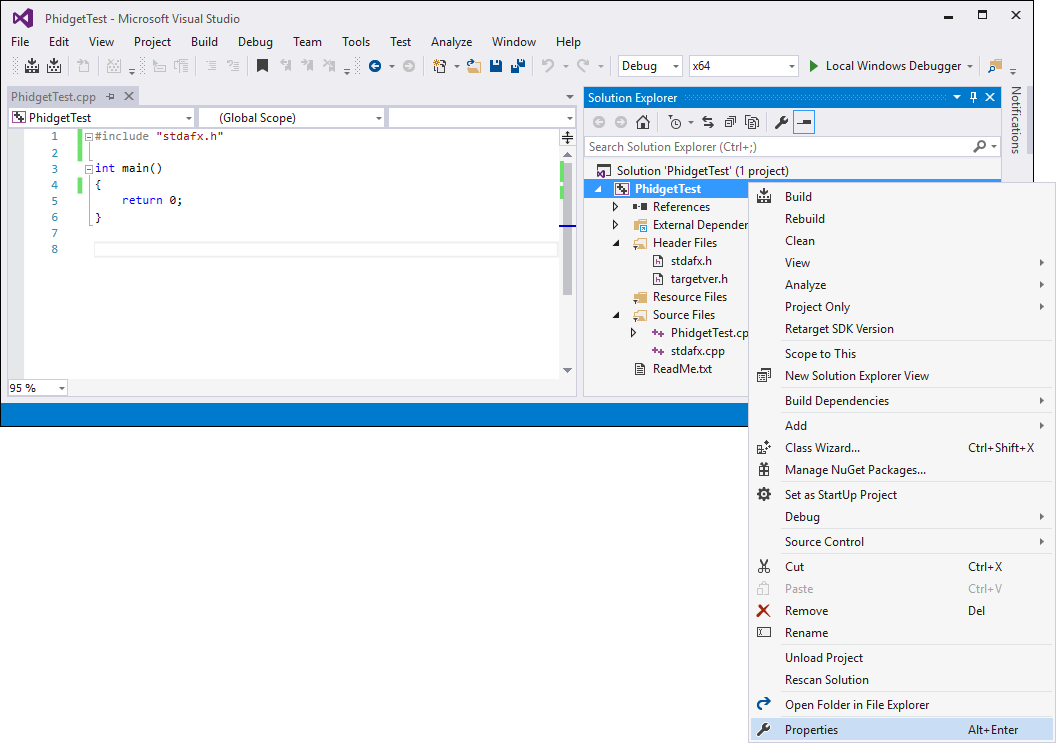
Next, navigate to Configuration Properties -> C/C++ -> General and add the following line to the additional include directories:
- C:\Program Files\Phidgets\Phidget22
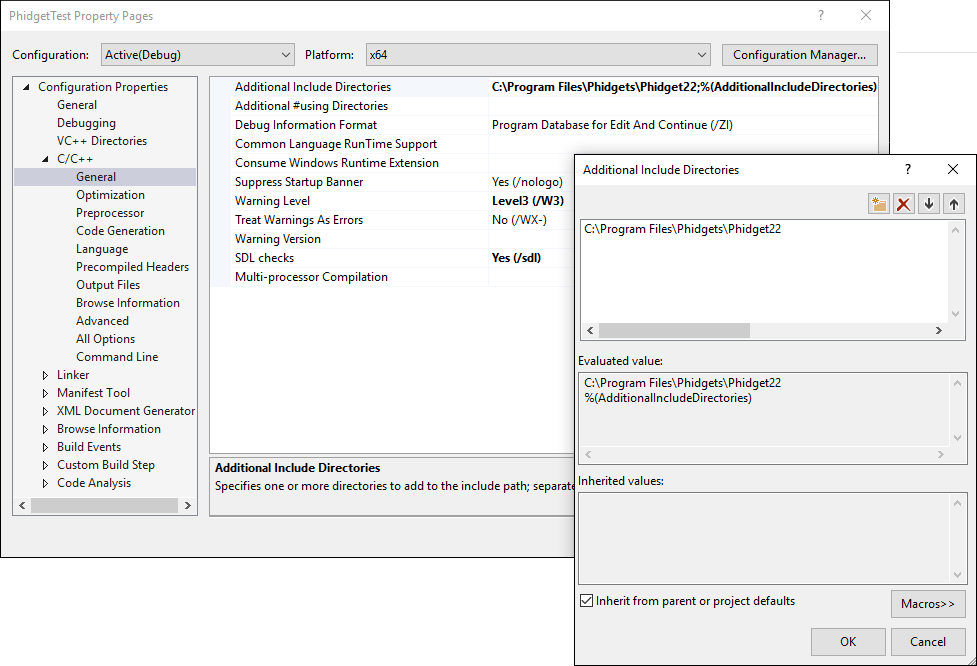
Navigate to Configuration Properties -> Linker -> Input and add the following line to the additional dependencies:
- C:\Program Files\Phidgets\Phidget22\phidget22.lib
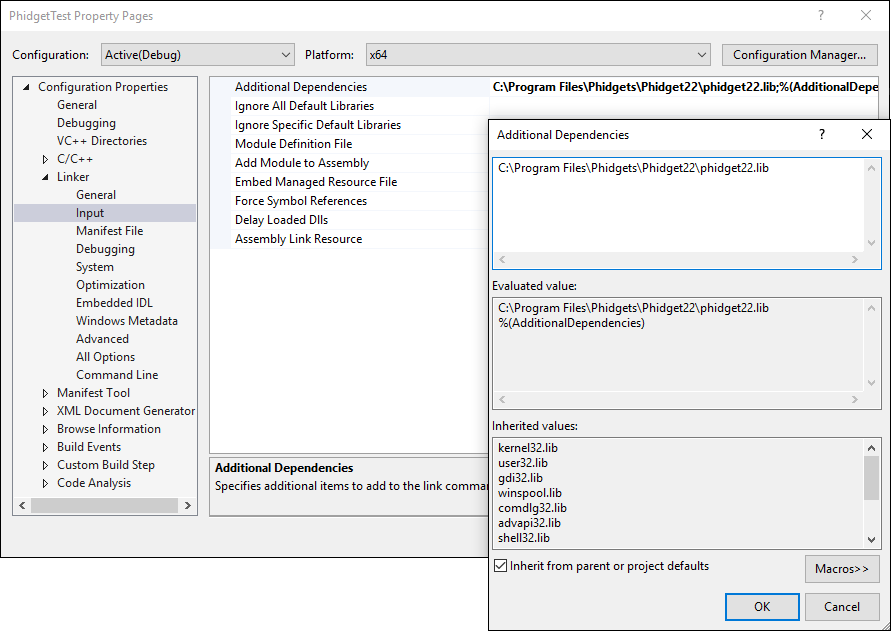
Finally, include the Phidget library in your code:
#include <phidget22.h>
Success! The project now has access to Phidgets. Next, view the write your own code section located below.
GCC
Cygwin/MinGW
Use our examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. In order to run the examples, you will need to download and install either MinGW or Cygwin.
Now that you have either MinGW or Cygwin installed, select an example that will work with your Phidget:
If you are using Cygwin, navigate to the folder where the example is and open the command prompt. Enter the following command to compile the example:
gcc example.c -o example -I"/cygdrive/c/Program Files/Phidgets/Phidget22" -L"/cygdrive/c/Program Files/Phidgets/Phidget22/x86" -lphidget22
If you are using MinGW, navigate to the folder where the example is and open the command prompt. Enter the following command to compile the example:
gcc example.c -o example -I"C:/Program Files/Phidgets/Phidget22" -L"C:/Program Files/Phidgets/Phidget22/x86" -lphidget22
After running the commands above for either Cygwin or MinGW, an executable file called example.exe will be created. Enter the following command to run the example:
example.exe
You should now have the example up and running. When you are ready, the next step is configuring your project and writing your own code!
Configure your project
When you are building a project from scratch, or adding Phidget functionality to an exisiting project, you'll need to configure your development environment to properly link the Phidget C/C++ library.
To include the Phidget C/C++ library, add the following line to your code:
#include <phidget22.h>
You can now compile the file as shown in the previous section.
The project now has access to Phidgets. Next, view the write your own code section located below.
Code::Blocks
Use our examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. In order to run the examples, you will need to download and install Code::Blocks.
Now that you have Code::Blocks installed, select an example that will work with your Phidget:
Open the example in Code::Blocks (you do not need to create a new project) and navigate to Settings -> Compiler... as shown in the image below:
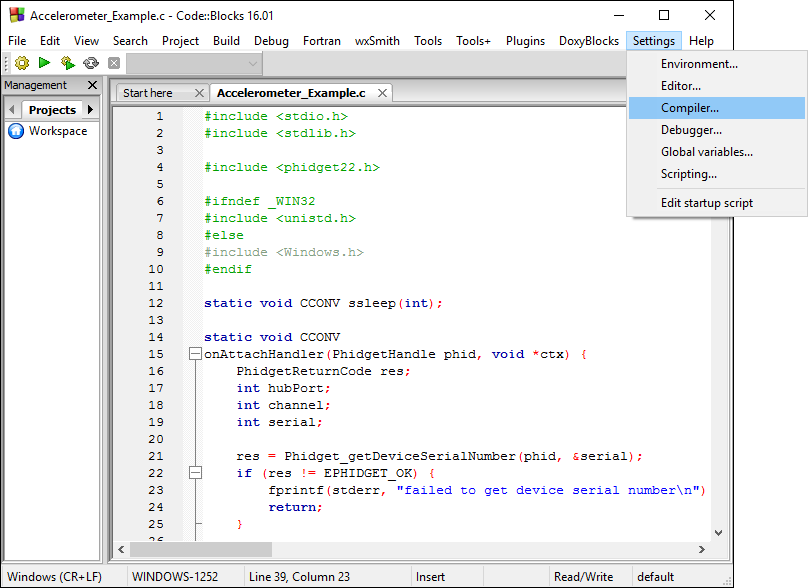
From the Global compiler settings screen, navigate to Search directories -> Compiler and add the following directory:
- C:\Program Files\Phidgets\Phidget22
Next, select Search directories -> Linker and add the following directory:
- C:\Program Files\Phidgets\Phidget22\x86
Finally, from the Global compiler settings screen, navigate to Linker settings and add the following line:
- phidget22
You can now build and run the example:
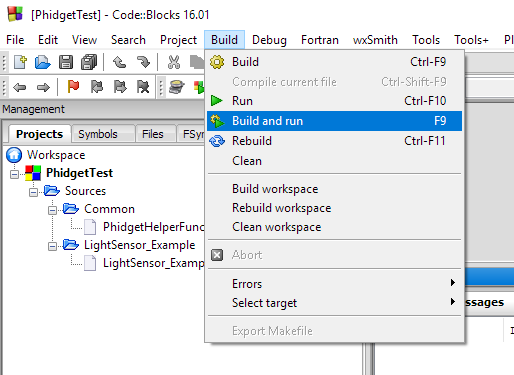
You should now have the example up and running for your device. Play around with the device and experiment with some of the functionality. When you are ready, the next step is configuring your project and writing your own code!
Configure your project
When you are building a project from scratch, or adding Phidget functionality to an existing project, you'll need to configure your development environment to properly link the Phidget C/C++ library.
To include the Phidget C/C++ library, add the following line to your code:
#include <phidget22.h>
You can now compile the file as shown in the previous section.
The project now has access to Phidgets. Next, view the write your own code section located below.
macOS
If you haven't already, please visit the macOS page before you continue reading. There you will be instructed on how to properly set up your macOS machine so you can follow the guides below!
GCC
Use our examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. You likely have gcc installed on your macOS machine already, but if not, you can easily get it by downloading Xcode.
Next, select an example that will work with your Phidget:
To compile the example program, enter the following command in the terminal:
gcc example.c -o example -F /Library/Frameworks -framework Phidget22 -I /Library/Frameworks/Phidget22.framework/Headers
Finally, run the program by entering the following command in the terminal:
./example
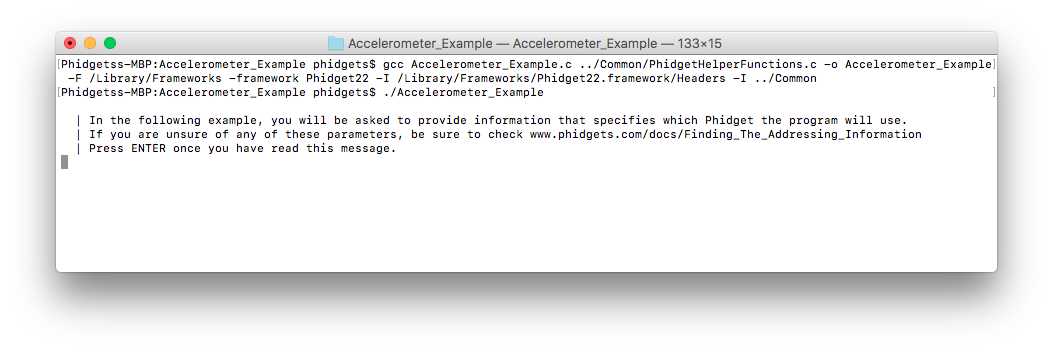
You should now have the example up and running for your device. Play around with the device and experiment with some of the functionality. When you are ready, the next step is configuring your project and writing your own code!
Configure your project
When you are building a project from scratch, or adding Phidget functionality to an exisiting project, you'll need to configure your development environment to properly link the Phidget C/C++ library.
To include the Phidget C/C++ library, simply add the following line to your code:
#include <phidget22.h>
You can now compile the file as shown in the previous section.
The project now has access to Phidgets. Next, view the write your own code section located below.
Linux
If you haven't already, please visit the Linux page before you continue reading. There you will be instructed on how to properly set up your Linux machine so you can follow the guides below!
GCC
Use our examples
One of the best ways to start programming with Phidgets is to use our example code as a guide. You likely have gcc installed on your Linux machine already, but if not, you can easily get it by entering the following command in the terminal:
apt-get install gcc
Next, select an example that will work with your Phidget:
To compile the example, enter the following command in the terminal:
gcc example.c -o example -lphidget22
After compiling, you can run the program by entering the following command in the terminal:
./example
You should now have the example up and running. When you are ready, the next step is configuring your project and writing your own code!
Configure your project
When you are building a project from scratch, or adding Phidget functionality to an exisiting project, you'll need to configure your development environment to properly link the Phidget C/C++ library.
To include the Phidget C/C++ library, simply add the following line to your code:
#include <phidget22.h>
You can now compile the file as shown in the previous section.
The project now has access to Phidgets. Next, view the write your own code section located below.
Write Code
By following the instructions for your operating system and compiler above, you now have working examples and a project that is configured. This teaching section will help you understand how the examples were written so you can start writing your own code.
Remember: your main reference for writing {{{1}}} code will be the Phidget22 API Manual and the example code.
Step One: Initialize and open
You will need to declare your Phidget object in your code. For example, we can declare a digital input object like this:
PhidgetDigitalInputHandle ch;
Next, the Phidget object needs to be initialized and opened:
PhidgetDigitalInput_create(&ch);
Phidget_open((PhidgetHandle)ch);
Although we are not including it on this page, you should handle the return values of all Phidget functions. Here is an example of the previous code with error handling:
PhidgetReturnCode res;
const char* errorString;
res = PhidgetDigitalInput_create(&ch);
if(res != EPHIDGET_OK){
Phidget_getErrorDescription ( returnValue, &errorString );
printf("\n%s", errorString );
}
res = Phidget_open((PhidgetHandle)ch);
if(res != EPHIDGET_OK){
Phidget_getErrorDescription ( returnValue, &errorString );
printf("\n%s", errorString );
}
Step Two: Wait for attachment of the Phidget
Simply calling open does not guarantee you can use the Phidget immediately. To use a Phidget, it must be plugged in (attached). We can handle this by using event driven programming and tracking the attach events. Alternatively, we can modify our code so we wait for an attachment:
PhidgetDigitalInput_create(&ch);
Phidget_openWaitForAttachment((PhidgetHandle)ch, 5000);
Waiting for attachment will block indefinitely until a connection is made, or until the timeout value is exceeded.
To use events to handle attachments, we have to modify our code slightly:
PhidgetDigitalInput_create(&ch);
Phidget_setOnAttachHandler((PhidgetHandle)ch, OnAttachedHandler, NULL)
Phidget_open((PhidgetHandle)ch);
Next, we have to declare the function that will be called when an attach event is fired - in this case the function OnAttachedHandler will be called:
void CCONV OnAttachedHandler(PhidgetHandle ch, void *userPtr){
printf("Phidget attached!");
}
Step Three: Do things with the Phidget
We recommend the use of event driven programming when working with Phidgets. In a similar way to handling an attach event as described above, we can also add an event handler for a state change event:
PhidgetDigitalInput_create(&ch);
Phidget_setOnAttachHandler((PhidgetHandle)ch,OnAttachedHandler, NULL);
PhidgetDigitalInput_setOnStateChangeHandler(ch, onStateChangeHandler, NULL);
Phidget_open((PhidgetHandle)ch);
This code will connect a function and an event. In this case, the onStateChangeHandler function will be called when there has been a change to the devices input. Next, we need to create the onStateChangeHandler function:
void CCONV onStateChangeHandler(PhidgetDigitalInputHandle ch, void *userPtr, int state){
printf("State: %d", state);
}
If events do not suit your needs, you can also poll the device directly for data using code like this:
int state;
PhidgetDigitalInput_getState(ch, &state);
printf("State: %d", state);
Step Four: Close and delete
At the end of your program, be sure to close and delete your device:
Phidget_close((PhidgetHandle)ch);
PhidgetDigitalInput_delete(&ch);
Further Reading
Phidget Programming Basics - Here you can find the basic concepts to help you get started with making your own programs that use Phidgets.
Data Interval/Change Trigger - Learn about these two properties that control how much data comes in from your sensors.
Using Multiple Phidgets - It can be difficult to figure out how to use more than one Phidget in your program. This page will guide you through the steps.
Polling vs. Events - Your program can gather data in either a polling-driven or event-driven manner. Learn the difference to determine which is best for your application.
Logging, Exceptions, and Errors - Learn about all the tools you can use to debug your program.
Phidget Network Server - Phidgets can be controlled and communicated with over your network- either wirelessly or over ethernet.